Interpreting PHP array sorting
PHP has a set of powerful function libraries. Let’s take a look at the specific usage of each array sorting function. The screenshots below are from the PHP7.0 manual.
- sort( ) — Sort the array from low to high. This function is the root function of all sorting functions;
bool sort ( array &$array [, int $sort_flags = SORT_REGULAR ] )
Two parameters, in addition to the array, the second optional parameter sort_flags changes the sorting through optional type flags Behavior.
<code><span><span><?php</span><span>$auto</span> = <span>array</span>(<span>"bens2"</span>,<span>"honda3"</span>,<span>"BMW4"</span>,<span>"aens1"</span>,<span>"BMW5"</span>); sort(<span>$auto</span>,SORT_STRING|SORT_FLAG_CASE);<span>//不区分大小写排序字符串</span><span>foreach</span>(<span>$auto</span><span>as</span><span>$key</span>=><span>$val</span>) { <span>echo</span><span>"auto["</span>.<span>$key</span>.<span>"]="</span>.<span>$val</span>.<span>"\n"</span>; <span>//输出auto[0]=BMW4 auto[1]=BMW5 auto[2]=aens1 auto[3]=bens2 auto[4]=honda3</span> }</span></span></code>
- rsort — Sort the array in reverse, the parameter list is the same as sort, and the return value is the same as
<code><span><span><?php</span><span>$auto</span> = <span>array</span>(<span>"bens2"</span>,<span>"honda3"</span>,<span>"BMW4"</span>,<span>"aens1"</span>,<span>"BMW5"</span>); rsort(<span>$auto</span>,SORT_STRING|SORT_FLAG_CASE);<span>//不区分大小写排序字符串</span><span>foreach</span>(<span>$auto</span><span>as</span><span>$key</span>=><span>$val</span>) { <span>echo</span><span>"auto["</span>.<span>$key</span>.<span>"]="</span>.<span>$val</span>.<span>"\n"</span>; <span>//输出auto[0]=honda3 auto[1]=BMW5 auto[2]=BMW4 auto[3]=bens2 auto[4]=aens1</span> }</span></span></code>
- asort() — Sort the array and maintain the index relationship, the parameters and return value are the same as above, the explanation in the manual is "This function sorts the array, and the index of the array remains associated with the unit. It is mainly used to sort associative arrays where the order of the units is important." Personally, I think it and sort() focus on sorting associative arrays. Simply put, the sorting rules are based on values, not keys. This is easily distinguished from ksort().
<code><span><span><?php</span><span>$auto</span> = <span>array</span>(<span>"a"</span>=><span>"bens2"</span>,<span>"b"</span>=><span>"honda3"</span>,<span>"c"</span>=><span>"BMW4"</span>,<span>"d"</span>=><span>"aens1"</span>,<span>"e"</span>=><span>"BMW5"</span>); asort(<span>$auto</span>,SORT_STRING|SORT_FLAG_CASE);<span>//不区分大小写排序字符串</span><span>foreach</span>(<span>$auto</span><span>as</span><span>$key</span>=><span>$val</span>) { <span>echo</span><span>"auto["</span>.<span>$key</span>.<span>"]="</span>.<span>$val</span>.<span>"\n"</span>; <span>//输出auto[d]=aens1 auto[a]=bens2 auto[c]=BMW4 auto[e]=BMW5 auto[b]=honda3</span> }</span></span></code>
- arsort() — Reverse sort the array and maintain index relationship, it is the inverse sort of asort().
<code><span><span><?php</span><span>$auto</span> = <span>array</span>(<span>"a"</span>=><span>"bens2"</span>,<span>"b"</span>=><span>"honda3"</span>,<span>"c"</span>=><span>"BMW4"</span>,<span>"d"</span>=><span>"aens1"</span>,<span>"e"</span>=><span>"BMW5"</span>); arsort(<span>$auto</span>,SORT_STRING|SORT_FLAG_CASE);<span>//不区分大小写排序字符串</span><span>foreach</span>(<span>$auto</span><span>as</span><span>$key</span>=><span>$val</span>) { <span>echo</span><span>"auto["</span>.<span>$key</span>.<span>"]="</span>.<span>$val</span>.<span>"\n"</span>; <span>//输出auto[b]=honda3 auto[e]=BMW5 auto[c]=BMW4 auto[a]=bens2 auto[d]=aens1</span> }</span></span></code>
- ksort() - Sort the array by key name. The manual explains that "sort the array by key name and retain the association between key name and data. This function is mainly used for associative arrays." The parameters and return value are the same as sort ()
<code><span><span><?php</span><span>$auto</span> = <span>array</span>(<span>"b"</span>=><span>"bens2"</span>,<span>"a"</span>=><span>"honda3"</span>,<span>"d"</span>=><span>"BMW4"</span>,<span>"c"</span>=><span>"aens1"</span>,<span>"e"</span>=><span>"BMW5"</span>); ksort(<span>$auto</span>,SORT_STRING|SORT_FLAG_CASE);<span>//不区分大小写排序字符串</span><span>foreach</span>(<span>$auto</span><span>as</span><span>$key</span>=><span>$val</span>) { <span>echo</span><span>"auto["</span>.<span>$key</span>.<span>"]="</span>.<span>$val</span>.<span>"\n"</span>; <span>//输出auto[a]=honda3 auto[b]=bens2 auto[c]=aens1 auto[d]=BMW4 auto[e]=BMW5</span> }</span></span></code>
- krsort — Sort the array in reverse order by key name. The parameters and return values are the same as above.
<code><span><span><?php</span><span>$auto</span> = <span>array</span>(<span>"b"</span>=><span>"bens2"</span>,<span>"a"</span>=><span>"honda3"</span>,<span>"d"</span>=><span>"BMW4"</span>,<span>"c"</span>=><span>"aens1"</span>,<span>"e"</span>=><span>"BMW5"</span>); ksort(<span>$auto</span>,SORT_STRING|SORT_FLAG_CASE);<span>//不区分大小写排序字符串</span><span>foreach</span>(<span>$auto</span><span>as</span><span>$key</span>=><span>$val</span>) { <span>echo</span><span>"auto["</span>.<span>$key</span>.<span>"]="</span>.<span>$val</span>.<span>"\n"</span>; <span>//输出auto[a]=honda3 auto[b]=bens2 auto[c]=aens1 auto[d]=BMW4 auto[e]=BMW5</span> }</span></span></code>
- natsort — Use the "natural sorting" algorithm to sort the array. The manual explains that "This function implements a sum People usually sort alphanumeric strings using the same sorting algorithm and retaining the original key/value association. This is called "natural sorting" and is different from the sorting method of sort() which takes only one parameter. That is, the parameters passed in.
shuffle() — shuffles the array, it will delete the key names, not just the key names. Its parameters are only the array variables passed in, and the return value is true or false
<code><span><span><?php</span><span>$auto</span> = <span>array</span>(<span>"b"</span>=><span>"bens2"</span>,<span>"e"</span>=><span>"bens20"</span>,<span>"a"</span>=><span>"bens31"</span>,<span>"d"</span>=><span>"bens41"</span>,<span>"c"</span>=><span>"bens1"</span>,<span>"e"</span>=><span>"bens15"</span>); sort(<span>$auto</span>,SORT_STRING|SORT_FLAG_CASE);<span>//不区分大小写排序字符串</span><span>echo</span><span>"sort排序:<br>"</span>; <span>foreach</span>(<span>$auto</span><span>as</span><span>$key</span>=><span>$val</span>) { <span>echo</span><span>"auto["</span>.<span>$key</span>.<span>"]="</span>.<span>$val</span>.<span>"<br>"</span>; } <span>$auto1</span> = <span>array</span>(<span>"b"</span>=><span>"bens2"</span>,<span>"e"</span>=><span>"bens20"</span>,<span>"a"</span>=><span>"bens31"</span>,<span>"d"</span>=><span>"bens41"</span>,<span>"c"</span>=><span>"bens1"</span>,<span>"e"</span>=><span>"bens15"</span>); natsort(<span>$auto1</span>);<span>//参数只有一个</span><span>echo</span><span>"natsort排序:<br>"</span>; <span>foreach</span>(<span>$auto1</span><span>as</span><span>$key</span>=><span>$val</span>) { <span>echo</span><span>"auto["</span>.<span>$key</span>.<span>"]="</span>.<span>$val</span>.<span>"<br>"</span>; } <span>//输出</span><span>/* sort排序: auto[0]=bens1 auto[1]=bens15 auto[2]=bens2 auto[3]=bens31 auto[4]=bens41 natsort排序: auto[c]=bens1 auto[b]=bens2 auto[e]=bens15 auto[a]=bens31 auto[d]=bens41 */</span></span></span></code>
Copy after login').addClass('pre-numbering').hide();
$(this).addClass('has-numbering').parent().append($numbering);
for (i = 1; i

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


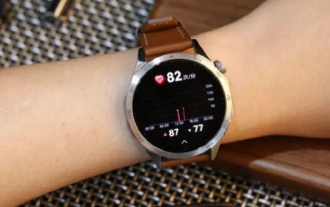
Many users will choose the Huawei brand when choosing smart watches. Among them, Huawei GT3pro and GT4 are very popular choices. Many users are curious about the difference between Huawei GT3pro and GT4. Let’s introduce the two to you. . What are the differences between Huawei GT3pro and GT4? 1. Appearance GT4: 46mm and 41mm, the material is glass mirror + stainless steel body + high-resolution fiber back shell. GT3pro: 46.6mm and 42.9mm, the material is sapphire glass + titanium body/ceramic body + ceramic back shell 2. Healthy GT4: Using the latest Huawei Truseen5.5+ algorithm, the results will be more accurate. GT3pro: Added ECG electrocardiogram and blood vessel and safety
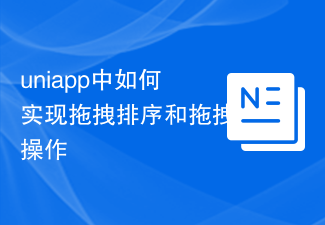
Uniapp is a cross-platform development framework. Its powerful cross-end capabilities allow developers to develop various applications quickly and easily. It is also very simple to implement drag-and-drop sorting and drag-and-drop operations in Uniapp, and it can support drag-and-drop operations of a variety of components and elements. This article will introduce how to use Uniapp to implement drag-and-drop sorting and drag-and-drop operations, and provide specific code examples. The drag-and-drop sorting function is very common in many applications. For example, it can be used to implement drag-and-drop sorting of lists, drag-and-drop sorting of icons, etc. Below we list
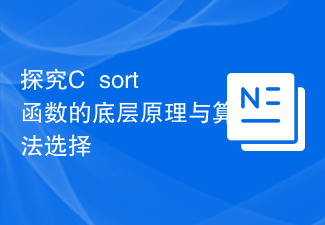
The bottom layer of the C++sort function uses merge sort, its complexity is O(nlogn), and provides different sorting algorithm choices, including quick sort, heap sort and stable sort.
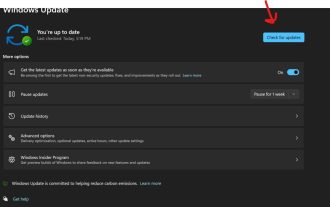
Why Snipping Tool Not Working on Windows 11 Understanding the root cause of the problem can help find the right solution. Here are the top reasons why the Snipping Tool might not be working properly: Focus Assistant is On: This prevents the Snipping Tool from opening. Corrupted application: If the snipping tool crashes on launch, it might be corrupted. Outdated graphics drivers: Incompatible drivers may interfere with the snipping tool. Interference from other applications: Other running applications may conflict with the Snipping Tool. Certificate has expired: An error during the upgrade process may cause this issu simple solution. These are suitable for most users and do not require any special technical knowledge. 1. Update Windows and Microsoft Store apps
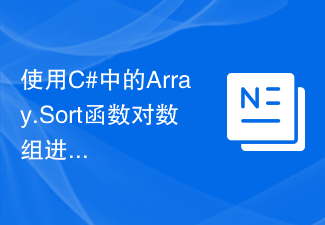
Title: Example of using the Array.Sort function to sort an array in C# Text: In C#, array is a commonly used data structure, and it is often necessary to sort the array. C# provides the Array class, which has the Sort method to conveniently sort arrays. This article will demonstrate how to use the Array.Sort function in C# to sort an array and provide specific code examples. First, we need to understand the basic usage of the Array.Sort function. Array.So
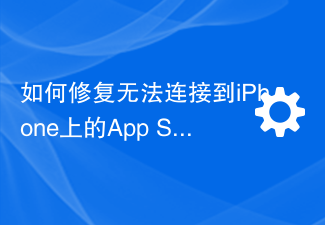
Part 1: Initial Troubleshooting Steps Checking Apple’s System Status: Before delving into complex solutions, let’s start with the basics. The problem may not lie with your device; Apple's servers may be down. Visit Apple's System Status page to see if the AppStore is working properly. If there's a problem, all you can do is wait for Apple to fix it. Check your internet connection: Make sure you have a stable internet connection as the "Unable to connect to AppStore" issue can sometimes be attributed to a poor connection. Try switching between Wi-Fi and mobile data or resetting network settings (General > Reset > Reset Network Settings > Settings). Update your iOS version:
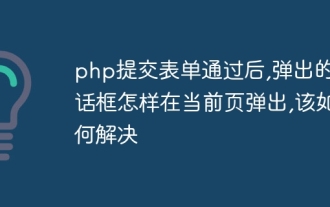
php提交表单通过后,弹出的对话框怎样在当前页弹出php提交表单通过后,弹出的对话框怎样在当前页弹出而不是在空白页弹出?想实现这样的效果:而不是空白页弹出:------解决方案--------------------如果你的验证用PHP在后端,那么就用Ajax;仅供参考:HTML code

Example In this example, we first look at the usage of list.sort() before continuing. Here, we have created a list and sorted it in ascending order using sort() method - #CreatingaListmyList=["Jacob","Harry","Mark","Anthony"]#DisplayingtheListprint("List=",myList)#SorttheListsinAscendingOrdermyList .sort(
