


MySQL database user authentication system code written in PHP_PHP tutorial
In the past two days, I was asked by a friend to help him write a user authentication system using the MySQL database. Of course I couldn't shirk it, so I had to spend a whole night's rest and write a very simple PHP program.
The principle of user authentication is very simple: first, the user needs to fill in the user name and password on the page. Of course, unregistered users need to register first. Then call the database to search whether there is a corresponding user. If yes, confirm it; if not, remind the user to register first. It's easy to do all this using PHP, but it should be noted that if I want to confirm the user's identity on subsequent pages, using PHP3 I can only figure out how to use cookies. If you want to use session, you can only wait for the official version of PHP4 to be released!
The first step is to create a login page, so I won’t go into details here. I just made a very simple one, you can make it more beautiful.
The second step begins with the design of the confirmation program after login.
login.php:
mysql_connect("localhost","user", "password")
/*Connect to the database, change the username and password yourself*/
or die("Unable to connect to the database, please try again");
mysql_select_db("userinfo")
or die ("Unable to select database, please try again");
$today=date("Y-m-d H:i:s");
$query="
select id
from usertbl
where name=$name and password=$password
/*Search and log in the user’s corresponding information from the database*/
";
$result=mysql_query($query);
$numrows= mysql_num_rows($result);
if($numrows==0){
/*Verify whether the user with the same information can be found, if not, it will not be registered*/
echo Illegal user
echo Please register first
echo Retry
}
else{
$row=mysql_fetch_array($result);
$id=$row[0];
$query="
update usertbl
set lastlogin=$today
where id=$id";
$result=mysql_query($query);
SetCookie("usercookie" , "Welcome, $name");
/*Cookies are used here to facilitate subsequent page authentication.
But I haven’t finished developing this area yet. I hope anyone who is interested can correct me*/
echo Login successful
echo Please come in!
}
?>
The third step is of course to prepare the registration page, which I won’t go into details.
The fourth step is to confirm your identity after registration and enter it into the database.
register.php:
mysql_connect("localhost","user"," password") /*Please change the username and password*/
or die("Unable to connect to the database, please try again");
mysql_select_db("userinfo")
or die("Unable to select the database, please try again"); Try again");
$query="select id from usertbl where name=$name";
/*Search for user information with the same name from the database*/
$result=mysql_query($query) ;
$numrows=mysql_num_rows($result);
if($numrows!=0) /*If you find it, of course someone has registered the same name first*/
{echo Someone has already registered this name. Please reselect your name!;}
else
{$query="insert into usertbl values(0,$name,$password,)";
/*If you can’t find the same one, enter a new user Information*/
mysql_query($query);
echo Registration successful;
echo Please log in!;}
?>
if(!$usercookie )
{header("Illegal user");
}
?>
welcome.php:
require("cookie.php"); /*Calling cookie.php*/
?>
echo $usercookie;
?>
create table usertbl
(
ID int auto_increment primary key,
Name varchar(30),
Password varchar(20),
Lastlogin varchar(20)
);

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


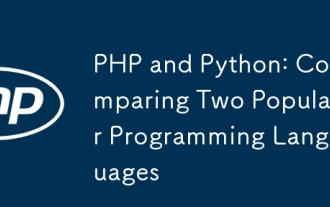
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
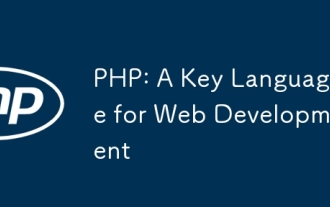
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
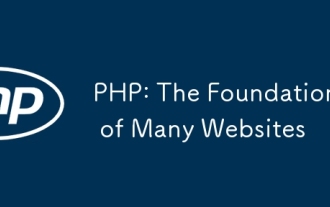
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
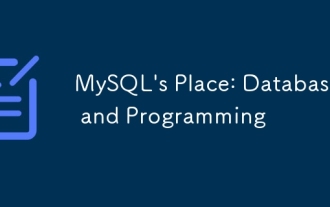
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
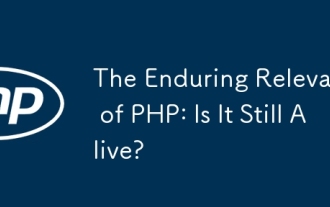
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
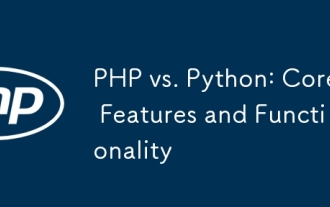
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
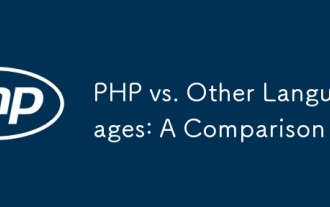
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
