Alternative method for session storage: redis
php uses files to store sessions by default. If the amount of concurrency is large, the efficiency is very low. Redis has very good support for high concurrency, so you can use redis to replace file storage sessions.
Here, we introduce the function and usage of PHP’s session_set_save_handler
function. This function defines user-level session saving functions (such as open, close, write, etc.). The prototype is as follows:
<code>bool session_set_save_hanler(callback open,callback close,callback read,callback write,callback destory,callback gc) </code>
Before using this function, set the session.save_handler option in the php.ini configuration file to user, otherwise session_set_save_handle will not take effect.
The following is an example of using redis to store sessions.
Write a session management class sessionManager, the code is as follows:
<code><?php class SessionManager { private $reids; private $sessionSavePath; private $sessionName; private $sessi public function __construct() { $this->reids=new Redis(); $this->reids->connect('127.0.0.1',6379); $retval=session_set_save_handler( array($this,"open"), array($this,"close"), array($this,"read"), array($this,"write"), array($this,"destroy"), array($this,"gc") ); } public function open($path,$name) { return true; } public function close() { return true; } public function read($id) { $vale=$this->reids->get($id); if($vale) { return $vale; }else { return ''; } } public function write($id,$data) { if($this->reids->set($id,$data)) { $this->reids->expire($id,$this->sessionExpireTime); return true; } return false; } public function destroy($id) { if($this->reids->delete($id)) { return true; } return false; } public function gc($maxlifetime) { return true; } public function __destruct() { session_write_close(); } } ?> </code>
The SessionManager constructor is mainly used to connect to the Redis server, use the session_set_save_handler function to set the session callback function, and call the session_start function to enable the session function. Because the open, close and gc callback functions in this example are not very useful, they return true directly.
In the write callback function, use the session id as the key, store the session data as the value in the redis server, and set the session expiration time to 300 seconds. In the read callback function, the session ID is used as the key to read data from the redis server and return this data. In the destroy callback function, the session ID is used as the key to delete the corresponding session data from the redis server.
To use, just include the SessionManager class and instantiate a SessionManager object. Create a session_set.php file below. Enter the code
<code><?php include("SessionManager.php"); new SessionManager(); //开启session管理 $_SESSION['username']='hezikuang';//创建session变量 ?> </code>
and then create a session_get.php file. Enter the following code:
<code><?php include("SessionManager.php"); new SessionManager(); //开启session管理 echo $_SESSION['username']; ?> </code>
You can access session_get.php to see if it is successful.
Copyright Statement: This article is an original article by the blogger and may not be reproduced without the blogger's permission.
The above introduces the alternative method of session storage, redis, including the relevant content. I hope it will be helpful to friends who are interested in PHP tutorials.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
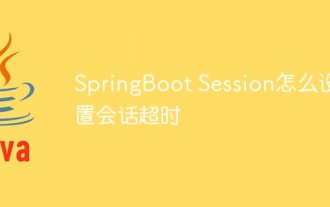
The problem was found in the springboot project production session-out timeout. The problem is described below: In the test environment, the session-out was configured by changing the application.yaml. After setting different times to verify that the session-out configuration took effect, the expiration time was directly set to 8 hours for release. Arrived in production environment. However, I received feedback from customers at noon that the project expiration time was set to be short. If no operation is performed for half an hour, the session will expire and require repeated logins. Solve the problem of handling the development environment: the springboot project has built-in Tomcat, so the session-out configured in application.yaml in the project is effective. Production environment: Production environment release is
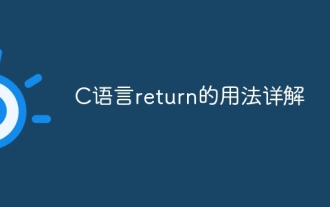
The usage of return in C language is: 1. For functions whose return value type is void, you can use the return statement to end the execution of the function early; 2. For functions whose return value type is not void, the function of the return statement is to end the execution of the function. The result is returned to the caller; 3. End the execution of the function early. Inside the function, we can use the return statement to end the execution of the function early, even if the function does not return a value.
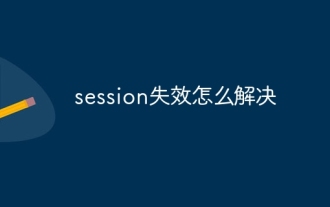
Session failure is usually caused by the session lifetime expiration or server shutdown. The solutions: 1. Extend the lifetime of the session; 2. Use persistent storage; 3. Use cookies; 4. Update the session asynchronously; 5. Use session management middleware.
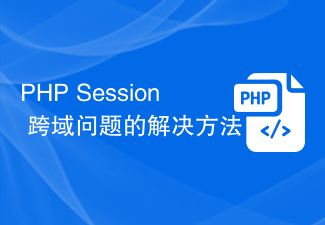
Solution to the cross-domain problem of PHPSession In the development of front-end and back-end separation, cross-domain requests have become the norm. When dealing with cross-domain issues, we usually involve the use and management of sessions. However, due to browser origin policy restrictions, sessions cannot be shared by default across domains. In order to solve this problem, we need to use some techniques and methods to achieve cross-domain sharing of sessions. 1. The most common use of cookies to share sessions across domains

Function means function. It is a reusable code block with specific functions. It is one of the basic components of a program. It can accept input parameters, perform specific operations, and return results. Its purpose is to encapsulate a reusable block of code. code to improve code reusability and maintainability.
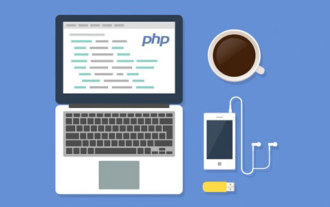
Solution to the problem that the php session disappears after refreshing: 1. Open the session through "session_start();"; 2. Write all public configurations in a php file; 3. The variable name cannot be the same as the array subscript; 4. In Just check the storage path of the session data in phpinfo and check whether the sessio in the file directory is saved successfully.
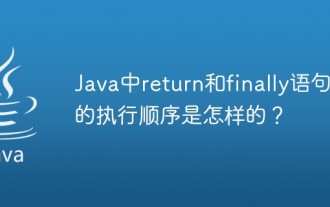
Source code: publicclassReturnFinallyDemo{publicstaticvoidmain(String[]args){System.out.println(case1());}publicstaticintcase1(){intx;try{x=1;returnx;}finally{x=3;}}}#Output The output of the above code can simply conclude: return is executed before finally. Let's take a look at what happens at the bytecode level. The following intercepts part of the bytecode of the case1 method, and compares the source code to annotate the meaning of each instruction in
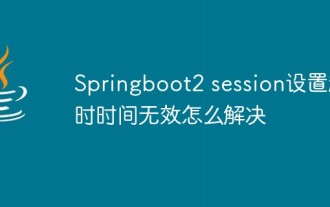
Problem: Today, we encountered a setting timeout problem in our project, and changes to SpringBoot2’s application.properties never took effect. Solution: The server.* properties are used to control the embedded container used by SpringBoot. SpringBoot will create an instance of the servlet container using one of the ServletWebServerFactory instances. These classes use server.* properties to configure the controlled servlet container (tomcat, jetty, etc.). When the application is deployed as a war file to a Tomcat instance, the server.* properties do not apply. They do not apply,
