The second day of learning PHP in ten days_PHP tutorial
Learning purpose: Master the process control of PHP
1. There are three structures of if..else loops
The first one is to use only the if condition and treat it as a simple judgment. Interpreted as "what to do if something happens". The syntax is as follows:
if (expr) { statement }
where expr is the condition for judgment, usually using logical operation symbols as the condition for judgment. The statement is the execution part of the program that meets the conditions. If the program has only one line, the curly brackets {} can be omitted.
Example: This example omits the curly brackets.
if ($state==1)echo "Haha" ;
?>
What’s important to note here is that judging whether they are equal is == Instead of =, ASP programmers may often make this mistake, = is assignment.
Example: The execution part of this example has three lines, and the curly brackets cannot be omitted.
if ($state==1) {
echo "Haha;
echo "
" ;
}
?>
The second type is to add an else condition in addition to if, which can be interpreted as "what to do if something happens, otherwise how to solve it" The syntax is as follows
if ($state==1) {
echo "haha" ;
echo "
";
}
else{
echo "haha";
echo "
";
}
?>
The third type is the recursive if..else loop, which is usually used in various decision-making situations. Combine several if..else and apply them
echo "a is bigger than b" ;
} elseif ( $a == $b ) {
echo "a is equal to b" ;
} else {
echo "a is smaller than b" ;
}
?>
The above example only uses a two-level if..else loop to compare the two variables a and b. This kind of recursive if..else loop is actually used. , please use it with caution, because too many layers of loops can easily cause problems in the design logic, or missing braces, etc., will cause inexplicable problems in the program 2. There is only one kind of for loop. , no change, its syntax is as follows for (expr1; expr2; expr3) { statement } where expr1 is the initial value of the condition and expr2 is the condition for judgment, which is usually used. Logical operators are the conditions for judgment. expr3 is the part to be executed after executing the statement. It is used to change the conditions for the next loop judgment, such as adding one, etc. The statement is the execution part of the program that meets the conditions. If the program has only one line, the curly brackets {} can be omitted. The following example is written using a for loop. for ( $i = 1 ; $i <= 10 ; $i ++) {
echo "This is the ".$i."th loop< ;br>" ;
}
?>
3. The switch loop usually handles compound conditional judgments. Each sub-condition is part of the case instruction. In practice, if many similar if instructions are used, it can be synthesized into a switch loop. The syntax is as follows switch (expr) { case expr1: statement1; break; case expr2: statement2; break; default: statementN; break; } The expr condition , usually a variable name. The exprN after case usually represents the variable value. After the colon is the part to be executed that meets the condition. Be sure to use break to break out of the loop. switch ( date ( "D" )) {
case "Mon" :
echo "Today is Monday" ;
break;
case "Tue" :
echo "Today is Tuesday" ;
break;
case "Wed" :
echo "Today is Wednesday" ;
break;
case "Thu" :
echo "Today is Thursday" ;
break;
case "Fri" :
echo "Today is Friday" ;
break;
default:
echo "Today is a holiday" ;
break;
}
?>
What needs to be noted here is break; don’t omit it, default, and omission is okay. Obviously, using the if loop in the above example is very troublesome. Of course, when designing, you should put the conditions with the greatest probability of occurrence at the front and the conditions with the least occurrence at the end, which can increase the execution efficiency of the program. In the above example, since the probability of occurrence is the same every day, there is no need to pay attention to the order of the conditions. That’s it for today. Let’s start talking about the use of database tomorrow.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
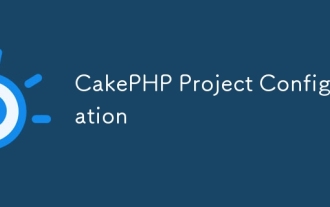
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
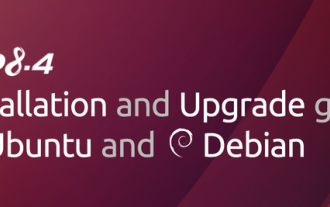
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
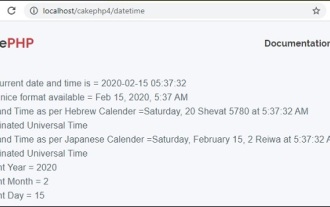
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
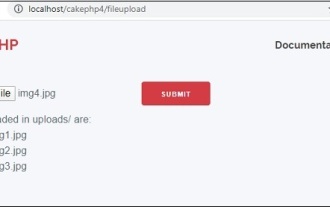
To work on file upload we are going to use the form helper. Here, is an example for file upload.
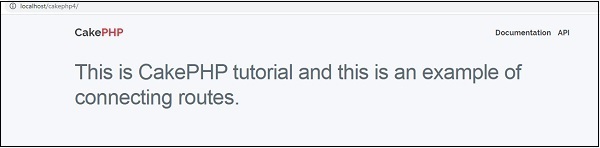
In this chapter, we are going to learn the following topics related to routing ?
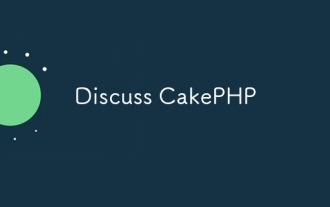
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
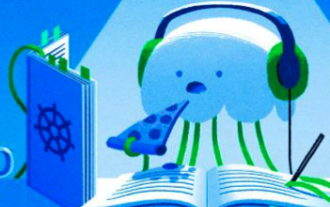
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
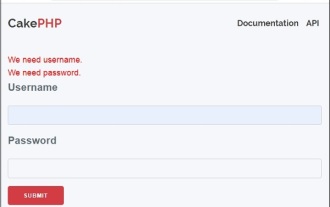
Validator can be created by adding the following two lines in the controller.
