


Basic data types and packaging types of JavaScript type system_javascript skills
Written in front
JavaScript data types can be divided into two types: primitive types and reference types
Primitive types are also called basic types or simple types because they occupy a fixed space and are simple data segments. In order to improve the speed of variable query, they are stored in the stack (accessed by value). Among them, the basic data types of JavaScript include Undefined, Null, Boolean, Number and String
Since the size of the value of a reference type will change, it cannot be stored on the stack, otherwise it will reduce the speed of variable query, so it is stored in the heap (heap). The value stored in the variable is a pointer pointing to The memory location where the object is stored (access by address)
[Note] For reference type values, you can add attributes and methods to them, and you can also change and delete their attributes and methods; but basic types cannot add attributes and methods
Undefined
The Undefined type has only one value, which is undefined. When a declared variable is not initialized, the default value of the variable is undefined
var test;//undefined console.log(test == undefined);//true var test = undefined;//undefined
Only one operation can be performed on a variable that has not been declared yet. Use the typeof operator to detect its data type, but in strict mode it will cause an error
typeof(test);//undefined
Scene appears
[1]Declared unassigned variable
[2] Get the non-existent attributes of the object
[3]Execution results of functions without return values
[4]The parameters of the function are not passed in
[5]void(expression)
Type conversion
Boolean(undefined): false Number(undefined): NaN String(undefined): 'undefined'
Null
The Null type has only one value, which is null. From a logical point of view, the null value represents a null object pointer. If the defined variable will be used to save the object, it is best to initialize the variable to null. In fact, the undefined value is derived from the null value, so undefined == null
[Note] null is a null object pointer, [] is an empty array, {} is an empty object, the three are different
console.log(null == undefined);//true
Scene appears
When the object does not exist
Type conversion
Boolean(null): false
Number(null): 0
String(null): 'null'
[Note] Because undefined and null are not constructor types, you cannot add custom properties
Packaging Type
Wrapper types are special reference types. Whenever a basic type value is read, an object of the corresponding basic wrapper type is created in the background, and some methods may be called to manipulate the data. There are three packaging types: Boolean, Number and String
var s1 = 'some text'; var s2 = s1.substring(2); //在上述过程中,实际上发生了三个步骤 var s1 = new String('some text'); //(1)创建String类型的一个实例 var s2 = s1.substring(2); //(2)在实例上调用指定的方法 s1 = null; //(3)销毁这个实例
[Note] The main difference between reference types and basic wrapper types is the lifetime of the object. Instances of reference types created using the new operator remain in memory until the execution flow leaves the current scope. The automatically created basic packaging type object only exists at the moment when a line of code is executed, and then is destroyed immediately. This means that properties and methods cannot be added at runtime for primitive type values
var s1 = 'some text'; s1.color = 'red'; alert(s1.color);//undefined
Creation method
There are two ways to explicitly create a packaging type:
[1] Object method [not recommended]
var s = new Object('abc'); var b = new Object(true); var n = new Object(123);
[2] Constructor method [not recommended]
var s = new String('abc'); var b = new Boolean(true); var n = new Number(123);
[Note] Using new to call the constructor of a basic packaging type is different from directly calling the transformation function of the same name
var value = '25'; var number = Number(value); console.log(typeof number);//number var obj = new Number(value); console.log(typeof obj);//object
Boolean
Boolean类型只有两个值:true 和 false。Boolean包装类型是与布尔值对应的引用类型,在布尔表达式中使用Boolean对象容易造成误解
出现场景
[1]条件语句导致系统执行的隐士类型转换
[2]字面量或变量定义
类型转换
Number(true): 1 || Number(false) : 0
String(true):'true' || String(false):'false'
Boolean()
Boolean(undefined):false
Boolean(null):false
Boolean(非空对象包括空数组[]和空对象{}):true
Boolean(非0): true || Boolean(0和NaN):false
Boolean(非空包括空格字符串):true || Boolean(''):false
[注意]true不一定等于1,false也不一定等于0
包装类型继承的方法
valueOf():返回基本类型值true 或 false
toString()和toLocaleString():返回字符串'true' 或'false'
console.log(typeof true.valueOf(),true.valueOf());//boolean true console.log(typeof false.valueOf(),false.valueOf());//boolean false console.log(typeof true.toString(),true.toString());//String 'true' console.log(typeof false.toString(),false.toString());//String 'false' console.log(typeof true.toLocaleString(),true.toLocaleString());//String 'true' console.log(typeof false.toLocaleString(),false.toLocaleString());//String 'false'
Number
javascript只有一种数字类型,既可以表示32位的整数,还可以表示64位的浮点数
关于Number类型的详细信息移步到此
String
String类型是javascript中唯一没有固定大小的原始类型
下面接着来理解下JavaScript基本数据类型的包装对象
现象:为什么可以对字符串的操作采用对象的表示法?
例如:
var s = "this is a String"; var len = s.length;
解析:
JavaScript三个基本数据类型都有相应的对象类;分别为Sring,Number,Boolean类;
JavaScript可以灵活的将一种类型的值转换为另一种类型;
当我们在对象环境中使用字符串时,即当我们试图访问这个字符串的属性或方法时;
JavaScript会为这个字符串值内部地创建一个String包装对象;
String对象会暂时代替原始的字符串值,完成我们的访问;
这个被内部创建的String对象是瞬间存在的,它的作用是使我们可以正常访问属性和方法;
String对象在使用过后会被系统丢弃掉;
而原始值并不会被改变;
以上同样适用于数字和布尔值类型;
使用Object()函数,任何数字、字符串、布尔值都可以转换为它对应的包装对象;
例如:
var number_wrapper = Object (3);

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


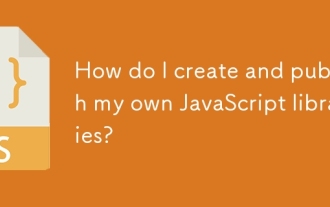
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
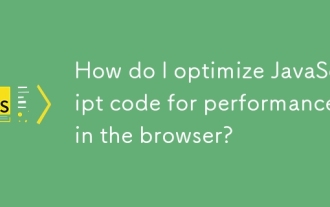
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
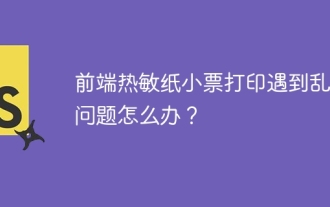
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
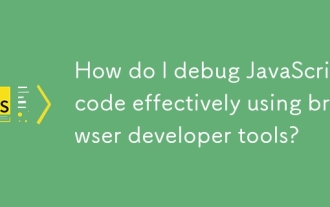
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
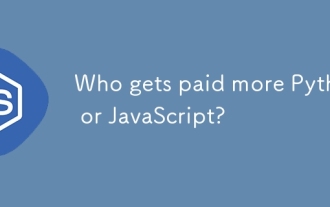
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
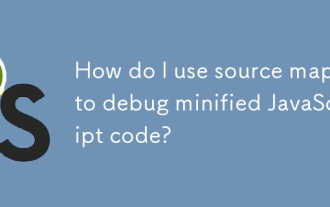
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
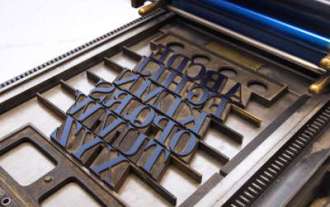
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
