Application of PHP dichotomy in IP address query_PHP tutorial
The database probably stores hundreds of thousands of IP records, and the record set is as follows:
+----------+----------+---- --------+---------+---------+--------+--------+
| ip_begin | ip_end | country_id | prov_id | city_id | isp_id | netbar |
+-----------+-----------+---------- ---+---------+---------+--------+--------+
| 0 | 16777215 | 2 | 0 | 0 | 0 |
| 16777216 | 33554431 | 2 | 0 | 0 |
| 33554432 | 50331647 | 2 | 0 | 0 | 0 | 0 |
| 50331648 | 67108863 | 3 | 0 | 0 | 0 |
| 67108864 | 67829759 | 3 | 0 | 0 0 | 0 |
+----------+--- -------+------------+---------+---------+--------+ --------+
To do this query, you need to use the following SQL:
$sql = 'SELECT * FROM i_m_ip WHERE ip_begin <= $client_ip AND ip_end > = $client_ip';
?>
Such a retrieval obviously does not use an index. Even if it is used, the MySQL query efficiency is unlikely to reach more than 500 times per second. I have done a lot of concurrency optimization, and finally the average The query efficiency is only about 200 times per second, which is really a headache. At the beginning, I also thought of using the retrieval method of the Innocence IP library, but I have always been resistant to the algorithm and thought that the dichotomy method was difficult, so I did not try to use it. It was not until I had no choice in the end that I finally realized the dichotomy IP address retrieval method. .
From the above table, you can see that the IP library is a continuous value from 0 to 4294967295. If this value is separated and stored, there will be hundreds of gigabytes of data, so there is no way to use indexes and no way to hash. In the end, I used PHP to convert these things into binary storage, abandoning database retrieval. You can see that the starting and ending length of the IP is a 4-byte long integer. The following country ID, province ID, etc. can be stored using a 2-byte short integer. A total of one row of data has 18 bytes, a total of 31 Ten thousand pieces of data only add up to 5M.The specific IP library generation code is as follows:
/*
IP file format:
3741319168 3758096383 182 0 0 0 0
3758096384 377487359 9 3 0 0 0 0
3774873600 4026531839 182 0 0 0
4026531840 4278190079 182 0 0 0
4294967040 42949672 95 312 0 0 0 0
*/
set_time_limit(0);
$handle = fopen('./ ip.txt', 'rb');
$fp = fopen("./ip.dat", 'ab');
if ($handle) {
while (!feof($handle )) {
$buffer = fgets($handle);
$buffer = trim($buffer);
$buffer = explode("t", $buffer);
foreach ($buffer as $key => $value) {
$buffer[$key] = (float) trim($value);
$str = pack('L', $buffer[0] );
$str .= pack('L', $buffer[1]);
$str .= pack('S', $buffer[2]);
$str .= pack ('S', $buffer[3]);
$str .= pack('S', $buffer[4]);
$str .= pack('S', $buffer[5] );
$str .= pack('S', $buffer[6]);
fwrite($fp, $str);
}
}
?>
In this way, the IPs are arranged in units of 18 bytes in order, so it is easy to use the binary method to retrieve the IP information:
function getip($ip, $fp) {
fseek($ fp, 0);
$begin = 0;
$end = filesize('./ip.dat');
$begin_ip = implode('', unpack('L', fread($ fp, 4)));
fseek($fp, $end - 14);
$end_ip = implode('', unpack('L', fread($fp, 4)));
$begin_ip = sprintf('%u', $begin_ip);
$end_ip = sprintf('%u', $end_ip);
do {
if ($end - $begin <= 18) {
fread($fp, 2)));
$info[1] = implode('', unpack('S', fread($fp, 2)));
$info[2] = implode ('', unpack('S', fread($fp, 2)));
$info[3] = implode('', unpack('S', fread($fp, 2)));
$info[4] implode('', unpack('S', fread($fp, 2)));
$middle_seek = ceil((($end - $begin) / 18) / 2) * 18 + $begin;
fseek($fp, $middle_seek);
$ middle_ip = implode('', unpack('L', fread($fp, 4)));
$middle_ip = sprintf('%u', $middle_ip);
if ($ip >= $middle_ip) {
(true);
}
The above $fp is the file handle to open ip.dat. Since it is a loop retrieval, it is written outside the function to avoid opening the file once for each retrieval. The 30W row data dichotomy only needs to be looped 7 times at most ( You can find accurate IP information in about 2^7). Later, I originally wanted to put ip.dat in the memory to speed up the retrieval. Later I found that the efficiency of the string positioning function was not at the same order of magnitude as the offset positioning of the file pointer, so I gave up using memory to store the IP library.
This implementation has improved the IP retrieval efficiency by nearly a hundred times. It is just a simple application of the dichotomy. From then on, the concept that the algorithm is not important in WEB applications has been completely dispelled. In fact, to achieve this, I also asked Jinhu for advice. I initially asked him to help me generate an IP library in a pure format, and then use Discuz’s IP query function to search for it. However, he refused to help me, and finally created my This practice and learning. Sometimes, it is better to ask for others than to ask for yourself.
http://www.bkjia.com/PHPjc/319312.html
www.bkjia.com

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


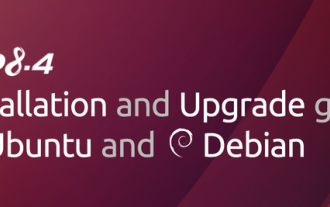
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
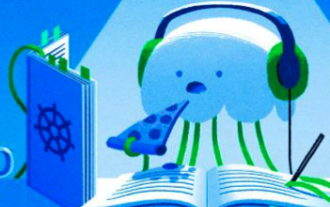
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
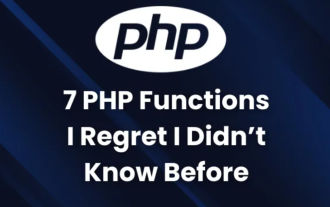
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
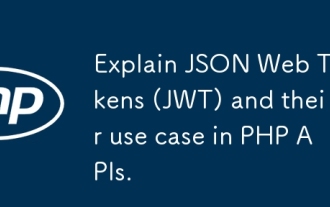
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
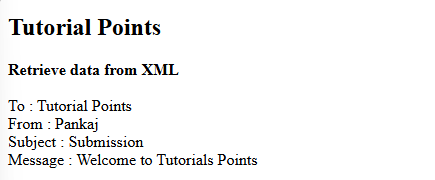
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
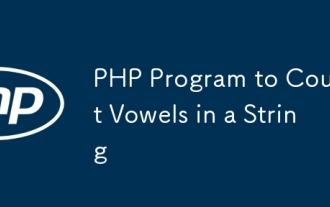
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
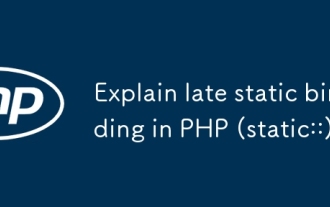
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
