Summary of jQuery data types (14)_jquery
In addition to the built-in datatypes in native JS, jQuery also includes some extended data types (virtual types), such as Selectors, Events, etc.
1. String
String is the most common and is supported by almost any high-level programming language and scripting language. For example, "Hello world!" is a string. The type of string is string. For example
var typeOfStr = typeof "hello world";//typeOfStr is "string"
1.1 String built-in methods
"hello".charAt(0) // "h"
"hello".toUpperCase() // "HELLO"
"Hello".toLowerCase() // "hello"
"hello".replace(/e|o/g, "x") // "hxllx"
"1,2,3".split(",") // ["1", "2", "3"]
1.2 length attribute: returns the character length, for example, "hello".length returns 5
1.3 Convert string to Boolean:
An empty string ("") defaults to false, while a non-empty string defaults to true (such as "hello").
2. Number
Number type, such as 3.1415926 or 1, 2, 3...
typeof 3.1415926 returns "number"
2.1 Number converted to Boolean:
If a Number value is 0, it defaults to false, otherwise it is true.
2.2 Since Number is implemented using double-precision floating point numbers, the following situation is reasonable:
0.1 + 0.2 // 0.30000000000000004
3. Math
The following method is similar to the static method of Math class in Java.
Math.PI // 3.141592653589793
Math.cos(Math.PI) // -1
3.1 Convert strings to numbers: parseInt and parseFloat methods:
parseInt("123") = 123 (using decimal conversion)
parseInt("010") = 8 (using octal conversion)
parseInt("0xCAFE") = 51966 (using hexadecimal conversion)
parseInt("010", 10) = 10 (specify decimal conversion)
parseInt("11", 2) = 3 (specify binary conversion)
parseFloat("10.10") = 10.1
3.2 Number to string
When you append Number to the string, you will get the string.
"" + 1 + 2; // "12"
"" + (1 + 2); // "3"
"" + 0.0000001; // "1e-7"
Or use forced type conversion:
String(1) + String(2); //"12"
String(1 + 2); //"3"
4. NaN and Infinity
If the parseInt method is called on a non-numeric string, NaN (Not a Number) will be returned. NaN is often used to detect whether a variable is of numeric type, as follows:
isNaN(parseInt("hello", 10)) // true
Infinity represents an infinitely large or infinitely small value, such as 1 / 0 // Infinity.
Calling the typeof operator on both NaN and Infinity returns "numuber".
Also NaN==NaN returns false, but Infinity==Infinity returns true.
5. Integer and Float
It is divided into integer type and floating point type.
6. BOOLEAN
Boolean type, true or false.
7. OBJECT
Everything in JavaScript is an object. Performing a typeof operation on an object returns "object".
var x = {}; var y = { name: "Pete", age: 15 };
For the above y object, you can use dots to obtain attribute values. For example, y.name returns "Pete" and y.age returns 15
7.1 Array Notation (array access method to access objects)
var operations = { increase: "++", decrease: "--" } var operation = "increase"; operations[operation] // "++"; operations["multiply"] = "*"; // "*"
The above operations["multiply"]="*"; adds a key-value pair to the operations object.
7.2 Object loop access: for-in
var obj = { name: "Pete", age: 15}; for(key in obj) { alert("key is "+[key]+", value is "+obj[key]); }
7.3 Any object, regardless of whether it has attributes or values, defaults to true
7.4 Prototype attribute of object
Use fn (alias of Prototype) in jQuery to dynamically add objects (functions) to jQuery Instances
var form = $("#myform"); form.clearForm; // undefined form.fn.clearForm = function() { return this.find(":input").each(function() { this.value = ""; }).end(); }; form.clearForm() // works for all instances of jQuery objects, because the new method was added
8. OPTIONS
Almost all jQuery plug-ins provide an API based on OPTIONS. OPTIONS is a JS object, which means that the object and its properties are optional. Allow customization.
For example, using Ajax to submit a form,
$("#myform").ajaxForm();//By default, the Action attribute value of the Form is used as the Ajax-URL, and the Method value is used as the submission type (GET/POST)
$("#myform").ajaxForm({ url: "mypage.php", type: "POST" }); // Covers the submitted URL and submission type
9. ARRAY
var arr = [1, 2, 3];
ARRAY is a variable list. ARRAY is also an object.
Read or set the value of an element in ARRAY, using this method:
var val = arr[0];//val为1 arr[2] = 4;//现在arr第三个元素为4
9.1 Array loop (traversal)
for (var i = 0; i < a.length; i++) { // Do something with a[i] } 但是当考虑性能时,则最好只读一次length属性,如下: for (var i = 0, j = a.length; i < j; i++) { // Do something with a[i] } jQuery提供了each方法遍历数组: var x = [1, 2, 3]; $.each(x, function(index, value) { console.log("index", index, "value", value); });
9.2 Calling the push method on an array means adding an element to the end of the array, such as x.push(5); and x.[x.length] = 5; are equivalent
9.3 Other built-in methods of arrays:
var x = [0, 3, 1, 2]; x.reverse() // [2, 1, 3, 0] x.join(" – ") // "2 - 1 - 3 - 0" x.pop() // [2, 1, 3] x.unshift(-1) // [-1, 2, 1, 3] x.shift() // [2, 1, 3] x.sort() // [1, 2, 3] x.splice(1, 2) // 用于插入、删除或替换数组元素,这里为删除从index=1开始的2个元素
9.4 数组为对象,所以始终为true
10. MAP
The map type is used by the AJAX function to hold the data of a request. This type could be a string, an array<form elements>, a jQuery object with form elements or an object with key/value pairs. In the last case, it is possible to assign multiple values to one key by assigning an array. As below: {'key[]':['valuea','valueb']}
11. FUNCTION:匿名和有名两种
11.1 Context、Call和Apply
In JavaScript, the variable "this" always refers to the current context. $(document).ready(function() { // this refers to window.document}); $("a").click(function() { // this refers to an anchor DOM element });
12. SELECTOR
There are lot of plugins that leverage jQuery's selectors in other ways. The validation plugin accepts a selector to specify a dependency, whether an input is required or not:
emailrules: { required: "#email:filled" }
This would make a checkbox with name "emailrules" required only if the user entered an email address in the email field, selected via its id, filtered via a custom selector ":filled" that the validation plugin provides.
13. EVENT
DOM标准事件包括:blur, focus, load, resize, scroll, unload, beforeunload, click, dblclick, mousedown, mouseup, mousemove, mouseover, mouseout, mouseenter, mouseleave, change, select, submit, keydown, keypress, andkeyup
14. JQUERY
JQUERY对象包含DOM元素的集合。比如$('p')即返回所有
...
JQUERY对象行为类似数组,也有length属性,也可以通过index访问DOM元素集合中的某个。但是不是数组,不具备数组的某些方法,比如join()。
许多jQuery方法返回jQuery对象本身,所以可以采用链式调用:
$("p").css("color", "red").find(".special").css("color", "green");
但是如果你调用的方法会破坏jQuery对象,比如find()和filter(),则返回的不是原对象。要返回到原对象只需要再调用end()方法即可。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


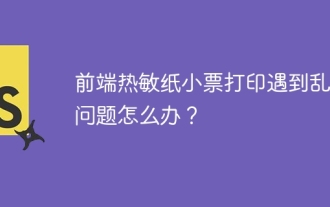
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
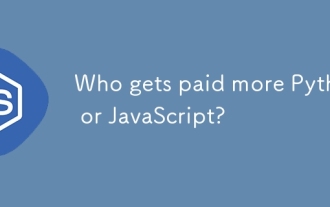
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
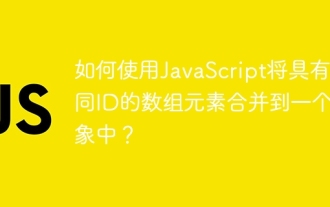
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
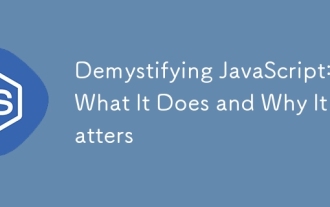
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
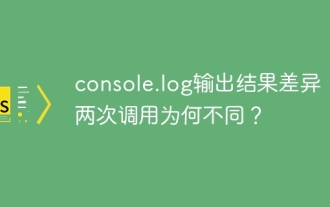
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
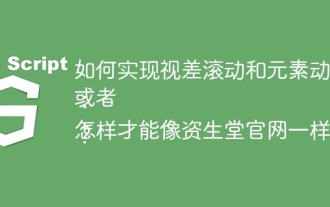
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
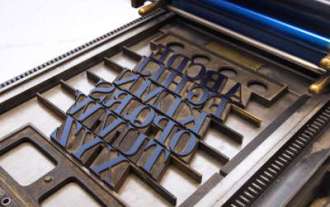
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
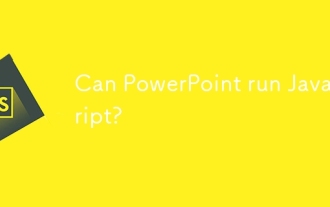
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
