


createcompatibledc code that uses bcompiler to encrypt PHP files
Instructions for use:
//Loading function
include_once('phpCodeZip.php');
//Create an encrypted file (sourceDir is the php file directory to be encrypted, targetDir is the encrypted file directory)
$encryption = new PhoCodeZip(' sourceDir','targetDir');
//Execute line encryption
$encryption->zip();
phpCodeZip.php source code download
phpCodeZip.rar
phpCodeZip.php source code content
Copy code The code is as follows :
/*
* @license: MIT & GPL
*/
class PhpCodeZip{
//The source folder to be compressed and encrypted
var $sourceDir = '.';
//The folder to be compressed and encrypted
var $targetDir = 'tmp';
//Whether to encrypt
var $bcompiler = true;
//Whether to remove blank comments and line breaks
var $strip = true;
//Source folder file path array
var $ sourcefilePaths = array();
//array of destination folder file paths
var $targetPaths = array();
//folder size before compression and encryption
var $sizeBeforeZip = null;
//data after compression and encryption Clip size
var $sizeAfterZip = null;
//Output of line break
var $newline = '';
/**
* Constructor
*
* @param string $sourceDir source folder
* @param string $targetDir destination folder
* @param boolean $bcompiler Whether to encrypt
* @param boolean $strip Whether to remove blank comments and line breaks
* @return boolean
*/
public function PhpCodeZip($sourceDir='.',$targetDir=' tmp' ,$bcompiler=true,$strip=true){
//Configure initial variables
$this->sourceDir = $sourceDir;
$this->targetDir = $targetDir;
$this->bcompiler = $bcompiler ;
//Check whether the source data exists
if(!is_dir($this->sourceDir)){
die('The specified source folder'.$this->sourceDir.' does not exist, please reset ');
} else {
//If the specified destination folder exists, cut it off and try again
if(is_dir($this->targetDir)){
echo '[Initialize destination folder]'.$ this ->newline.$this->newline;
$this->cleanDir($this->targetDir,true);
}
//Create a destination folder with the same structure as the source folder
mkdir( $ this->targetDir,0777);
$dir_paths = $this->getPaths($this->sourceDir,'*',GLOB_ONLYDIR);
foreach($dir_paths as $key => $path){
$path = explode('/',$path);
$path[0] = $this->targetDir;
echo '=> '.join('/',$path).$this-> ; newline;
mkdir(join('/',$path),0777);
}
//Get the file path list of the source folder
$this->sourcefilePaths = $this->getPaths($this- > ;sourceDir,'*');
//Match the file path list of the corresponding destination
foreach($this->sourcefilePaths as $key => $path){
//Set the destination folder file path
$ path = explode('/',$path);
$path[0] = $this->targetDir;
$this->targetPaths[$key] = join('/',$path);
}
//Record the folder size before execution
$this->sizeBeforeZip = $this->getSizeUnit($this->getDirSize($this->sourceDir),2);
echo $this-> newline.$this->newline;
}
}
/**
* Perform compression and encryption
* @return boolean
*/
public function zip(){
$this->newline = '';
echo '【Start encryption process】( Folder size: '.$this->sizeBeforeZip.')'.$this->newline.$this->newline;
//Compress the source file
foreach($this->sourcefilePaths as $ key => $path){
if(is_file($path)){
//Get file information
$pathInfo = pathInfo($path);
echo 'Read source file:'.$path.$this- >newline;
//Get the compressed content
echo '=>Remove blank annotations.....';
if($this->strip && $pathInfo['extension'] == 'php'){
$fileAterZip = php_strip_whitespace($path);
} else {
$fileAterZip = file_get_contents($path);
}
echo 'Finished'.$this->newline;
//Get The compressed content is written to the destination location
$fp = fopen($this->targetPaths[$key],'w+');
echo '=>Write to the destination file..... ';
fwrite($fp,$fileAterZip);
fclose($fp);
echo 'Complete'.$this->newline;
//Whether to choose to encrypt
if($this->bcompiler && $pathInfo['extension'] == 'php'){
echo '=>Encrypt the original file.....';
//Copy the original file
$fh = fopen($this- >targetPaths[$key].'encrypt.php', "w");
bcompiler_write_header($fh);
bcompiler_write_file($fh, $this->targetPaths[$key]);
bcompiler_write_footer($fh) ;
fclose($fh);
//Delete the unencrypted original file
unlink($this->targetPaths[$key]);
//Rename the encrypted file
rename($this->targetPaths [$key].'encrypt.php',$this->targetPaths[$key]);
echo 'Finished'.$this->newline;
}
echo $this->newline.$this- >newline;
}
}
//Recalculate the compressed and encrypted folder size
$this->sizeAfterZip = $this->getSizeUnit($this->getDirSize($this->targetDir),2);
echo '[End Encryption program】'.$this->newline.$this->newline;
echo '"Report Information"'.$this->newline;
echo 'Source folder:'.$this->sourceDir .'('.$this->sizeBeforeZip.')'.$this->newline;
echo 'Destination folder:'.$this->targetDir.'('.$this->sizeAfterZip. ')'.$this->newline;
echo 'File size increase: +'.$this->getSizeUnit(($this->getDirSize($this->targetDir) - $this->getDirSize ($this->sourceDir))).$this->newline;
echo 'Total number of files:'.count($this->sourcefilePaths).'.$this->newline;
}
/**
* Delete all files in the directory
*
* @param string $dir The folder to be deleted
* @param boolean $deleteSelf Delete the folder at the same time
* @return void
*/
private function cleanDir($dir='.',$deleteSelf=true){
if(!$dh = @opendir($dir)) return;
while (($obj = readdir ($dh))) {
if($obj=='.' || $obj=='..') continue;
if (!@unlink($dir.'/'.$obj)) $this ->cleanDir($dir.'/'.$obj, true);
}
if ($deleteSelf){
closedir($dh);
@rmdir($dir);
}
}
/**
* Get the total file size of the folder
*
* @param string $dir The folder to be analyzed
* @return int byte
*/
private function getDirSize($dir='.'){
//Get the file path list
$filePaths = $this->getPaths($dir,'*');
//Initialize the counter
$sizeCounter = 0;
foreach($filePaths as $key => $path){
$sizeCounter = $sizeCounter + filesize($path);
}
return ($sizeCounter);
}
/**
* Get all matching paths of the folder
*
* @param string $start_dir The folder to be analyzed
* @return array File path array
*/
private function getPaths($sDir, $sPattern, $nFlags = NULL){
$sDir = escapeshellcmd($sDir);
$aFiles = glob("$sDir/$sPattern", $nFlags);
foreach (glob("$sDir/*", GLOB_ONLYDIR) as $sSubDir) {
$aSubFiles = $this->getPaths($sSubDir, $sPattern, $nFlags);
$aFiles = array_merge($aFiles, $aSubFiles) ;
}
return $aFiles;
}
/**
* File size unit conversion function
*
* @param int file size
* @param int number of decimal points
* @param boolean whether to cut the data into an array
* @return mix string or array
*/
public function getSizeUnit($size,$decimal=2,$split=false){
//Set unit sequence
$unit = array ('Bytes','KB','MB','GB','TB','PB','EB','ZB','YB');
//Initialization index
$flag = 0;
//Perform simplified division
while($size >= 1024){
$size = $size / 1024;
$flag++;
}
//Whether to separate the value from the unit
if($split){
$ sizeUnit = array(
'size' => number_format($size,$decimal),
'unit' => $unit[$flag]
);
} else {
$sizeUnit = (number_format($size, $ decimal)).$unit[$flag];
}
//Return size and unit
return ($sizeUnit);
}
}
The above introduces the code for createcompatibledc to use bcompiler to encrypt PHP files, including the content of createcompatibledc. I hope it will be helpful to friends who are interested in PHP tutorials.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


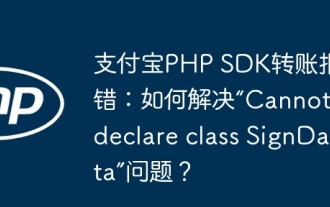
Alipay PHP...
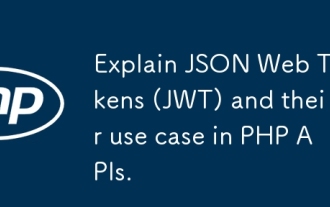
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
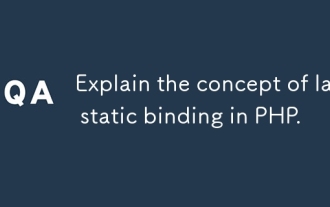
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
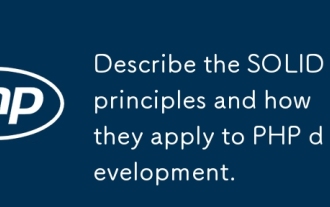
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
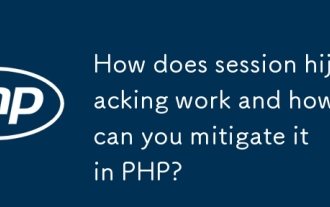
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
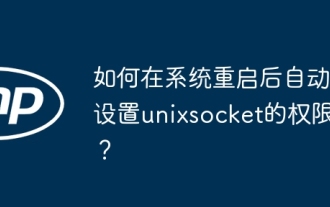
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
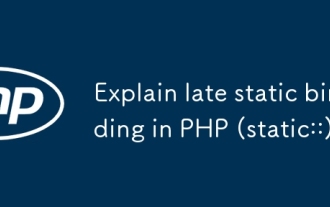
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
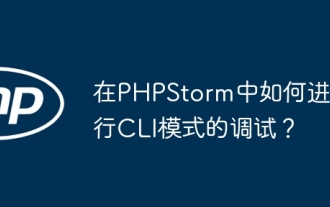
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
