Section 8--Access Method_PHP Tutorial
/*
+-------------------------------------------------- ----------------------------------+
| = This article is read by Haohappy<
| = Notes from the Chapter Classes and Objects
| = Translation + personal experience
| = To avoid possible unnecessary trouble, please do not reprint, thank you
| = We welcome criticisms and corrections, and hope to make progress together with all PHP enthusiasts!
| = PHP5 Research Center: http://blog.csdn.net/haohappy2004
+---------- -------------------------------------------------- ------------------+
*/
Section 8--Access Method
PHP5's access method allows restricting access to class members. This is a new feature in PHP5, but it has long existed in many object-oriented languages. With access, you can develop a reliable object-oriented application and build a reusable object-oriented class library.
Like C++ is the same as Java. PHP has three access methods: public, private and protected. The access method of a class member can be one of them. If you do not specify the access method, the default access method is public. You can also use static Members specify an access method, and the access method is placed before the static keyword (such as public static).
Public members can be accessed without restrictions. Any code outside the class can read and write public properties. You can read and write public properties from Call a public method anywhere in the script. In previous versions of PHP, all methods and properties were public, which made objects feel like well-structured arrays.
Private members were only in classes Visible internally. You cannot change or read the value of a private property outside the class method in which it resides. Likewise, only methods in the same class can call a private method. Inherited subclasses cannot access the parent class. private members in.
It should be noted that any member in the class and instances of the class can access private members. See Example 6.8, the equals method compares two widgets. The == operator compares two widgets of the same class. Object, but in this example each object instance has a unique ID. The equals method only compares name and price. Note how the equals method accesses the private property of another Widget instance. Both Java and C allow this operation.
Listing 6.8 Private members
{
private $name;
private $price;
private $id;
public function __construct($name, $price)
{
$this->name = $name;
$this->price = floatval($price);
$this->id = uniqid();
}
//checks if two widgets are the same 检查两个widget是否相同
public function equals($widget)
{
return(($this->name == $widget->name)AND
($this->price == $widget->price));
}
}
$w1 = new Widget('Cog', 5.00);
$w2 = new Widget('Cog', 5.00);
$w3 = new Widget('Gear', 7.00);
//TRUE
if($w1->equals($w2))
{
print("w1 and w2 are the same
n");
}
//FALSE
if($w1->equals($w3))
{
print("w1 and w3 are the same
n");
}
//FALSE, == includes id in comparison
if($w1 == $w2) //不等,因为ID不同
{
print("w1 and w2 are the same
n");
}
?>
Of course, most private properties can still be shared by external code. The solution is to use a pair of public methods, one is get (get the value of the property), the other is set (set the value of the property). The constructor also accepts The initial value of the property. This allows communication between members to occur through a narrow, well-qualified interface. This also provides the opportunity to change the value passed to the method. Note in Example 6.8 how the constructor forces price to be a float number (floadval()).
Protected (protected) members can be accessed by all methods in the same class and all methods in inherited classes. Public properties violate the spirit of encapsulation because they allow Subclasses rely on a specific attribute to be written. Protected methods do not cause this concern. A subclass that uses a protected method needs to be very clear about the structure of its parent class.
Example 6.9 is derived from Example 6.8 Improved to include a Widget subclass, Thing. Note that Widget now has a protected method called getName. If an instance of Widget attempts to call the protected method an error will occur: $w1->getName() generated an error. But the getName method in the subclass Thing can call this protected method. Of course, this example is too simple to prove that the Widget::getName method is protected. In actual situations, using the protected method depends on understanding the internal structure of the object.
Listing 6.9 Protected members
{
private $name;
private $price;
private $id;
public function __construct($name, $price)
{
$this->name = $name;
$this->price = floatval($price);
$this->id = uniqid();
}
//checks if two widgets are the same
public function equals($widget)
{
return(($this->name == $widget->name)AND
($this->price == $widget->price));
}
protected function getName()
{
return($this->name);
}
}
class Thing extends Widget
{
private $color;
public function setColor($color)
{
$this->color = $color;
}
public function getColor()
{
return($this->color);
}
public function getName()
{
return(parent::getName());
}
}
$w1 = new Widget('Cog', 5.00);
$w2 = new Thing('Cog', 5.00);
$w2->setColor('Yellow');
//TRUE (still!) 结果仍然为真
if($w1->equals($w2))
{
print("w1 and w2 are the same
n");
}
//print Cog 输出 Cog
print($w2->getName());
?>
A subclass may change the way the method is accessed by overriding the parent class method. However, there are still some restrictions. If you override a public class member, it must remain public in the subclass. If you override You write a protected member, which can remain protected or become public. Private members are still visible only in the current class. Declaring a member with the same name as a private member of the parent class will simply create a different member in the current class. . Therefore, technically you cannot override a private member.
The Final keyword is another way to restrict access to member methods. Subclasses cannot override methods marked final in the parent class. The Final keyword cannot be used Properties.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


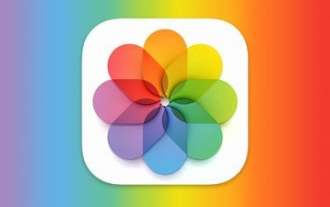
In iOS17, Apple has more control over what apps can see in photos. Read on to learn how to manage app access by app. In iOS, Apple's in-app photo picker lets you share specific photos with the app, while the rest of your photo library remains private. Apps must request access to your entire photo library, and you can choose to grant the following access to apps: Restricted Access – Apps can only see images that you can select, which you can do at any time in the app or by going to Settings > ;Privacy & Security>Photos to view selected images. Full access – App can view photos
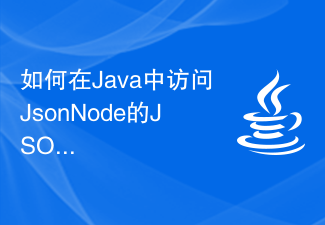
A JsonNode is Jackson's JSON tree model that can read JSON into JsonNode instances and write JsonNode into JSON. We can use Jackson to read JSON into a JsonNode by creating an ObjectMapper instance and calling the readValue() method. We can access fields, arrays or nested objects using the get() method of the JsonNode class. We can use the asText() method to return a valid string representation and convert the node's value to Javaint using the asInt() method of the JsonNode class. In the example below we can access Json
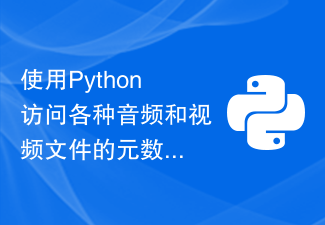
We can access the metadata of audio files using Mutagen and the eyeD3 module in Python. For video metadata we can use movies and the OpenCV library in Python. Metadata is data that provides information about other data, such as audio and video data. Metadata for audio and video files includes file format, file resolution, file size, duration, bitrate, etc. By accessing this metadata, we can manage media more efficiently and analyze the metadata to obtain some useful information. In this article, we will take a look at some of the libraries or modules provided by Python for accessing metadata of audio and video files. Access audio metadata Some libraries for accessing audio file metadata are - using mutagenesis
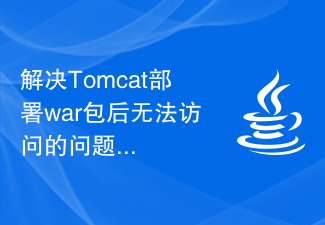
How to solve the problem that Tomcat cannot successfully access the war package after deploying it requires specific code examples. As a widely used Java Web server, Tomcat allows developers to package their own developed Web applications into war files for deployment. However, sometimes we may encounter the problem of being unable to successfully access the war package after deploying it. This may be caused by incorrect configuration or other reasons. In this article, we'll provide some concrete code examples that address this dilemma. 1. Check Tomcat service
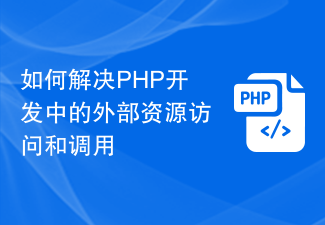
How to solve the problem of accessing and calling external resources in PHP development requires specific code examples. In PHP development, we often encounter situations where we need to access and call external resources, such as API interfaces, third-party libraries or other server resources. When dealing with these external resources, we need to consider how to access and call safely while ensuring performance and reliability. This article describes several common solutions and provides corresponding code examples. 1. Use the curl library to call external resources. Curl is a very powerful open source library.
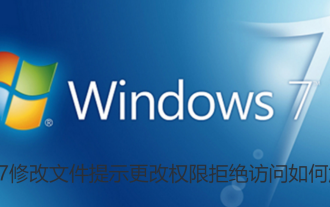
How to solve the problem of access denied when modifying files in win7? When modifying some system files, we will often be prompted that we do not have permission to perform the operation. We can turn off the folder permissions or obtain administrator rights. For users who need to modify such files, let’s take a look at the following specific tutorials. Solution to the problem of access denied when modifying files in win7: 1. First select the corresponding folder, click the tool above, and select the folder option. 2. Enter the View tab. 3. Uncheck Use Simple File Sharing and confirm. 4. Then right-click the corresponding folder and click Properties. 5. Enter the Security tab. 6. Select the icon position and click Advanced. 7
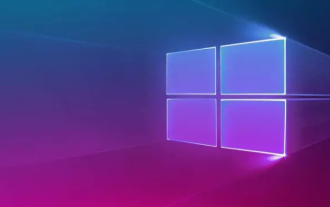
Sharing folders is indeed an extremely useful feature in a home or business network environment. It allows you to easily share folders with other users, thereby facilitating file transfer and sharing. Win10 Home Edition shared folder cannot be accessed Solution: Solution 1: Check network connection and user permissions When trying to use Win10 shared folders, we first need to confirm whether the network connection and user permissions are normal. If there is a problem with the network connection or the user does not have permission to access the shared folder, it may result in inaccessibility. 1. First, please ensure that the network connection is smooth so that the computer and the computer where the shared folder is located are in the same LAN and can communicate normally. 2. Secondly check the user permissions to confirm that the current user has permission to share files.
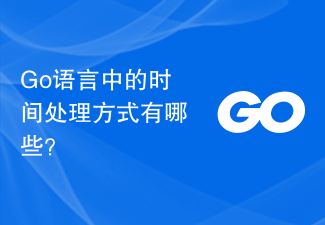
As a modern programming language, Go language plays an important role in development. The Go language provides some built-in time functions and structures to make time processing more convenient. In this article, we will introduce some commonly used time processing methods in the Go language. time.Now() We can use the time.Now() function to get the current time: now:=time.Now()fmt.Println(now) output: 2019-06-131
