PHP Object-Oriented Programming - Special Practice DAY 4
(only available in PHP, other languages are not Object-oriented)
Object-oriented--magic methods __construct(), __destruct() constructors and destructors
__tostring()
__invoke()
__call(), __callStatic()
__get(), __set(), __isset(), __unset()
__clone()
__tostring()
When the
object is used as String, this method will be called automatically. Echo $obj;
__invoke()
When the
object is called as a method, this method will be automatically called
$obj(4);
<?php class MagicTest{ //__tostring会在把<strong>对象</strong>转换为string的时候自动调用 public function __tostring(){ return "This is the Class MagicTest. "; } // __invoke会在把<strong>对象</strong>当作一个方法调用的时候自动调用 public function __invoke($x){ echo "__invoke called with parameter ".$x."\n"; } } $obj = new MagicTest(); echo $obj."\n"; $obj(5); ?>
This is the Class MagicTest.
__invoke called with parameter 5
When the
object accesses a non-existing method name, the __call() method will be automatically called __callStatic()
When the
object accesses a non-existing static method name When Can be implemented in different ways
<?php class MagicTest{ public function __tostring(){ return "This is the Class MagicTest. "; } public function __invoke($x){ echo "__invoke called with parameter ".$x."\n"; } //方法的重载 //这个方法的参数第一个就是调用的方法的名称,第二个参数是方法调用的参数组成的数组 public function __call($name,$arguments){ echo "Calling " . $name . " with parameters: ". implode(",", $arguments)."\n"; } //<strong>静态方法</strong>的重载,注意这个方法需要设定为static public static function __callStatic($name,$arguments){ echo "Static Calling " . $name . " with parameters: ". implode(",", $arguments)."\n"; } } $obj = new MagicTest(); //runTest这两个方法的名称本来不能完全相同,但是通过魔术方法,可以调用了 $obj->runTest("para1","para2"); //没有声明这个runTest方法,因为有__call这个魔术方法,也可以被调用 MagicTest::runTest("para1","para2"); //没有声明这个runTest方法,因为有__callStatic这个魔术方法,也可以被调用 ?>
Output:
Calling runTest with parameters: para1, para2
Static Calling runTest with parameters: para1, para2
__get(), __set(), __isset(), __unset( )
When assigning a value to an inaccessible property, __set() will be called
When calling isset() or empty() on an inaccessible property, __isset( ) will be called
When unset() is called on an inaccessible attribute, __unset() will be called
The so-called inaccessible attribute actually means that when calling a certain attribute, it is found that the attribute is not defined. At this time, different operations will Trigger different magic methods
These methods are also called magic methods of property overloading
<?php class MagicTest{ public function __tostring(){ return "This is the Class MagicTest. "; } public function __invoke($x){ echo "__invoke called with parameter ".$x."\n"; } //方法的重载 //这个方法的参数第一个就是调用的方法的名称,第二个参数是方法调用的参数组成的数组 public function __call($name,$arguments){ echo "Calling " . $name . "with parameters: ". implode(",", $arguments)."\n"; } //<strong>静态方法</strong>的重载,注意这个方法需要设定为static public static function __callStatic($name,$arguments){ echo "Static Calling " . $name . "with parameters: ". implode(",", $arguments)."\n"; } //属性重载 public function __get($name){ return "Getting the property ".$name."\n"; } public function __set($name, $value){ echo "Setting the property ".$name." to value " . $value."\n"; } public function __isset($name){ echo "__isset invoked\n"; return true; } public function __unset($name){ echo "unsetting property ".$name."\n"; } } $obj = new MagicTest(); echo $obj->className."\n"; //className未定义,但是通过魔术方法__get,这个方法好像被定义了一样 $obj->className='MagicClassX'; //通过魔术方法__get将className名称定义为MagicClassX echo '$obj->name is set?'.isset($obj->className)."\n"; echo '$obj->name is empty?'.empty($obj->className)."\n"; unset($obj->className); ?>
Output:Getting the property className
Setting the property className to value MagicClassX
__isset invoked
$obj-> name is set?1 //If return is true, the result is displayed as 1; if return is false, the result is displayed as empty
__isset invoked
$obj->name is empty? //If return is true, the result is displayed Displayed as empty; if return is false, the result is displayed as 1
unsetting property className
oriented
object
--magic method

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Function means function. It is a reusable code block with specific functions. It is one of the basic components of a program. It can accept input parameters, perform specific operations, and return results. Its purpose is to encapsulate a reusable block of code. code to improve code reusability and maintainability.
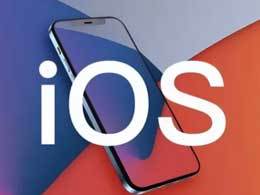
Every year before Apple releases a new major version of iOS and macOS, users can download the beta version several months in advance and experience it first. Since the software is used by both the public and developers, Apple has launched developer and public versions, which are public beta versions of the developer beta version, for both. What is the difference between the developer version and the public version of iOS? Literally speaking, the developer version is a developer test version, and the public version is a public test version. The developer version and the public version target different audiences. The developer version is used by Apple for testing by developers. You need an Apple developer account to download and upgrade it.
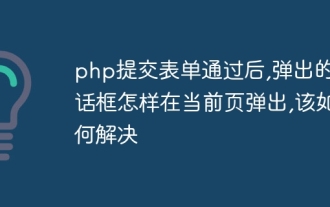
php提交表单通过后,弹出的对话框怎样在当前页弹出php提交表单通过后,弹出的对话框怎样在当前页弹出而不是在空白页弹出?想实现这样的效果:而不是空白页弹出:------解决方案--------------------如果你的验证用PHP在后端,那么就用Ajax;仅供参考:HTML code
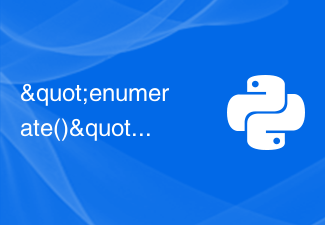
In this article, we will learn about enumerate() function and the purpose of “enumerate()” function in Python. What is the enumerate() function? Python's enumerate() function accepts a data collection as a parameter and returns an enumeration object. Enumeration objects are returned as key-value pairs. The key is the index corresponding to each item, and the value is the items. Syntax enumerate(iterable,start) Parameters iterable - The passed in data collection can be returned as an enumeration object, called iterablestart - As the name suggests, the starting index of the enumeration object is defined by start. if we ignore
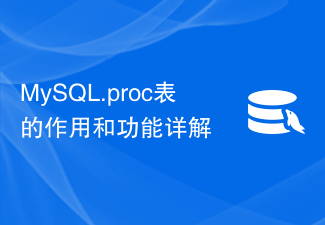
Detailed explanation of the role and function of the MySQL.proc table. MySQL is a popular relational database management system. When developers use MySQL, they often involve the creation and management of stored procedures (StoredProcedure). The MySQL.proc table is a very important system table. It stores information related to all stored procedures in the database, including the name, definition, parameters, etc. of the stored procedures. In this article, we will explain in detail the role and functionality of the MySQL.proc table
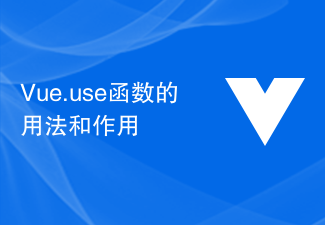
Usage and Function of Vue.use Function Vue is a popular front-end framework that provides many useful features and functions. One of them is the Vue.use function, which allows us to use plugins in Vue applications. This article will introduce the usage and function of the Vue.use function and provide some code examples. The basic usage of the Vue.use function is very simple, just call it before Vue is instantiated, passing in the plugin you want to use as a parameter. Here is a simple example: //Introduce and use the plug-in
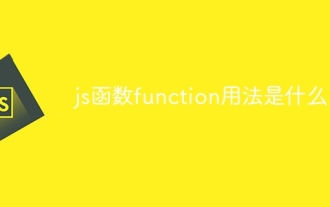
The usage of js function function is: 1. Declare function; 2. Call function; 3. Function parameters; 4. Function return value; 5. Anonymous function; 6. Function as parameter; 7. Function scope; 8. Recursive function.

The file_exists method checks whether a file or directory exists. It accepts as argument the path of the file or directory to be checked. Here's what it's used for - it's useful when you need to know if a file exists before processing it. This way, when creating a new file, you can use this function to know if the file already exists. Syntax file_exists($file_path) Parameters file_path - Set the path of the file or directory to be checked for existence. Required. Return file_exists() method returns. Returns TrueFalse if the file or directory exists, if the file or directory does not exist Example let us see a check for "candidate.txt" file and even if the file
