php设计模式之单例模式使用示例_php实例
以下为单例模式代码:
class EasyFramework_Easy_Mysql{
protected static $_instance = null;
private function __construct(){
}
public static function getInstance(){
if (self::$_instance === null){
self::$_instance = new self();
}
return self::$_instance;
}
protected function __clone(){
}
}
$x = EasyFramework_Easy_Mysql::getInstance();
var_dump($x);
?>
/*
* 1.第一步:
* 既然是单例,也就是只能实例化一次,也就代表在实例化时
* 不可能使用new关键字!!!!
* 在使用new关键字时,类中的构造函数将自动调用。
* 但是,如果我们将构造函数的访问控制符设置为protected或private
* 那么就不可能直接使用new关键字了!!!
* 第二步:
* 无论protected/private修饰的属性或方法,请问在当前类的
* 内部是否可以访问?---> 可以
* 第三步:
* 现在我们根本没有办法得到对象(因为你不能使用new关键字了),
* 第四步:静态成员(包括属性或方法)在访问时,只能通过
* 类名称::属性()
* 类名称::方法()
* 第五步:如果我现在存在一个静态方法--> getInstance()
* 那么在调用时就应写成
* $object = EasyFramework_Easy_Mysql::getInstance()
* 如果,getInstance()方法可以得到唯一的一个对象
* 也就代表是所谓的单例模式了!!!
* 第六步,怎么让getInstace()只得到一个对象呢?
* 既然要得到对象,那么肯定是:
* $variabl = new ????();
* 我们又知道静态属性的值是可以所有的对象来继承的!!!
* 静态成员是属于类的,而非对象的!
* 所以:
* 第七步:声明一个静态的属性,用其存储实例化的对象
* protectd static $_instance
*
* 并且初始值为null
* 那么我在调用getInstance()方法时,只需要判断其值是否为空即可
*
* public static function getInstance(){
* if(self::_instance === null){
* self::_instance = new self();
* }
* return self::_instance;
* }
* 在实例时,一定是这样写:
* $x = EasyFramework_Easy_Mysql::getInstance();
* 在第一时调用时,类的$_instance这个静态属性值为null,
* 那么也就代表,getInstance()方法的判断条件为真了,
* 也就意味着
* self::$_instance这个成员有了值了!!!
* 并且还返回这个值
* $y = EasyFramework_Easy_Mysql::getInstance();
* 在第二次或第N次调用时,self::$_instance已经有了值了
* 也就代表getInstance()方法的条件为假了!!!
* 也就代表其中的程序代表不可能执行了!!!
* 也就代表将直接返回以前的值了!!!
*
*
*
* */

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
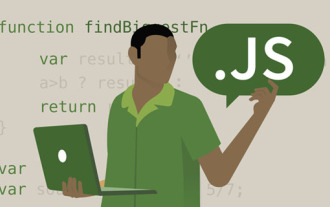
The JS singleton pattern is a commonly used design pattern that ensures that a class has only one instance. This mode is mainly used to manage global variables to avoid naming conflicts and repeated loading. It can also reduce memory usage and improve code maintainability and scalability.
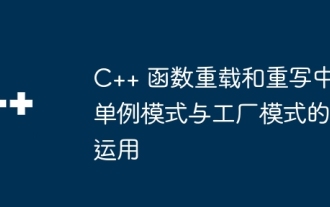
Singleton pattern: Provide singleton instances with different parameters through function overloading. Factory pattern: Create different types of objects through function rewriting to decouple the creation process from specific product classes.
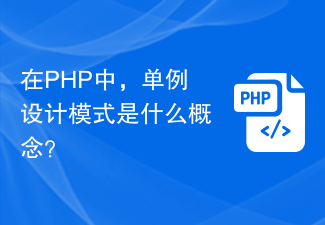
The Singleton pattern ensures that a class has only one instance and provides a global access point. It ensures that only one object is available and under control in the application. The Singleton pattern provides a way to access its unique object directly without instantiating the object of the class. Example<?php classdatabase{ publicstatic$connection; privatefunc
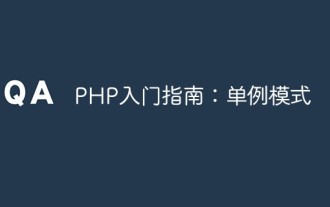
In software development, we often encounter situations where multiple objects need to access the same resource. In order to avoid resource conflicts and improve program efficiency, we can use design patterns. Among them, the singleton pattern is a commonly used way to create objects, which ensures that a class has only one instance and provides global access. This article will introduce how to use PHP to implement the singleton pattern and provide some best practice suggestions. 1. What is the singleton mode? The singleton mode is a commonly used way to create objects. Its characteristic is to ensure that a class has only one instance and provides
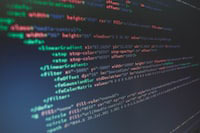
Introduction PHP design patterns are a set of proven solutions to common challenges in software development. By following these patterns, developers can create elegant, robust, and maintainable code. They help developers follow SOLID principles (single responsibility, open-closed, Liskov replacement, interface isolation and dependency inversion), thereby improving code readability, maintainability and scalability. Types of Design Patterns There are many different design patterns, each with its own unique purpose and advantages. Here are some of the most commonly used PHP design patterns: Singleton pattern: Ensures that a class has only one instance and provides a way to access this instance globally. Factory Pattern: Creates an object without specifying its exact class. It allows developers to conditionally
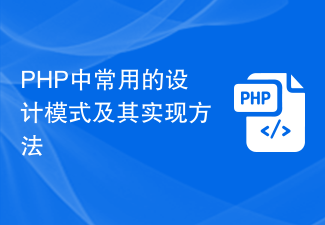
PHP is a widely used and very popular programming language. PHP is a very important part of today's web applications. Design patterns play a vital role in developing PHP applications. Design pattern is a template for solving problems that can be reused in different environments. It helps us write better code and make the code more reliable, maintainable and scalable. In this article, we will explore some commonly used design patterns in PHP and how to implement them. Singleton Pattern Singleton pattern is a creation pattern that allows
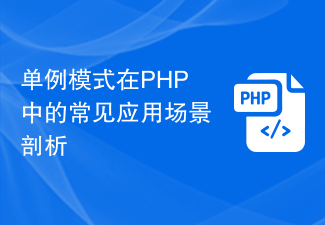
Overview of the analysis of common application scenarios of the singleton pattern in PHP: The singleton pattern (SingletonPattern) is a creational design pattern that ensures that a class has only one instance and provides a global access point to access the instance. In PHP, using the singleton mode can effectively limit the number of instantiations and resource usage of a class, and improve the performance and maintainability of the code. This article will analyze common application scenarios and give specific PHP code examples to illustrate the use and benefits of the singleton pattern. Database connection pipe
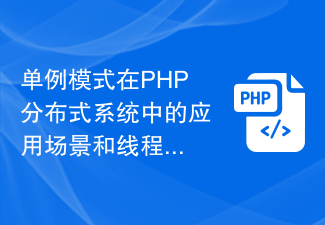
Application scenarios and thread safety processes of singleton mode in PHP distributed systems Introduction: With the rapid development of the Internet, distributed systems have become a hot topic in modern software development. In distributed systems, thread safety has always been an important issue. In PHP development, the singleton pattern is a commonly used design pattern, which can effectively solve the problems of resource sharing and thread safety. This article will focus on the application scenarios and thread safety processes of the singleton pattern in PHP distributed systems, and provide specific code examples. 1. Singleton mode
