


[Modern PHP] Chapter 2 New Features 2 Interface-based Programming
Interface-based programming
As a PHP programmer, learning how to program based on interfaces has changed my life and greatly improved my ability to improve my projects by integrating third-party PHP components. Interfaces are not a new feature, but they are important features that you must understand and use in your daily work.
So what exactly is the interface of PHP? An interface is a contract between two PHP objects. When one object calls another object, it does not need to know what the other party is, but only needs to know what the other party can do. Interfaces can reduce the coupling of our code's dependencies, allowing our code to call any third-party code that implements the desired interface. We only need to care about whether the third-party code implements the interface, and we don't need to care at all about how the third-party code implements these interfaces. Let's look at a real-life example.
Suppose I go to Miami, Florida to attend the Sunshine PHP Developer Conference. I wanted to explore the city so I went straight to the local car rental agency. They had a modern compact car, a Subaru station wagon and a Bugatti Veyron (to my surprise). I knew I just wanted some form of transportation to get around town, and all three of these vehicles would fit my needs. But each car is so different. The Hyundai Accent is not bad, but I like something a little more dynamic. I don’t have kids, so the station wagon is still a bit big. So, okay, just choose Bugatti.
The reality is that I can drive any of these three cars because they all share a common, known interface. Every car has a steering wheel, a gas pedal, a brake pedal and turn signals, and every car uses gasoline as fuel. But the Bugatti's power is so powerful that I can't handle it, but the driving interface of the modern car is exactly the same. Because all three cars share the same known interface, I have the opportunity to choose the model I like better (to be honest, I will probably choose Hyundai in the end).
The same concept exists in the object-oriented aspect of PHP. If my code uses objects of a specific class (representing a specific implementation), then the functionality of my code becomes very limited, because it can only use objects of that class forever. However, if my code is going to use an interface, my code will immediately know how to use any object that implements the interface. My code doesn't care at all about how the interface is implemented. My code only cares about whether the object implements the interface. Let's use an example to explain it all.
Suppose we have a PHP class named DocumentStore that can get data from different data sources. It can get HTML from a remote address, read data from the document stream, and get terminal commands. line output. Each document saved in a DocumentStore instance has a unique ID. Example 2-6 shows the DocumentStore class.
Example 2-6 Definition of DocumentStore class
class DocumentStore { protected $data = []; public function addDocument(Documentable $document) { $key = $document->getId(); $value = $document->getContent(); $this->data[$key] = $value; } public function getDocuments() { return $this->data; } }
If the addDocument method only receives instances of the Documentable class as parameters, how can we achieve the function described above? Very good at observation. In fact, Documentable is not a class, but an interface. Take a look at the definition of the interface in Example 2-7
Example 2-7 The definition of Documentable interface
interface Documentable { public function getId(); public function getContent(); }
In the definition of the interface we can see any implementation Objects of the Documentable interface must define a public getId() method and a public getContent() method.
So what are the benefits of doing this? The advantage is that we can construct multiple document acquisition classes with different functions. Example 2-8 shows an interface implementation that obtains HTML from a remote address through curl.
Example 2-8 Definition of HtmlDocument class
<?php class HtmlDocument implements Documentable { protected $url; public function __construct($url) { $this->url = $url; } public function getId() { return $this->url; } public function getContent() { $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $this->url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 3); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($ch, CURLOPT_MAXREDIRS, 3); $html = curl_exec($ch); curl_close($ch); return $html; } }
Another implementation (Example 2-9) can read the data stream from a given resource.
Example 2-9 Definition of StreamDocument class
<?php class StreamDocument implements Documentable { protected $resource; protected $buffer; public function __construct($resource, $buffer = 4096) { $this->resource = $resource; $this->buffer = $buffer; } public function getId() { return 'resource-' . (int)$this->resource; } public function getContent() { $streamContent = ''; rewind($this->resource); while (feof($this->resource) === false) { $streamContent .= fread($this->resource, $this->buffer); } return $streamContent; } }
The last implementation (Example 2-10) can execute a terminal command and obtain the execution results
Example 2-10 Definition of CommandOutputDocument class
<?php class CommandOutputDocument implements Documentable { protected $command; public function __construct($command) { $this->command = $command; } public function getId() { return $this->command; } public function getContent() { return shell_exec($this->command); } }
Example 2-11 shows how we use the DocumentStore class to operate the three document collection classes that implement the Documentable interface
Example 2-11 DocumentStore
<?php $documentStore = new DocumentStore(); // Add HTML document $htmlDoc = new HtmlDocument('http://php.net'); $documentStore->addDocument($htmlDoc); // Add stream document $streamDoc = new StreamDocument(fopen('stream.txt', 'rb')); $documentStore->addDocument($streamDoc); // Add terminal command document $cmdDoc = new CommandOutputDocument('cat /etc/hosts'); $documentStore->addDocument($cmdDoc); print_r($documentStore->getDocuments());
The biggest highlight of this is the HtmlDocument, StreamDocument and CommandOutputDocument classes Except for the consistent interface, other aspects are completely different.
Today, programming interfaces creates more flexible code, and we no longer care about the specific implementation, but leave these tasks to others. More and more people (such as your colleagues, users of your open source projects, or developers you have never met) can write code that works seamlessly with you, and they just need to understand Just interface.
The above introduces [Modern PHP] Chapter 2 New Features 2 Interface-based Programming, including aspects of content. I hope it will be helpful to friends who are interested in PHP tutorials.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Function means function. It is a reusable code block with specific functions. It is one of the basic components of a program. It can accept input parameters, perform specific operations, and return results. Its purpose is to encapsulate a reusable block of code. code to improve code reusability and maintainability.
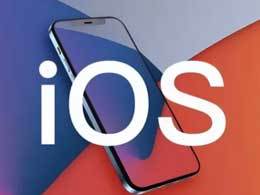
Every year before Apple releases a new major version of iOS and macOS, users can download the beta version several months in advance and experience it first. Since the software is used by both the public and developers, Apple has launched developer and public versions, which are public beta versions of the developer beta version, for both. What is the difference between the developer version and the public version of iOS? Literally speaking, the developer version is a developer test version, and the public version is a public test version. The developer version and the public version target different audiences. The developer version is used by Apple for testing by developers. You need an Apple developer account to download and upgrade it.
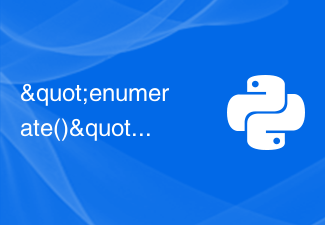
In this article, we will learn about enumerate() function and the purpose of “enumerate()” function in Python. What is the enumerate() function? Python's enumerate() function accepts a data collection as a parameter and returns an enumeration object. Enumeration objects are returned as key-value pairs. The key is the index corresponding to each item, and the value is the items. Syntax enumerate(iterable,start) Parameters iterable - The passed in data collection can be returned as an enumeration object, called iterablestart - As the name suggests, the starting index of the enumeration object is defined by start. if we ignore
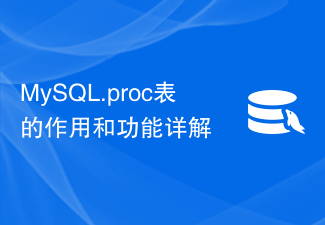
Detailed explanation of the role and function of the MySQL.proc table. MySQL is a popular relational database management system. When developers use MySQL, they often involve the creation and management of stored procedures (StoredProcedure). The MySQL.proc table is a very important system table. It stores information related to all stored procedures in the database, including the name, definition, parameters, etc. of the stored procedures. In this article, we will explain in detail the role and functionality of the MySQL.proc table

The file_exists method checks whether a file or directory exists. It accepts as argument the path of the file or directory to be checked. Here's what it's used for - it's useful when you need to know if a file exists before processing it. This way, when creating a new file, you can use this function to know if the file already exists. Syntax file_exists($file_path) Parameters file_path - Set the path of the file or directory to be checked for existence. Required. Return file_exists() method returns. Returns TrueFalse if the file or directory exists, if the file or directory does not exist Example let us see a check for "candidate.txt" file and even if the file
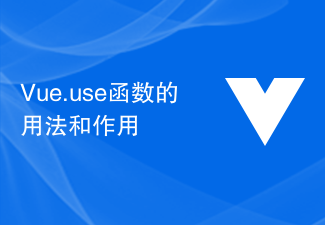
Usage and Function of Vue.use Function Vue is a popular front-end framework that provides many useful features and functions. One of them is the Vue.use function, which allows us to use plugins in Vue applications. This article will introduce the usage and function of the Vue.use function and provide some code examples. The basic usage of the Vue.use function is very simple, just call it before Vue is instantiated, passing in the plugin you want to use as a parameter. Here is a simple example: //Introduce and use the plug-in

A colleague got stuck due to a bug pointed by this. Vue2’s this pointing problem caused an arrow function to be used, resulting in the inability to get the corresponding props. He didn't know it when I introduced it to him, and then I deliberately looked at the front-end communication group. So far, at least 70% of front-end programmers still don't understand it. Today I will share with you this link. If everything is wrong If you haven’t learned it yet, please give me a big mouth.
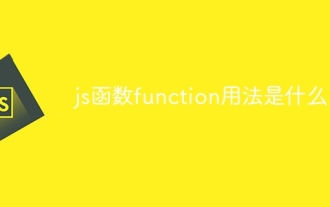
The usage of js function function is: 1. Declare function; 2. Call function; 3. Function parameters; 4. Function return value; 5. Anonymous function; 6. Function as parameter; 7. Function scope; 8. Recursive function.
