


Quick Fix 6 - PHP: Obtain http request data, obtain get data and post data, and convert between json strings and objects
[Source code download]
Author: webabcd
Introduction
Quick fix PHP
- Get http request data
- Get get data and post data
- Conversion between json strings and objects
Example
1. Get http request data
http/http1.php
<?<span>php </span><span>/*</span><span>* * 获取 http 请求数据 </span><span>*/</span><span>//</span><span> 通过 $_SERVER 获取相关数据</span><span>echo</span> "PHP_SELF : " . <span>$_SERVER</span>['PHP_SELF'] . "<br />"<span>; </span><span>echo</span> "GATEWAY_INTERFACE : " . <span>$_SERVER</span>['GATEWAY_INTERFACE'] . "<br />"<span>; </span><span>echo</span> "SERVER_ADDR : " . <span>$_SERVER</span>['SERVER_ADDR'] . "<br />"<span>; </span><span>echo</span> "SERVER_NAME : " . <span>$_SERVER</span>['SERVER_NAME'] . "<br />"<span>; </span><span>echo</span> "SERVER_SOFTWARE : " . <span>$_SERVER</span>['SERVER_SOFTWARE'] . "<br />"<span>; </span><span>echo</span> "SERVER_PROTOCOL : " . <span>$_SERVER</span>['SERVER_PROTOCOL'] . "<br />"<span>; </span><span>echo</span> "REQUEST_METHOD : " . <span>$_SERVER</span>['REQUEST_METHOD'] . "<br />"<span>; </span><span>echo</span> "REQUEST_TIME : " . <span>$_SERVER</span>['REQUEST_TIME'] . "<br />"<span>; </span><span>echo</span> "REQUEST_TIME_FLOAT : " . <span>$_SERVER</span>['REQUEST_TIME_FLOAT'] . "<br />"<span>; </span><span>echo</span> "QUERY_STRING : " . <span>htmlspecialchars</span>(<span>$_SERVER</span>['QUERY_STRING']) . "<br />"<span>; </span><span>echo</span> "DOCUMENT_ROOT : " . <span>$_SERVER</span>['DOCUMENT_ROOT'] . "<br />"<span>; </span><span>echo</span> "HTTP_ACCEPT : " . <span>$_SERVER</span>['HTTP_ACCEPT'] . "<br />"<span>; </span><span>echo</span> "HTTP_ACCEPT_CHARSET : " . <span>$_SERVER</span>['HTTP_ACCEPT_CHARSET'] . "<br />"<span>; </span><span>echo</span> "HTTP_ACCEPT_ENCODING : " . <span>$_SERVER</span>['HTTP_ACCEPT_ENCODING'] . "<br />"<span>; </span><span>echo</span> "HTTP_ACCEPT_LANGUAGE : " . <span>$_SERVER</span>['HTTP_ACCEPT_LANGUAGE'] . "<br />"<span>; </span><span>echo</span> "HTTP_CONNECTION : " . <span>$_SERVER</span>['HTTP_CONNECTION'] . "<br />"<span>; </span><span>echo</span> "HTTP_HOST : " . <span>$_SERVER</span>['HTTP_HOST'] . "<br />"<span>; </span><span>echo</span> "HTTP_REFERER : " . <span>$_SERVER</span>['HTTP_REFERER'] . "<br />"<span>; </span><span>echo</span> "HTTP_USER_AGENT : " . <span>$_SERVER</span>['HTTP_USER_AGENT'] . "<br />"<span>; </span><span>echo</span> "HTTPS : " . <span>$_SERVER</span>['HTTPS'] . "<br />"<span>; </span><span>echo</span> "REMOTE_ADDR : " . <span>$_SERVER</span>['REMOTE_ADDR'] . "<br />"<span>; </span><span>echo</span> "REMOTE_HOST : " . <span>$_SERVER</span>['REMOTE_HOST'] . "<br />"<span>; </span><span>echo</span> "REMOTE_PORT : " . <span>$_SERVER</span>['REMOTE_PORT'] . "<br />"<span>; </span><span>echo</span> "REMOTE_USER : " . <span>$_SERVER</span>['REMOTE_USER'] . "<br />"<span>; </span><span>echo</span> "REDIRECT_REMOTE_USER : " . <span>$_SERVER</span>['REDIRECT_REMOTE_USER'] . "<br />"<span>; </span><span>echo</span> "SCRIPT_FILENAME : " . <span>$_SERVER</span>['SCRIPT_FILENAME'] . "<br />"<span>; </span><span>echo</span> "SERVER_ADMIN : " . <span>$_SERVER</span>['SERVER_ADMIN'] . "<br />"<span>; </span><span>echo</span> "SERVER_PORT : " . <span>$_SERVER</span>['SERVER_PORT'] . "<br />"<span>; </span><span>echo</span> "SERVER_SIGNATURE : " . <span>$_SERVER</span>['SERVER_SIGNATURE'] . "<br />"<span>; </span><span>echo</span> "PATH_TRANSLATED : " . <span>$_SERVER</span>['PATH_TRANSLATED'] . "<br />"<span>; </span><span>echo</span> "SCRIPT_NAME : " . <span>$_SERVER</span>['SCRIPT_NAME'] . "<br />"<span>; </span><span>echo</span> "REQUEST_URI : " . <span>htmlspecialchars</span>(<span>$_SERVER</span>['REQUEST_URI']) . "<br />"<span>; </span><span>echo</span> "PHP_AUTH_DIGEST : " . <span>$_SERVER</span>['PHP_AUTH_DIGEST'] . "<br />"<span>; </span><span>echo</span> "PHP_AUTH_USER : " . <span>$_SERVER</span>['PHP_AUTH_USER'] . "<br />"<span>; </span><span>echo</span> "PHP_AUTH_PW : " . <span>$_SERVER</span>['PHP_AUTH_PW'] . "<br />"<span>; </span><span>echo</span> "AUTH_TYPE : " . <span>$_SERVER</span>['AUTH_TYPE'] . "<br />"<span>; </span><span>echo</span> "PATH_INFO : " . <span>$_SERVER</span>['PATH_INFO'] . "<br />"<span>; </span><span>echo</span> "ORIG_PATH_INFO : " . <span>$_SERVER</span>['ORIG_PATH_INFO'] . "<br />";
2. Get get data and post data
http/http2.php
<?<span>php </span><span>/*</span><span>* * 获取 get 数据 和 post 数据 </span><span>*/</span><span>//</span><span> 获取 url 参数(get 数据)</span><span>$params</span> = getQueryParams(<span>$_SERVER</span>['QUERY_STRING'<span>]); </span><span>echo</span> "param1: " . <span>$params</span>["param1"<span>]; </span><span>echo</span> "<br />"<span>; </span><span>echo</span> "param2: " . <span>$params</span>["param2"<span>]; </span><span>echo</span> "<br />"<span>; </span><span>function</span> getQueryParams(<span>$query</span><span>) { </span><span>$queryParts</span> = <span>explode</span>('&', <span>$query</span><span>); </span><span>$params</span> = <span>array</span><span>(); </span><span>foreach</span> (<span>$queryParts</span><span>as</span><span>$param</span><span>) { </span><span>$item</span> = <span>explode</span>('=', <span>$param</span><span>); </span><span>$params</span>[<span>$item</span>[0]] = <span>$item</span>[1<span>]; } </span><span>return</span><span>$params</span><span>; } </span><span>//</span><span> 通过 $_POST 获取 post 数据 // 判断 $_POST["btnSubmit"] 是否存在,如果存在则表示有 post 过来的 form</span><span>if</span> (<span>isset</span>(<span>$_POST</span>["btnSubmit"<span>])) { </span><span>if</span> (<span>empty</span>(<span>$_POST</span>['txtUsername'<span>])) { </span><span>echo</span> "您没有输入用户名"<span>; </span><span>exit</span>(0<span>); } </span><span>echo</span> "您的用户名: " . <span>$_POST</span>['txtUsername'] . ""<span>; } </span>?> <form action="" method="post"> <div>姓名:</div> <div><input type="text" name="txtUsername" value="wanglei" /></div> <div><input type="submit" name="btnSubmit" value="提交"/></div> </form>
3. Get get data and post data
encode/json.php
<?<span>php </span><span>/*</span><span>* * Created by PhpStorm. * User: wanglei * Date: 2015/12/30 * Time: 9:45 </span><span>*/</span><span>class</span><span> Name { </span><span>public</span><span>$firstName</span><span>; </span><span>public</span><span>$lastName</span><span>; } </span><span>class</span><span> Student { </span><span>public</span><span>$name</span><span>; </span><span>public</span><span>$number</span><span>; </span><span>public</span><span>$date1</span><span>; </span><span>public</span><span>$date2</span><span>; } </span><span>$jsonArray</span> = '<span>[ { "name":{"firstName":"abc", "lastName":"xyz"}, "number":1, "date1":"2015-12-30 10:00:48", "date2":1451440848 }, { "name":{"firstName":"lmn", "lastName":"rst"}, "number":2, "date1":"2015-11-22 17:13:41", "date2":1448183621 } ]</span>'<span>; </span><span>//</span><span> 设置当前时区为东八时区(北京时区)</span>date_default_timezone_set("Etc/GMT-8"<span>); </span><span>//</span><span> json 字符串转对象</span><span>$result</span> = <span>array</span><span>(); </span><span>$myArray</span> = json_decode(<span>$jsonArray</span>, <span>true</span><span>); </span><span>$myArrayCount</span> = <span>count</span>(<span>$myArray</span><span>); </span><span>for</span> (<span>$i</span> = 0; <span>$i</span> < <span>$myArrayCount</span>; <span>$i</span>++<span>) { </span><span>$student</span> = <span>new</span><span> Student(); </span><span>$student</span>-><span>number</span> = <span>$myArray</span>[<span>$i</span>]["number"<span>]; </span><span>$student</span>->date1 = <span>$myArray</span>[<span>$i</span>]["date1"<span>]; </span><span>$student</span>->date2 = <span>date</span>('Y-m-d H:i:s', <span>$myArray</span>[<span>$i</span>]["date2"<span>]); </span><span>$name</span> = <span>new</span><span> Name(); </span><span>$jsonName</span> = <span>$myArray</span>[<span>$i</span>]["name"<span>]; </span><span>$name</span>->firstName = <span>$jsonName</span>["firstName"<span>]; </span><span>$name</span>->lastName = <span>$jsonName</span>["lastName"<span>]; </span><span>$student</span>->name = <span>$name</span><span>; </span><span>$result</span>[] = <span>$student</span><span>; } </span><span>var_dump</span>(<span>$result</span><span>); </span><span>//</span><span> 对象转 json 字符串</span><span>echo</span> json_encode(<span>$result</span><span>); </span><span>echo</span> "<br />"<span>; </span><span>echo</span> "<br />"<span>; </span><span>//</span><span> 获取当前时间的时间戳(到 1970-1-1 的秒数)</span><span>echo</span><span>time</span><span>(); </span><span>echo</span> "<br />"<span>; </span><span>//</span><span> 获取指定时间的时间戳(到 1970-1-1 的秒数)</span><span>echo</span><span>strtotime</span>("2015-12-30 16:00:10"<span>); </span><span>echo</span> "<br />"<span>; </span><span>//</span><span> 时间戳的格式化</span><span>echo</span><span>date</span>('Y-m-d H:i:s', <span>time</span><span>()); </span><span>echo</span> "<br />"<span>; </span><span>//</span><span> 时间戳的格式化</span><span>echo</span><span>date</span>('Y-m-d H:i:s', 1460969676<span>); </span><span>echo</span> "<br />";
OK
[Source code download]
The above introduces Quick Solution 6 - PHP: Obtain http request data, obtain get data and post data, and convert between json strings and objects, including aspects of the content. I hope friends who are interested in PHP tutorials can learn from it. help.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


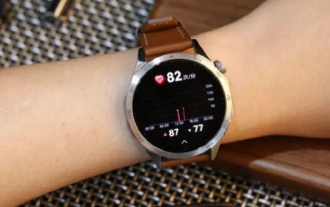
Many users will choose the Huawei brand when choosing smart watches. Among them, Huawei GT3pro and GT4 are very popular choices. Many users are curious about the difference between Huawei GT3pro and GT4. Let’s introduce the two to you. . What are the differences between Huawei GT3pro and GT4? 1. Appearance GT4: 46mm and 41mm, the material is glass mirror + stainless steel body + high-resolution fiber back shell. GT3pro: 46.6mm and 42.9mm, the material is sapphire glass + titanium body/ceramic body + ceramic back shell 2. Healthy GT4: Using the latest Huawei Truseen5.5+ algorithm, the results will be more accurate. GT3pro: Added ECG electrocardiogram and blood vessel and safety
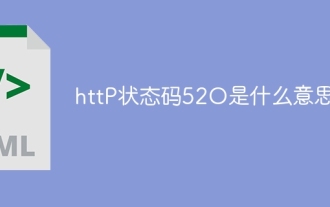
HTTP status code 520 means that the server encountered an unknown error while processing the request and cannot provide more specific information. Used to indicate that an unknown error occurred when the server was processing the request, which may be caused by server configuration problems, network problems, or other unknown reasons. This is usually caused by server configuration issues, network issues, server overload, or coding errors. If you encounter a status code 520 error, it is best to contact the website administrator or technical support team for more information and assistance.
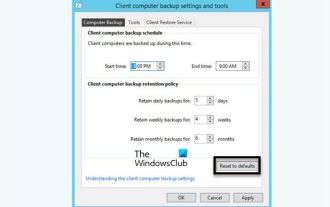
WindowsServerBackup is a function that comes with the WindowsServer operating system, designed to help users protect important data and system configurations, and provide complete backup and recovery solutions for small, medium and enterprise-level enterprises. Only users running Server2022 and higher can use this feature. In this article, we will explain how to install, uninstall or reset WindowsServerBackup. How to Reset Windows Server Backup If you are experiencing problems with your server backup, the backup is taking too long, or you are unable to access stored files, then you may consider resetting your Windows Server backup settings. To reset Windows
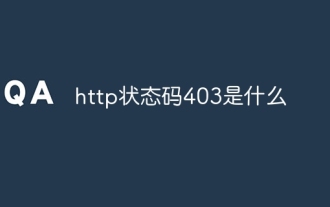
HTTP status code 403 means that the server rejected the client's request. The solution to http status code 403 is: 1. Check the authentication credentials. If the server requires authentication, ensure that the correct credentials are provided; 2. Check the IP address restrictions. If the server has restricted the IP address, ensure that the client's IP address is restricted. Whitelisted or not blacklisted; 3. Check the file permission settings. If the 403 status code is related to the permission settings of the file or directory, ensure that the client has sufficient permissions to access these files or directories, etc.
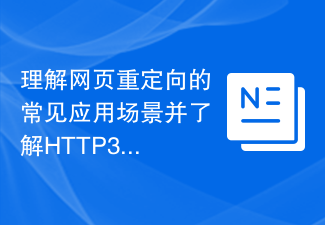
Understand the meaning of HTTP 301 status code: common application scenarios of web page redirection. With the rapid development of the Internet, people's requirements for web page interaction are becoming higher and higher. In the field of web design, web page redirection is a common and important technology, implemented through the HTTP 301 status code. This article will explore the meaning of HTTP 301 status code and common application scenarios in web page redirection. HTTP301 status code refers to permanent redirect (PermanentRedirect). When the server receives the client's

In today's era of rapid technological development, programming languages are springing up like mushrooms after a rain. One of the languages that has attracted much attention is the Go language, which is loved by many developers for its simplicity, efficiency, concurrency safety and other features. The Go language is known for its strong ecosystem with many excellent open source projects. This article will introduce five selected Go language open source projects and lead readers to explore the world of Go language open source projects. KubernetesKubernetes is an open source container orchestration engine for automated
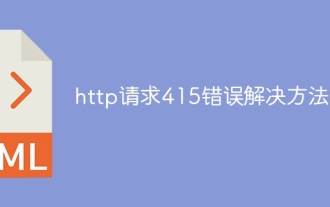
Solution: 1. Check the Content-Type in the request header; 2. Check the data format in the request body; 3. Use the appropriate encoding format; 4. Use the appropriate request method; 5. Check the server-side support.
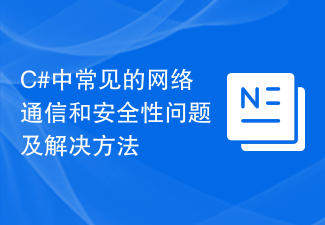
Common network communication and security problems and solutions in C# In today's Internet era, network communication has become an indispensable part of software development. In C#, we usually encounter some network communication problems, such as data transmission security, network connection stability, etc. This article will discuss in detail common network communication and security issues in C# and provide corresponding solutions and code examples. 1. Network communication problems Network connection interruption: During the network communication process, the network connection may be interrupted, which may cause
