


Examples to explain javascript registration event processing function_javascript skills
Events are the core content of JavaScript, and their importance will not be introduced here. After the event is triggered, an event processing function is needed to handle it. For example, we can define that when a button is clicked, the background of a div will be set to green. Then let's first take a look at how to achieve this effect. The code example is as follows:
<html> <head> <meta charset=" utf-8"> <title>javascript如何注册事件处理函数</title> <style type="text/css"> #mydiv{ width:100px; height:100px; background-color:red; } </style> <script type="text/javascript"> function changebg(){ var mydiv=document.getElementById("mydiv"); mydiv.style.backgroundColor="green"; } </script> </head> <body> <div id="mydiv"></div> <button id="bt">点击查看效果</button> </body> </html>
In the above code, clicking the button will set the background color of the div to green. This is because the code registers an event handler function for the onclick event of the button. This function can set the background color of the div to green. Let’s briefly introduce how to register event processing functions for object events with examples:
Method 1:
Register the event processing function directly in the HTML code, that is, set the event processing function directly through the HTML attribute. The code to be executed by the event processing function is the HTML attribute value. This method is used at the beginning of the article. The advantages and disadvantages are as follows:
- 1. Easy to understand and use.
- 2. All major browsers support this method.
- 3. Mixed with HTML code, making the page very complicated and inconsistent with the principle of separation of performance and content.
- 4. Only one event handler of the same type can be registered on the same object.
Method 2:
Event handle mode, the so-called event handle is the event processing function, and the specified event of the specified object corresponds to an event handler. To register an event processing function in this way, you must first obtain a reference to the object, and then assign the event handle to the corresponding event processing function attribute of the object. In fact, method one is also a kind of event handler method.
The code example is as follows:
<html> <head> <meta charset=" utf-8"> <title>javascript如何注册事件处理函数</title> <style type="text/css"> #mydiv{ width:100px; height:100px; background-color:red; } </style> <script type="text/javascript"> window.onload=function(){ var mydiv=document.getElementById("mydiv"); var bt=document.getElementById("bt"); bt.onclick=function(){ mydiv.style.backgroundColor="green"; } } </script> </head> <body> <div id="mydiv"></div> <button id="bt">点击查看效果</button> </body> </html>
In the above code, first use document.getElementById("bt") to obtain the reference of the button object, and then assign the event handle (event handler function) to the onclick event attribute of the button object, so that onclick will be triggered when the button is clicked. event, and then execute the code in the event handler. The advantages and disadvantages are as follows:
- 1. Simple and easy to understand.
- Supported by 2 browsers.
- 3. Only one event handler of the same type can be registered on the same object.
Method 3:
It is a more advanced event registration method, that is, event listener. This method solves the problem that only one handler function for a specified type of event can be registered in a specified object. However, there are certain compatibility issues, which are introduced below:
1).IE browser:
In IE browser, you can use the attachEvent() and detachEvent() methods to register event handling functions for the specified object and to delete the registered event handling functions.
The syntax format is as follows:
element.attachEvent("onevent",eventListener)
This function has two parameters, the first parameter is the name of the event type, and the second parameter is the event handler function to be registered.
The code example is as follows:
<html> <head> <meta charset=" utf-8"> <title>javascript如何注册事件处理函数</title> <style type="text/css"> #mydiv{ width:100px; height:100px; background-color:red; } </style> <script type="text/javascript"> window.onload=function(){ var mydiv=document.getElementById("mydiv"); var bt=document.getElementById("bt"); bt.attachEvent("onclick",changebg); function changebg(){ mydiv.style.backgroundColor="green"; } } </script> </head> <body> <div id="mydiv"></div> <button id="bt">点击查看效果</button> </body> </html>
The above code uses the attachEvent() function to register the onclick event handler for the button, but it is only valid in IE browser. Use the detachEvent() function to detach the originally registered event processing function. The syntax format is as follows:
element.detachEvent("onevent",eventListener)
The format is the same as the attachEvent() function.
Special note: The first parameter must contain on. For example, the click event must be written as "onclick".
2). Standard browser:
In standard browsers (including IE9 and IE9 and above browsers), use the addEventListener() and removeEventListener() functions to register and delete registration processing functions.
The syntax format is as follows:
element.addEventListener('event', eventListener, useCapture);
This function has three parameters. The first parameter is the event type name. The second parameter is the event handler function to be registered. The third function specifies whether this handler function is called during the capture phase of the event delivery process or bubbles up. The stage is called. Under default conditions, the value of this property is false, which means that the event handler is called during the bubbling stage.
Special note: The first parameter cannot contain on. For example, the click event cannot be written as "onclick", but must be written as "click".
The code example is as follows:
<html> <head> <meta charset=" utf-8"> <title>javascript如何注册事件处理函数</title> <style type="text/css"> #mydiv{ width:100px; height:100px; background-color:red; } </style> <script type="text/javascript"> window.onload=function(){ var mydiv=document.getElementById("mydiv"); var bt=document.getElementById("bt"); bt.addEventListener("click",changebg); function changebg(){ mydiv.style.backgroundColor="green"; } } </script> </head> <body> <div id="mydiv"></div> <button id="bt">点击查看效果</button> </body> </html>
以上代码在IE9和IE9以上或者其他标准浏览器中,点击按钮可以将div的背景颜色设置为绿色。使用removeEventListener()函数可以解除原来注册的事件处理函数,语法格式如下:
element.removeEventListener('event', eventListener, useCapture);
格式和addEventListener()函数式一样的。
跨浏览器注册事件处理函数:
只要加个判断语句即可,代码如下:
var EventUtil={ //注册 addHandler: function(element, type, handler){ if (element.addEventListener){ element.addEventListener(type, handler, false); } else if (element.attachEvent){ element.attachEvent("on" + type, handler); } else { element["on" + type] = handler; } }, //移除注册 removeHandler: function(element, type, handler){ if (element.removeEventListener){ element.removeEventListener(type, handler, false); } else if (element.detachEvent){ element.detachEvent("on" + type, handler); } else { element["on" + type] = null; } } };
以上就是本文的详细内容,希望对大家的学习有所帮助。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


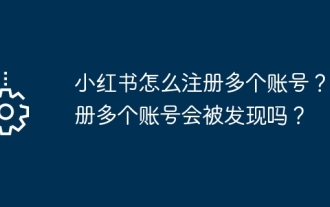
As a platform integrating social networking and e-commerce, Xiaohongshu has attracted more and more users to join. Some users hope to register multiple accounts to better experience interacting with Xiaohongshu. So, how to register multiple accounts on Xiaohongshu? 1. How to register multiple accounts on Xiaohongshu? 1. Use different mobile phone numbers to register. Currently, Xiaohongshu mainly uses mobile phone numbers to register accounts. Users sometimes try to purchase multiple mobile phone number cards and use them to register multiple Xiaohongshu accounts. However, this approach has some limitations, because purchasing multiple mobile phone number cards is cumbersome and costly. 2. Use email to register. In addition to your mobile phone number, your email can also be used to register a Xiaohongshu account. Users can prepare multiple email addresses and then use these email addresses to register accounts. but
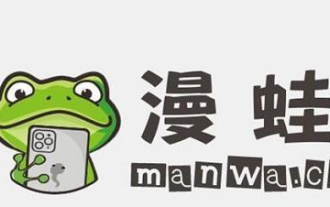
On the Manwa Comics platform, there are rich comic resources waiting for everyone to explore. As long as you easily enter the official platform of Manwa Comics, you can enjoy all kinds of wonderful comic works. Everyone can easily find their favorite comics to read according to their own preferences. So how to register the official account of Manwa Comics? The editor of this website will bring you this detailed tutorial guide, hoping to help everyone in need. Manwa Comics-Official entrance: https://fuw11.cc/mw666 Manwa Comics app download address: https://www.siemens-home.cn/soft/74440.html Manwa Comics non-mainland area entrance: https: /
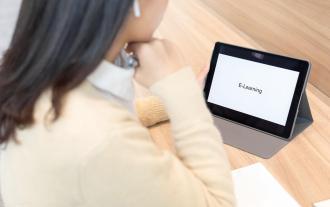
I don’t know if you have such an experience. Your mobile phone often receives some inexplicable text messages, or registration information for some websites or other verification information. In fact, our mobile phone number may be bound to many unfamiliar websites, and we ourselves Even if you don’t know, what I will share with you today is to teach you how to unbind all unfamiliar websites with one click. Step 1: Open the number service platform. This technique is very practical. The steps are as follows: Open WeChat, click the plus icon in the search box, select Add Friend, and then enter the code number service platform to search. We can see that there is a number service platform. Of course, it belongs to a public institution and was launched by the National Institute of Information and Communications Technology. It can help everyone unbind mobile phone number information with one click. Step 2: Check whether the phone has been marked for me
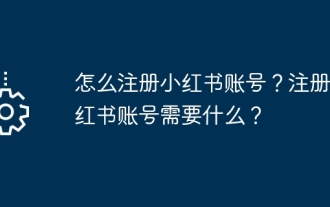
Xiaohongshu, a social platform integrating life, entertainment, shopping and sharing, has become an indispensable part of the daily life of many young people. So, how to register a Xiaohongshu account? 1. How to register a Xiaohongshu account? 1. Open the Xiaohongshu official website or download the Xiaohongshu APP. Click the "Register" button below and you can choose different registration methods. Currently, Xiaohongshu supports registration with mobile phone numbers, email addresses, and third-party accounts (such as WeChat, QQ, Weibo, etc.). 3. Fill in the relevant information. According to the selected registration method, fill in the corresponding mobile phone number, email address or third-party account information. 4. Set a password. Set a strong password to keep your account secure. 5. Complete the verification. Follow the prompts to complete mobile phone verification or email verification. 6. Perfect the individual
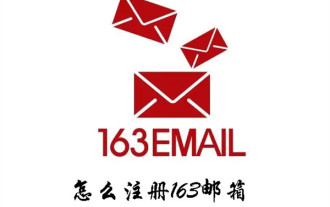
Some users find that they do not have an account when they want to use 163 mailbox. So what should they do if they need to register an account at this time? Now let’s take a look at the 163 email registration method brought by the editor. 1. First, search the 163 Email official website in the browser and click [Register a new account] on the page; 2. Then select [Free Email] or [VIP Email]; 3. Finally, after selecting, fill in the information and click [Now Just register];
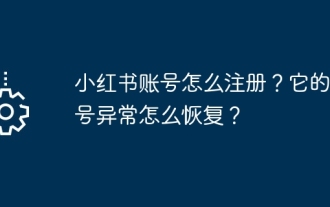
As one of the most popular lifestyle sharing platforms in the world, Xiaohongshu has attracted a large number of users. So, how to register a Xiaohongshu account? This article will introduce you to the Xiaohongshu account registration process in detail, and answer the question of how to recover Xiaohongshu account abnormalities. 1. How to register a Xiaohongshu account? 1. Download the Xiaohongshu APP: Search and download the Xiaohongshu APP in the mobile app store, and open it after the installation is complete. 2. Register an account: After opening the Xiaohongshu APP, click the "Me" button in the lower right corner of the homepage, and then select "Register". 3. Fill in the registration information: Fill in the mobile phone number, set password, verification code and other registration information according to the prompts. 4. Complete personal information: After successful registration, follow the prompts to complete personal information, such as name, gender, birthday, etc. 5. Settings
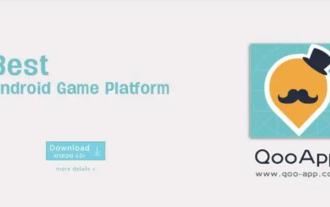
qooapp is a software that can download many games, so how to register an account? Users need to click the "Register" button if they don't have a pass yet, and then choose a registration method. This account registration method introduction is enough to tell you how to operate it. The following is a detailed introduction, so take a look. How to register a qooapp account? Answer: Click to register, and then choose a registration method. Specific methods: 1. After entering the login interface, click below. Don’t have a pass yet? Apply now. 2. Then choose the login method you need. 3. You can use it directly after that. Official website registration: 1. Open the website https://apps.ppaooq.com/ and click on the upper right corner to register. 2. Select registration
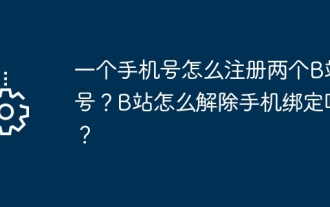
Bilibili (Bilibili), as a video sharing website very popular among Chinese young people, has attracted a large number of users. Some users hope to have two Bilibili accounts so that they can be managed and used separately. So, how to register two B-site numbers with one mobile phone number? This article will focus on this issue and how to unbind the mobile phone. 1. How to register two B-site numbers with one mobile phone number? 1. Register a new account: First, open the Bilibili App on your mobile phone or log in to the official website, click the "Register" button, and select the registration method. You can use your mobile phone number, email or third-party account (such as WeChat, QQ, etc.) to register. 2. When registering an account, please fill in the necessary information according to the system prompts, including mobile phone number, verification code, and set password. Be sure to use different accounts
