


javascript - Ajax is combined with file in html5 to implement file upload, and PHP is used to receive it in the background. How to use PHP to receive the passed formData?
How to use PHP to receive files transmitted from the front end? What type of binary data does the file of the formdata object contain?
Front-end code:
<code><input type="file" name="file" id="file" multiple> <script type="text/javascript"> var file = document.querySelector("#file"); file.onchange = function(){ var files = this.files; for(var i=0;i<files.length;i++){ ajax('ajax.php',files[i],function(data){ console.log(data) console.log('fn') }) } } function ajax(url,data,fn){ var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function(){ if(xhr.readyState==4&&xhr.status==200){ fn(xhr.responseText) } } var formData = new FormData(); formData.append('file',data); xhr.open('POST',url,true); //xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); xhr.send(formData); } </script></code>
php code:
<code><?php if(!empty($_FILES['file'])){ $fileinfo = $_FILES['file']; $destination = "image/"; $destination = $destination.basename($_FILES['file']['name']); move_uploaded_file($fileinfo['tmp_name'],$destination); echo "succ"; } ?></code>
I don’t know why the judgment of if(!empty($_FILES['file'])) is always false, and the same is true when using if(!empty($_POST['file'])); Does anyone know how the background receives the transmission? Did you get the documents? Can you give me a demo PHP file?
Reply content:
How to use PHP to receive files transmitted from the front end? What type of binary data does the file of the formdata object contain?
Front-end code:
<code><input type="file" name="file" id="file" multiple> <script type="text/javascript"> var file = document.querySelector("#file"); file.onchange = function(){ var files = this.files; for(var i=0;i<files.length;i++){ ajax('ajax.php',files[i],function(data){ console.log(data) console.log('fn') }) } } function ajax(url,data,fn){ var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function(){ if(xhr.readyState==4&&xhr.status==200){ fn(xhr.responseText) } } var formData = new FormData(); formData.append('file',data); xhr.open('POST',url,true); //xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); xhr.send(formData); } </script></code>
php code:
<code><?php if(!empty($_FILES['file'])){ $fileinfo = $_FILES['file']; $destination = "image/"; $destination = $destination.basename($_FILES['file']['name']); move_uploaded_file($fileinfo['tmp_name'],$destination); echo "succ"; } ?></code>
I don’t know why the judgment of if(!empty($_FILES['file'])) is always false, and the same is true when using if(!empty($_POST['file'])); Does anyone know how the background receives the transmission? Did you get the documents? Can you give me a demo PHP file?
I added 3 log records in php:
<code>file_put_contents('/tmp/tmp.log', '$_FILES'.":\n".print_r($_FILES, true)."\n\n", FILE_APPEND); file_put_contents('/tmp/tmp.log', '$_POST'.":\n".print_r($_POST, true)."\n\n", FILE_APPEND); file_put_contents('/tmp/tmp.log', '$_SERVER'.":\n".print_r($_SERVER, true)."\n\n", FILE_APPEND);</code>
The storage path has been changed to tmp, but other things have not changed. The result is:
<code>[root@localhost tmp]# cat tmp.log $_FILES: Array ( [file] => Array ( [name] => Screenshot_2010-01-01-08-11-30.png [type] => image/png [tmp_name] => /tmp/phposvIcw [error] => 0 [size] => 30920 ) ) $_POST: Array ( ) $_SERVER: Array ( [USER] => nginx [HOME] => /var/lib/nginx [FCGI_ROLE] => RESPONDER [SCRIPT_FILENAME] => /var/www/test.php [SCRIPT_NAME] => /test.php [PATH_INFO] => [QUERY_STRING] => [REQUEST_METHOD] => POST [CONTENT_TYPE] => multipart/form-data; boundary=----WebKitFormBoundaryiJpJZSxazdqa8hzb [CONTENT_LENGTH] => 31127 [REQUEST_URI] => /test.php [DOCUMENT_URI] => /test.php [DOCUMENT_ROOT] => /var/www [SERVER_PROTOCOL] => HTTP/1.1 [GATEWAY_INTERFACE] => CGI/1.1 [SERVER_SOFTWARE] => nginx/1.6.3 [REMOTE_ADDR] => 192.168.255.1 [REMOTE_PORT] => 60032 [SERVER_ADDR] => 192.168.255.128 [SERVER_PORT] => 80 [SERVER_NAME] => [REDIRECT_STATUS] => 200 [HTTP_HOST] => 192.168.255.128 [HTTP_CONNECTION] => keep-alive [HTTP_CONTENT_LENGTH] => 31127 [HTTP_ORIGIN] => http://192.168.255.128 [HTTP_USER_AGENT] => Mozilla/5.0 (Windows NT 6.1; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/51.0.2704.103 Safari/537.36 [HTTP_CONTENT_TYPE] => multipart/form-data; boundary=----WebKitFormBoundaryiJpJZSxazdqa8hzb [HTTP_ACCEPT] => */* [HTTP_DNT] => 1 [HTTP_REFERER] => http://192.168.255.128/test.html [HTTP_ACCEPT_ENCODING] => gzip, deflate [HTTP_ACCEPT_LANGUAGE] => zh-CN,zh;q=0.8,ja;q=0.6,en;q=0.4 [PHP_SELF] => /test.php [REQUEST_TIME_FLOAT] => 1470377177.1168 [REQUEST_TIME] => 1470377177 ) </code>
File uploaded successfully
<code>[root@localhost tmp]# ll total 36 -rw-r--r-- 1 nginx nginx 30920 Aug 5 14:06 Screenshot_2010-01-01-08-11-30.png -rw-r--r-- 1 nginx nginx 1705 Aug 5 14:06 tmp.log [root@localhost tmp]# </code>
Nothing wrong...
Let’s print it out first! Don’t judge with if
yet. Direct
<code>echo 'FILES:'.var_dump($_FILES); echo 'POST:'.var_dump($_POST); die();</code>
Look what it is. Then do it step by step
F12 to see the request
The poster uses $_REQUES
to receive it and try it.
You can also directly upload base64
encoding
Open the chrome console to see what the request sent, mainly look at the Content-Type of the request header and the request body

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


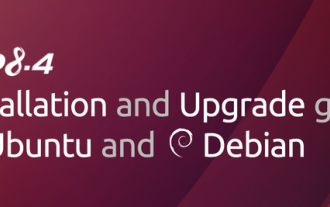
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
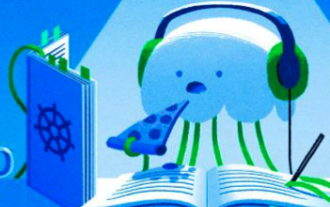
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
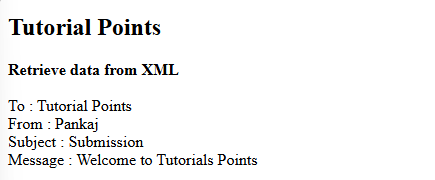
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
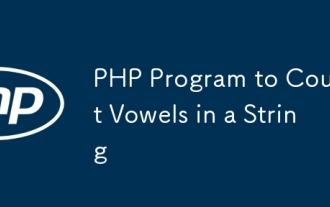
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
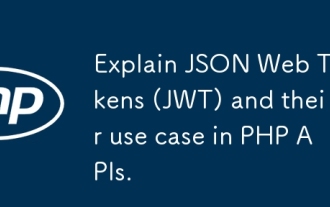
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
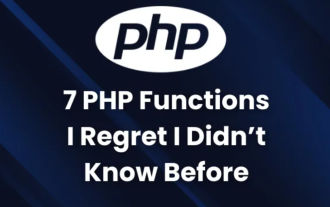
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
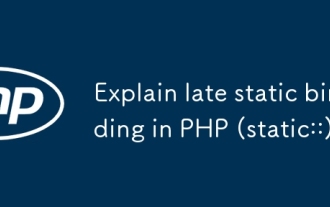
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
