usb interface type an improved UBB class
/*
If reprinted, please indicate the author
Original author: He Zhiqiang
Improvement: SonyMusic[ sonymusic@163.net ]
File: ubb.php
Remarks: It is said to be an improvement, but in fact the core function parse( ) has been completely rewritten, and the ideas are different.
But it is still inspired by He Zhiqiang’s example, and the test examples and several functions such as URLCHECK also follow He Zhiqiang’s program. Thank you He Zhiqiang.
There is no color function yet, but I will add it.
If there are any bugs or inconveniences in the program, please email me.
Thank you!
Improved functions:
UBB encoding of strings. This class currently only supports the following simple and practical encodings:
1. URL link
[url] http://phpuser.com/ [/url]
http The :// header is not required. For example, [url]phpuser.com[/url] is also acceptable.
2. Email link
[email] sonymusic@163.net [/email]
3. Picture link
[img] http://www.phpchina.com/images/logo.gif [/img]
Same The URL link is the same, and the previous http is optional.
4. In terms of text
[b]Bold font[/b]
[i]Italic font[/i]
[u]Underline[/u]
[h1]Title No. 1[/h1] .. . [h6] Title word No. 6[/h6]
[sup][/sup]
[sub][/sub]
[tt][/tt]
[s][/s]
[strike][/ strike]
[em][/em]
[strong][/strong]
[code][/code]
[samp][/samp]
[kbd][/kbd]
[var][/var]
[dfn][/dfn]
[cite][/cite]
[small][/small]
[big][/big]
[blink][/blink]
Pay attention to the following points:
1. url , email, img and other tags are not case-sensitive.
2. TAB keys are not allowed in tags, but spaces are allowed.
3. This class needs to call htmlencode, htmlencode4textarea, emailcheck function and urlcheck class.
4. After modification, nesting is supported, but nesting of the three tags url, email, and img is not allowed.
Technical information:
Ultimate Bulletin Board
http://www.ultimatebb.com/
What is UBB Code
http://www.scriptkeeper.com/ubb/ubbcode.html
*/
include("urlcheck.php ");
include("otherfunc.php"); //The contents of these two files are attached at the end.
//ubbcode类
class ubbcode{
var $call_time=0;
//可处理标签及处理函数对应表
var $tags = array( //小写的标签 => 对应的处理函数
'url' => '$this->url',
'email' => '$this->email',
'img' => '$this->img',
'b' => '$this->simple',
'i' => '$this->simple',
'u' => '$this->simple',
'tt' => '$this->simple',
's' => '$this->simple',
'strike' => '$this->simple',
'h1' => '$this->simple',
'h2' => '$this->simple',
'h3' => '$this->simple',
'h4' => '$this->simple',
'h5' => '$this->simple',
'h6' => '$this->simple',
'sup' => '$this->simple',
'sub' => '$this->simple',
'em' => '$this->simple',
'strong' => '$this->simple',
'code' => '$this->simple',
'samp' => '$this->simple',
'kbd' => '$this->simple',
'var' => '$this->simple',
'dfn' => '$this->simple',
'cite' => '$this->simple',
'small' => '$this->simple',
'big' => '$this->simple',
'blink' => '$this->simple'
);
//url裢接属性
var $attr_url;
//url合法性检查对象
var $urlcheck;
function ubbcode($attr_url){
$this->attr_url = ''.$attr_url;
$this->urlcheck = new urlcheck();
}
//对$str进行UBB编码解析
function parse($str){
$this->call_time++;
$parse = ''.htmlencode($str);
$ret = '';
while(true){
$eregi_ret=eregi("[[#]{0,1}[[:alnum:]]{1,7}]",$parse,$eregi_arr); //查找[xx]
if(!$eregi_ret){
$ret .= $parse;
break; //如果没有,返回
}
$pos = @strpos ($parse,$eregi_arr[0]);
$tag_len=strlen($eregi_arr[0])-2;//标记长度
$tag_start=substr($eregi_arr[0],1,$tag_len);
$tag=strtolower($tag_start);
if((($tag=="url") or ($tag=="email") or ($tag=="img")) and ($this->call_time>1)){
echo $this->call_time."
";
return $parse;//如果不能是不能嵌套的标记,直接返回
}
$parse2 = substr($parse,0,$pos);//标记之前
$parse = substr($parse,$pos+$tag_len+2);//标记之后
if(!isset($this->tags[$tag])){
echo "$tag_start
";
$ret .= $parse2.'['.$tag_start.']';
continue;//如果是不支持的标记
}
//查找对对应的结束标记
$eregi_ret=eregi("[/".$tag."]",$parse,$eregi_arr);
if(!$eregi_ret){
$ret .= $parse2.'['.$tag_start.']';
continue;//如果没有对应该的结束标记
}
$pos=strpos($parse,$eregi_arr[0]);
$value=substr($parse,0,$pos);//这是起止标记之间的内容
$tag_end=substr($parse,$pos+2,$tag_len);
$parse=substr($parse,$pos+$tag_len+3);//结束标记之后的内容
if(($tag!="url") and ($tag!="email") and ($tag!="img")){
$value=$this->parse($value);
}
$ret .= $parse2;
eval('$ret .= '.$this->tags[$tag].'("'.$tag_start.'","'.$tag_end.'","'.$value.'");');
}
$this->call_time--;
return $ret;
}
function simple($start,$end,$value){
return '<'.$start.'>'.$value.''.$end.'>';
}
function url($start,$end,$value){
$trim_value=trim($value);
if (strtolower(substr($trim_value,0,7))!="http://")
$trim_value="http://".$trim_value;
if($this->urlcheck->check($trim_value)) return 'attr_url.'>'.$value.'';
else return '['.$start.']'.$value.'[/'.$end.']';
}
function email($start,$end,$value){
if(emailcheck($value)) return ''.$value.'';
else return '['.$start.']'.$value.'[/'.$end.']';
}
function img($start,$end,$value){
$trim_value=trim($value);
if ((strtolower(substr($trim_value,0,7))!="http://") or ($this->urlcheck->check($trim_value)))
return '';
else return '['.$start.']'.$value.'[/'.$end.']';
}
}
//Test
echo '';
echo '
echo '';
echo '< ;form action="'.str2url($PATH_INFO).'" method="post">';
echo '
';
echo '';
echo '';
if( isset($ubb)){
$ubbcode = new ubbcode('target="_blank"');
echo '
'.$ubbcode->parse($ubb);
}
echo '< /body>';
echo '';
?>
Contents of file urlcheck.php:
//urlcheck.php
class urlcheck{
var $regex = array(/ /Protocol name (note that it must be written in lowercase here) => Corresponding regular expression
'ftp' => '$this->ftpurl',
'file' => '$this->fileurl' ,
'http' => '$this->httpurl',
'https' => '$this->httpurl',
'gopher' => '$this->gopherurl',
'news' => '$this->newsurl',
'nntp' => '$this->nntpurl',
'telnet' => '$this->telneturl',
'wais ' => '$this->waisurl'
);
var $lowalpha;
var $hialpha;
var $alpha;
var $digit;
var $safe;
var $extra;
var $national;
var $punctuation;
var $reserved;
var $hex;
var $escape;
var $unreserved;
var $uchar;
var $xchar;
var $user;
var $port;
var $hostnumber;
var $alphadigit;
var $toplabel;
var $domainlabel;
var $hostname;
var $host;
var $hostport;
var $login;
//ftp
var $ftptype;
var $fsegment;
var $fpath;
var $ftpurl;
//file
var $fileurl;
//http,https
var $search;
var $hsegment;
var $hpath;
var $httpurl;
//gopher
var $gopher_string;
var $selector;
var $gtype;
var $gopherurl;
//news
var $article;
var $group;
var $ grouppart;
var $newsurl;
//nntp
var $nntpurl;
//telnet
var $telneturl;
//wais
var $wpath;
var $wtype;
var $database;
var $waisdoc;
var $waisindex;
var $waisdatabase;
var $waisurl;
function check($url){
$pos = @strpos ($url,':',1);
if($pos<1) return false;
$prot = substr($url,0,$pos);
if(!isset($this->regex[$prot])) return false;
eval('$regex = '.$this-> regex[$prot].';');
return ereg('^'.$regex.'$',$url);
}
function urlcheck(){
$this->lowalpha = '[a-z] ';
$this->hialpha = '[A-Z]';
$this->alpha = '('.$this->lowalpha.'|'.$this->hialpha.')';
$this->digit = '[0-9]';
$this->safe = '[$.+_-]';
$this->extra = '[*()'!, ]';
$this->national = '([{}|^~`]|[|])';
$this->punctuation = '[<>#%"]';
$ this->reserved = '[?;/: @&= ]';
$this->hex = '('.$this->digit.'|[a-fA-F])';
$this->escape = '(%'.$this->hex.'{2})';
$this->unreserved = '('.$this->alpha.'|'.$this->digit.'|'.$this->safe.'|'.$this->extra.')';
$this->uchar = '('.$this->unreserved.'|'.$this->escape.')';
$this->xchar = '('.$this->unreserved.'|'.$this->reserved.'|'.$this->escape.')';
$this->digits = '('.$this->digit.'+)';
$this->urlpath = '('.$this->xchar.'*)';
$this->password = '(('.$this->uchar.'|[?;&=]'.')*)';
$this->user = '(('.$this->uchar.'|[?;&=]'.')*)';
$this->port = $this->digits;
$this->hostnumber = '('.$this->digits.'.'.$this->digits.'.'.$this->digits.'.'.$this->digits.')';
$this->alphadigit = '('.$this->alpha.'|'.$this->digit.')';
$this->toplabel = '('.$this->alpha.'|('.$this->alpha.'('.$this->alphadigit.'|-)*'.$this->alphadigit.'))';
$this->domainlabel = '('.$this->alphadigit.'|('.$this->alphadigit.'('.$this->alphadigit.'|-)*'.$this->alphadigit.'))';
$this->hostname = '(('.$this->domainlabel.'.)*'.$this->toplabel.')';
$this->host = '('.$this->hostname.'|'.$this->hostnumber.')';
$this->hostport = '('.$this->host.'(:'.$this->port.')?)';
$this->login = '(('.$this->user.'(:'.$this->password.')?@)?'.$this->hostport.')';
$this->ftptype = '[aidAID]';
$this->fsegment = '(('.$this->uchar.'|[?: @&= ])*)';
$this->fpath = '('.$this->fsegment.'(/'.$this->fsegment.')*)';
$this->ftpurl = '([fF][tT][pP]://'.$this->login.'(/'.$this->fpath.'(;[tT][yY][pP][eE]='.$this->ftptype.')?)?)';
$this->fileurl = '([fF][iI][lL][eE]://('.$this->host.'|[lL][oO][cC][aA][lL][hH][oO][sS][tT])?/'.$this->fpath.')';
$this->search = '(('.$this->uchar.'|[;: @&= ])*)';
$this->hsegment = '(('.$this->uchar.'|[;: @&= ])*)';
$this->hpath = '('.$this->hsegment.'(/'.$this->hsegment.')*)';
$this->httpurl = '([hH][tT][tT][pP][sS]?://'.$this->hostport.'(/'.$this->hpath.'([?]'.$this->search.')?)?)';
$this->gopher_string = '('.$this->xchar.'*)';
$this->selector = '('.$this->xchar.'*)';
$this->gtype = $this->xchar;
$this->gopherurl = '([gG][oO][pP][hH][eE][rR]://'.$this->hostport.'(/('.$this->gtype.'('.$this->selector.'(%09'.$this->search.'(%09'.$this->gopher_string.')?)?)?)?)?)';
$this->article = '(('.$this->uchar.'|[;/?:&=]) +@'.$this- >host.')';
$this->group = '('.$this->alpha.'('.$this->alpha.'|'.$this->digit.'|[-.+_])*)';
$this->grouppart = '([*]|'.$this->group.'|'.$this->article.')';
$this->newsurl = '([nN][eE][wW][sS]:'.$this->grouppart.')';
$this->nntpurl = '([nN][nN][tT][pP]://'.$this->hostport.'/'.$this->group.'(/'.$this->digits.')?)';
$this->telneturl = '([tT][eE][lL][nN][eE][tT]://'.$this->login.'/?)';
$this->wpath = '('.$this->uchar.'*)';
$this->wtype = '('.$this->uchar.'*)';
$this->database = '('.$this->uchar.'*)';
$this->waisdoc = '([wW][aA][iI][sS]://'.$this->hostport.'/'.$this->database.'/'.$this->wtype.'/'.$this->wpath.')';
$this->waisindex = '([wW][aA][iI][sS]://'.$this->hostport.'/'.$this->database.'[?]'$this->search.')';
$this->waisdatabase = '([wW][aA][iI][sS]://'.$this->hostport.'/'.$this->database.')';
$this->waisurl = '('.$this->waisdatabase.'|'.$this->waisindex.'|'.$this->waisdoc.')';
}
}
?>
文件otherfunc.php的内容:
//otherfunc.php
function htmlencode($str){
$str = (string)$str;
$ret = '';
$len = strlen($str);
$nl = false;
for($i=0;$i<$len;$i++){
$chr = $str[$i];
switch($chr){
case '<':
$ret .= '<';
$nl = false;
break;
case '>':
$ret .= '>';
$nl = false;
break;
case '"':
$ret .= '"';
$nl = false;
break;
case '&':
$ret .= '&';
$nl = false;
break;
/*
case ' ':
$ret .= ' ';
$nl = false;
break;
*/
case chr(9):
$ret .= ' ';
$nl = false;
break;
case chr(10):
if($nl) $nl = false;
else{
$ret .= '
';
$nl = true;
}
break;
case chr(13):
if($nl) $nl = false;
else{
$ret .= '
';
$nl = true;
}
break;
default:
$ret .= $chr;
$nl = false;
break;
}
}
return $right;
}
function htmlencode4textarea($str){
$str = (string)$str;
$right = '';
$len = strlen($str);
for($i=0;$i<$len;$i++){
$chr = $str[$i];
switch($chr){
case '<':
$ret .= '<';
break;
case '>':
$ret .= '>';
break;
case '"':
$ret .= '"';
break;
case '&':
$ret .= '&';
break;
case ' ':
$ret .= ' ';
break;
case chr(9):
$ret .= ' ';
break;
default:
$ret .= $chr;
break;
}
}
return $right;
}
function emailcheck($email){
$ret=false;
if(strstr($email, '@' ) && strstr($email, '.')){
if(eregi("^([_a-z0-9]+([._a-z0-9-] +)*)@([a-z0-9]{2,}(.[a-z0-9-]{2,})*.[a-z]{2,3})$", $email)) {
$ret=true;
}
}
return $right;
}
function str2url($path){
return eregi_replace("%2f","/",urlencode($path));
}
?>
下载就introduced usb interface type 一个improved UBB class,公司了usb interface type 内容,最好对PHP tutorial have friends who are interested have some help。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
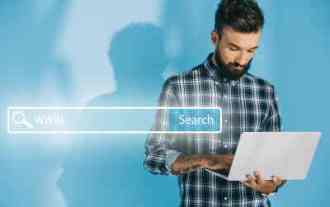
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
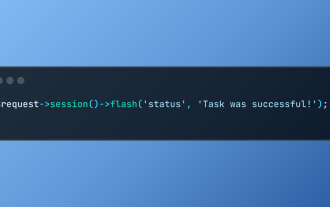
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
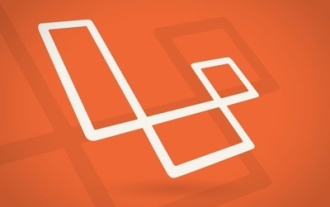
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
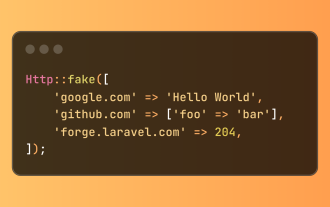
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
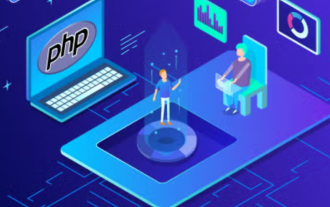
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
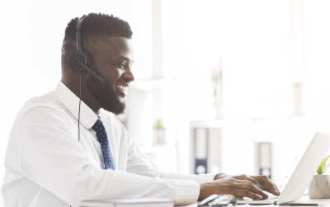
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
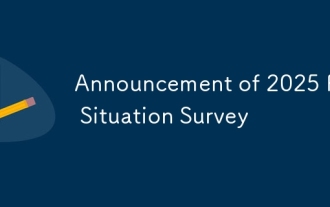
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio
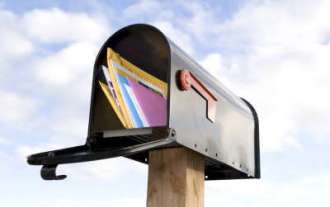
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov
