Laravel 514 + Bootstrap 334 Note 2: Laravel Routing
1 Routing mechanism
Routing in MVC is a very important function. Its function is:
A. Match the incoming request and the parameters attached to the request according to the user access (URL);
B. Call Request to map the Controller's Action method and pass in the parameters;
C. Return the Action method processing result;
The following figure represents a user request in a simple form:
2 In Laravel Routing
In Laravel 5.1.4, the routing configuration file is app/Http/routes.php.
2.1 Directly return string routing
Append the following code segment after the original code:
Route::get('/hw', function () { return 'Hello World'; });
2.2 Route to return the view
Add the following code segment to the above code:
Route::get('/home', function () { return view('home'); });
<html> <body> <h1>home</h1> </body> </html>

What if the code in the above example needs to pass parameters to the view page? Modify our routing code:
Route::get('/home', function () { return view('home', ['name' => '张三']); });
<html> <body> [<?php echo $name; ?>],您好! </body> </html>
If there are too many views, they are usually stored by module or even by function. Create a new directory in the resources/views directory: public/demo, and then move home.php to this directory.
Modify the routing code in the above example to:
Route::get('/home', function () { return view('public.demo.home', ['name' => '张三']); });
2.3 Routing Parameters
As mentioned before, routing can match the user’s request parameters, so how to match? Append the code snippet to the routing file in the above example:
Route::get('user/{name}', function($name) { return '用户姓名:'.$name; });
What if there are two parameters? Woolen cloth? Modify the routing code:
Route::get('user/{name}/{age}', function($name,$age) { return '用户姓名:'.$name.',年龄:'.$age; });
What if the age parameter is not necessary? Modify the routing code again:
Route::get('user/{name}/{age?}', function($name,$age=null) { return '用户姓名:'.$name.',年龄:'.$age; });
2.4 Constraints on routing parameters
Under normal circumstances, some parameters accessed by users have certain Rules, for example, the user ID when reading user information may be a number, the news ID when modifying news information may be a GUID, etc.
Modify the routes.php file and add the following code:
Route::get('new/{id}', function($id) { return '新闻ID:'.$id; })->where('id', '[0-9]+');
When you can access http://localhost:801/new/abc, the provided page does not exist:
Correspondingly, when multiple parameters are restricted at the same time, you need to use an array , modify the routing code of the above example:
Route::get('new/{id}/{title}', function($id,$title) { return '新闻ID:'.$id.',新闻标题:'.$title; })->where(['id' => '[0-9]+', 'title' => '[a-z]+']);
In addition, we can configure global restrictions, open the file: app/Providers/RouteServiceProviders.php, and modify the boot method as follows:
public function boot(Router $router) { // $router->pattern('id', '[0-9]+'); parent::boot($router); }
Modify the routing code in the above example to:
Route::get('new/{id}', function($id) { return '新闻ID:'.$id; });
2.5 Get routing parameters
You can get routing parameters in routes.php to do other operations. Modify the routing code in the above example:
Route::get('new/{id}', function(Request $request, $id) { if ($request->route('id') == '2') { return '新闻ID是2'; }else{ return '新闻ID不是2,值是:'.$id; } });
in routes.php Insert a new line after php:
use Illuminate\Http\Request;
Open the browser and visit http://localhost:801/new/2 and http://localhost:801/new/3 respectively You can see different page effects.
Routing still has many complex functions that need to be studied.
Copyright Statement: This article is an original article by the blogger and may not be reproduced without the blogger's permission.
The above introduces Laravel 514 + Bootstrap 334 Note 2: Laravel routing, including aspects of content, I hope it will be helpful to friends who are interested in PHP tutorials.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


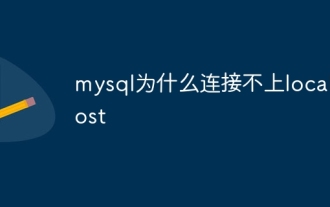
The reasons why mysql cannot connect to localhost are that the mysql service is not started, the mysql port is occupied, and there is a problem with the MySQL configuration file. Detailed introduction: 1. In Windows systems, you can open the service manager by entering "services.msc" at the command prompt, then find the mysql service and ensure that its status is "Running". In Linux systems, you can use the "services.msc" command to check and control the service status; 2. You can use the open command and so on.
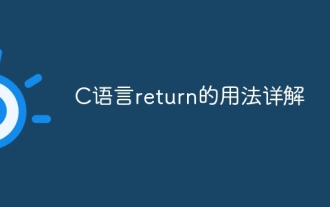
The usage of return in C language is: 1. For functions whose return value type is void, you can use the return statement to end the execution of the function early; 2. For functions whose return value type is not void, the function of the return statement is to end the execution of the function. The result is returned to the caller; 3. End the execution of the function early. Inside the function, we can use the return statement to end the execution of the function early, even if the function does not return a value.

Function means function. It is a reusable code block with specific functions. It is one of the basic components of a program. It can accept input parameters, perform specific operations, and return results. Its purpose is to encapsulate a reusable block of code. code to improve code reusability and maintainability.

Solution: 1. Check the running status of the server and make sure it is listening on the correct port; 2. Try to temporarily disable the firewall or security software, and then try to access localhost again; 3. Check the hosts file of the operating system to ensure that localhost is resolved correctly ; 4. Try to restart the network adapter or reconfigure the network connection; 5. Try to change the port used by the local server, or close other programs that occupy the same port; 6. Try to manually add the corresponding IP address and domain name in the hosts file, etc.
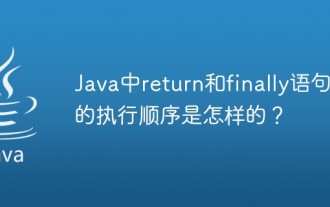
Source code: publicclassReturnFinallyDemo{publicstaticvoidmain(String[]args){System.out.println(case1());}publicstaticintcase1(){intx;try{x=1;returnx;}finally{x=3;}}}#Output The output of the above code can simply conclude: return is executed before finally. Let's take a look at what happens at the bytecode level. The following intercepts part of the bytecode of the case1 method, and compares the source code to annotate the meaning of each instruction in
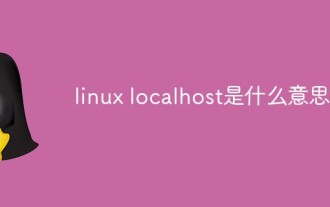
linux localhost means "computer host name". The host name is used to identify an independent computer on the network; the root in "root@localhost" represents the currently logged-in user. In Linux, the administrator account is root, and the user is root. Log in to the Linux machine as a user.
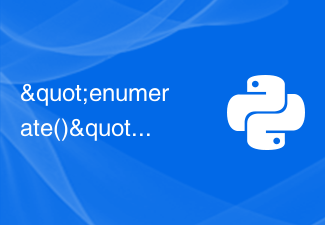
In this article, we will learn about enumerate() function and the purpose of “enumerate()” function in Python. What is the enumerate() function? Python's enumerate() function accepts a data collection as a parameter and returns an enumeration object. Enumeration objects are returned as key-value pairs. The key is the index corresponding to each item, and the value is the items. Syntax enumerate(iterable,start) Parameters iterable - The passed in data collection can be returned as an enumeration object, called iterablestart - As the name suggests, the starting index of the enumeration object is defined by start. if we ignore
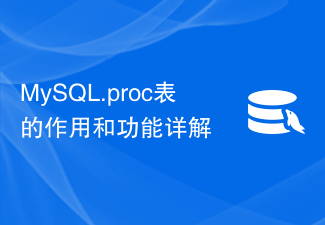
Detailed explanation of the role and function of the MySQL.proc table. MySQL is a popular relational database management system. When developers use MySQL, they often involve the creation and management of stored procedures (StoredProcedure). The MySQL.proc table is a very important system table. It stores information related to all stored procedures in the database, including the name, definition, parameters, etc. of the stored procedures. In this article, we will explain in detail the role and functionality of the MySQL.proc table
