Passing by reference of JavaScript object parameters_javascript tips
I encountered a problem today, how to affect parameter changes outside the function, such as:
<script> var myname = "wood"; A(myname); document.write(myname); function A(n) { n = "Yao"; } </script>
The output result is still wood, which means that when myname is passed into function A, there is equivalent to a copy of myname in the function body. The value of this copy is equal to myname. The subsequent operations performed on it in the function body are based on this copy. carried out.
But the situation is different. When the parameters passed in are arrays and objects, changes made to the parameters in the function body will be reflected in the original variables.
<script> var myname = ["wood"]; A(myname); document.write(myname[0]); function A(n) { n[0] = "Yao"; } </script>
As you can see, the first element of the friut array has been changed in the above code.
The following is an example of an object:
<script> var myname = {name1:"wood"}; A(myname); document.write(myname.name1); function A(n) { n.name1 = "Yao"; } </script>
It can be clearly seen that the changes to the parameters in the function body affect the original variables, which is qualitatively different from the usual parameter passing. Special attention is required.
But, when assigning a value to the passed array or object inside the function body, this change will not be reflected in the original variable outside the function body!
Please see:
<script> var myname = {name1:"wood"}; A(myname); document.write(myname.name1); function A(n) { n = {name1:"Yao"}; } </script>
According to the theory that changes within the function above will be reflected in the original variable, you must think that the value of the name1 attribute of the myname variable has changed to 'Yao' after executing A(). But the results were a bit hard to swallow.
The reason is that when the assignment operation is used in the function body, the system creates a variable named p. This p is a variable inside the function. Of course, assigning a value to it only works within the function body. The myname outside is still the original myname.
The only difference between this step and the original code is whether to assign new values to the parameters within the function body or to change the attributes of the parameters or the elements of the array.
Below we use the method of passing objects to re-implement an example of clock digital formatting output:
<script> var mytime = self.setInterval(function() { getTime(); }, 1000); //alert("ok"); function getTime() { var timer = new Date(); var t = { h: timer.getHours(), m: timer.getMinutes(), s: timer.getSeconds() } //将时间对象t,传入函数checkTime(),直接在checkTime()中改变对象中的值。 //而无需再去接收返回值再赋值 checkTime(t); document.getElementById("timer").innerHTML = t.h + ":" + t.m + ":" + t.s; } function checkTime(i) { if (i.h < 10) { i.h = "0" + i.h; } if (i.m < 10) { i.m = "0" + i.m; } if (i.s < 10) { i.s = "0" + i.s; } } </script>
The example uses the setInterval() function to call the refresh event regularly, or it can also be implemented by using setTimeout() to call recursively in getTime().
The above is the entire content of this article. I hope it will be helpful to everyone in learning javascript programming.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
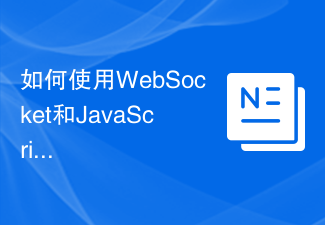
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
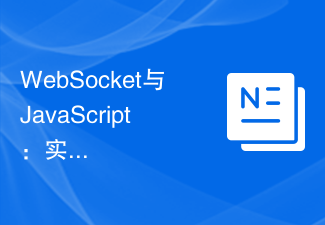
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
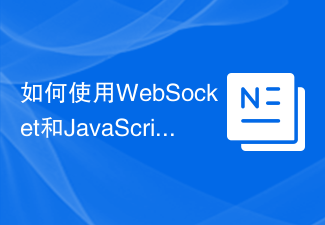
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
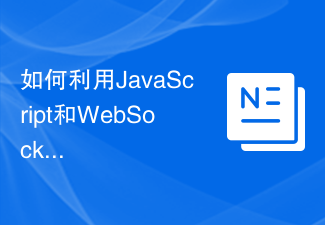
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
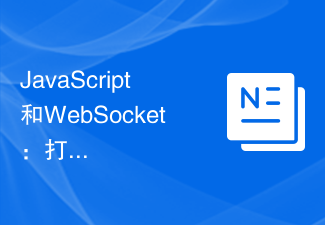
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
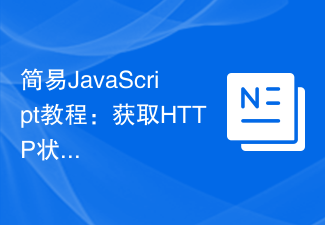
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
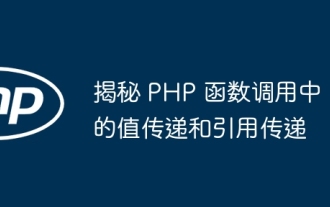
Function calls in PHP can be passed by value or by reference. The default is to pass by value, the function receives a copy of the parameter, and modifications to it do not affect the original value. Pass by reference is declared by adding an & symbol before the parameter, and the function directly modifies the passed variable. Passing by reference is useful when you need a function to modify an external variable, such as an array element.
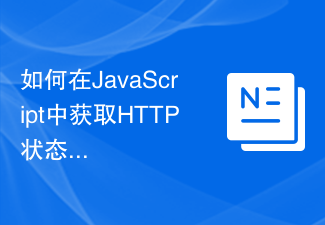
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
