php实现的替换敏感字符串类实例_php技巧
本文实例讲述了php实现的替换敏感字符串类及其用法,在php程序开发中有着非常广泛的应用价值。分享给大家供大家参考。具体方法如下:
StrFilter.class.php类文件如下:
<?php /** string filter class * Date: 2013-01-09 * Author: fdipzone * Ver: v1.0 * * Func: * public replace 替换非法字符 * public check 检查是否含有非法字符 * private protect_white_list 保护白名单 * private resume_white_list 还原白名单 * private getval 白名单 key转为value */ class StrFilter{ // class start private $_white_list = array(); private $_black_list = array(); private $_replacement = '*'; private $_LTAG = '[[##'; private $_RTAG = '##]]'; /** * @param Array $white_list * @param Array $black_list * @param String $replacement */ public function __construct($white_list=array(), $black_list=array(), $replacement='*'){ $this->_white_list = $white_list; $this->_black_list = $black_list; $this->_replacement = $replacement; } /** 替换非法字符 * @param String $content 要替換的字符串 * @return String 替換后的字符串 */ public function replace($content){ if(!isset($content) || $content==''){ return ''; } // protect white list $content = $this->protect_white_list($content); // replace black list if($this->_black_list){ foreach($this->_black_list as $val){ $content = str_replace($val, $this->_replacement, $content); } } // resume white list $content = $this->resume_white_list($content); return $content; } /** 检查是否含有非法自符 * @param String $content 字符串 * @return boolean */ public function check($content){ if(!isset($content) || $content==''){ return true; } // protect white list $content = $this->protect_white_list($content); // check if($this->_black_list){ foreach($this->_black_list as $val){ if(strstr($content, $val)!=''){ return false; } } } return true; } /** 保护白名单 * @param String $content 字符串 * @return String */ private function protect_white_list($content){ if($this->_white_list){ foreach($this->_white_list as $key=>$val){ $content = str_replace($val, $this->_LTAG.$key.$this->_RTAG, $content); } } return $content; } /** 还原白名单 * @param String $content * @return String */ private function resume_white_list($content){ if($this->_white_list){ $content = preg_replace_callback("/\[\[##(.*?)##\]\].*?/si", array($this, 'getval'), $content); } return $content; } /** 白名单 key还原为value * @param Array $matches 匹配white_list的key * @return String white_list val */ private function getval($matches){ return isset($this->_white_list[$matches[1]])? $this->_white_list[$matches[1]] : ''; // key->val } } // class end ?>
demo示例如下:
<?php header("content-type:text/html;charset=utf8"); require("StrFilter.class.php"); $white = array('屌丝', '曹操'); $black = array('屌', '操'); $content = "我操,曹操你是屌丝,我屌你啊"; $obj = new StrFilter($white, $black); echo $obj->replace($content); ?>
完整实例代码点击本站下载
希望本文所述对大家php程序设计的学习有所帮助。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




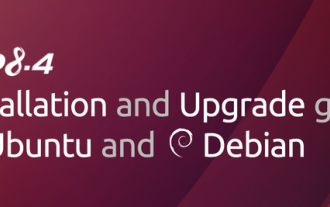
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
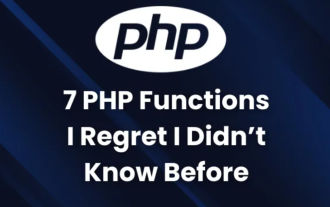
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
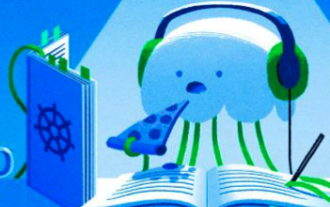
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
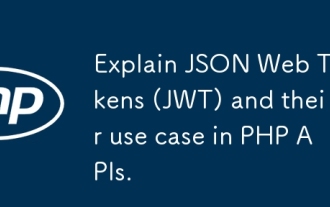
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
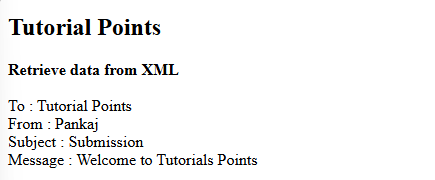
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
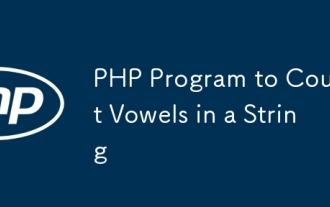
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
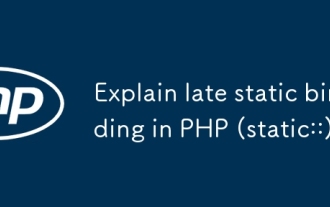
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
