


The use of the final static const keyword in PHP object-oriented
This keyword can only be used to define classes and methods. The final keyword cannot be used to define member properties, because final means constant. We use the define() function to define constants in PHP, so final cannot be used. to define member properties.
14. Application of the final keyword
This keyword can only be used to define classes and methods. The final keyword cannot be used to define member properties, because
final means constant. We define constants in PHP using is the define() function, so final cannot be used to define member properties.
Classes marked with the final key cannot be inherited;
Code snippets
final class Person{
… …
}
class Student extends Person{
}
The following error will appear:
Fatal error: Class Student may not inherit from final class (Person)
Methods marked with the final key cannot be overridden by subclasses and are the final version;
code snippet
class Person{
final function say() {
}
}
class Student extends Person{
function say() {
}
}
The following error will occur:
Fatal error: Cannot override final method Person::say()
15. Use of static and const keywords
Static keyword is to describe member properties and member methods in the class as static ;What are the benefits of static members?
We declared the human being "Person" earlier. If we add an attribute of "the country to which the person belongs" to the "Person" class, hundreds or more instances can be instantiated using the "Person" class. Object, each object has the attribute of "country to which it belongs". If the project is developed for the Chinese, then each object has the attribute of "China" and other attributes of the country. is different. If we make the "country" attribute a static
member, then there will be only one country attribute in the memory, and let hundreds or more objects share this attribute, the
static member can Restrict external access, because static members belong to the class and do not belong to any object instance. It is the space allocated when the class is loaded for the first time. It is inaccessible to other classes and is only shared with instances of the class. It can protect the members of the class to a certain extent; Let’s analyze it from the perspective of memory. The memory is logically divided into four segments, in which the object is placed in the "heap memory", and the reference of the object is placed in the "heap memory". In the "stack memory", the static members are placed in the "initialization static segment", which is placed when the class is loaded for the first time. It can be shared by every object in the heap memory, as shown below;
Static variables of a class are very similar to global variables and can be shared by all instances of the class. The same is true for static methods of a class
, similar to global functions.
Code snippet
Copy the code The code is as follows:
class Person{
//The following are the static member attributes of the person public static $myCountry="China"; // var $name ; //The name of the person //This is the static member method of the person public static function say(){
echo "I am Chinese";
}
}
//Output static attributes
echo Person::$myCountry;
//Access static methods
Person::say();
//Reassign static properties
Person::$myCountry="United States";
echo Person::$myCountry;
?> ;
Because static members are created when the class is loaded for the first time, you can access the static members by using the class
name without requiring an object outside the class; as mentioned above, static members are used by every member of this class. Shared by an instance object, then can we access static members in a class using objects? From the picture above, we can see that static members do not exist inside each object, but each object can be shared, so if we use objects to access members, there will be no such attribute definition and use object access. If there are no static members, in other object-oriented languages, such as Java, you can use objects to access static members. If you can use objects to access static members in PHP, we should try not to use them because static members When we are working on a project, our purpose is to use the class name to access the members.
Static methods in a class can only access the static attributes of the class. Static methods in the class cannot access non-static members of the class. The reason is very simple. We want to access this class in the method of this class. For other members, we need to use the reference $this
, and the reference pointer $this represents the object that calls this method. We said that static methods are not called with objects
, but are accessed using the class name, so it is simply If no object exists, there is no reference to $this. Without the reference to $this, non-static members in the class cannot be accessed. And because static members in the class can be accessed without objects, so the Static methods can only access static properties of the class. Since $this does not exist, we use a special class "self" to access other static members in the static method; self is similar to $this, except that self is the representative
The class where this static method is located. So in a static method, you can use the "class name" of the class where the method is located, or you can use "self" to access other static members. If there are no special circumstances, we usually use the latter, that is, "self::
member attribute" method.
Code snippet
Copy the code
The code is as follows:
class Person{
//The following are the static member attributes of the person
public static $myCountry="China"; //This is the person Static member methods, access other static members through self public static function say(){ echo "I am".self::$myCountry."
"; }
//Access static methods
Person::say();
?>
Can you access static members in non-static methods? Of course it is possible, but you cannot use "$this"
References must also use class names or " self::form of member attribute".
const is a keyword that defines constants. To define constants in PHP, you use the "define()" function, but
to define constants in a class, you use the "const" keyword, which is similar to # in C. define If its value is changed in the program, an error will occur. The member attributes modified with "const" are accessed in the same way as the members modified with "static". The "class name" is also used. In the method The "self" keyword is used inside. But you don't need to use the "$"
symbol, nor can you use objects to access it.
Code snippet
The code is as follows:
class MyClass{
//Define a constant constant
const constant = 'constant value';
function showConstant() {
echo self ::constant . "n"; //Use self to access, do not add "$" } } echo MyClass::constant . "n"; //Use class name to access, do not add "$" $ class = new MyClass();
$class->showConstant();// echo $class::constant; is not allowed
The above introduces the use of the final static const keyword in PHP object-oriented, including the content. I hope it will be helpful to friends who are interested in PHP tutorials.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


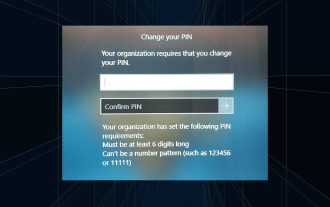
The message "Your organization has asked you to change your PIN" will appear on the login screen. This happens when the PIN expiration limit is reached on a computer using organization-based account settings, where they have control over personal devices. However, if you set up Windows using a personal account, the error message should ideally not appear. Although this is not always the case. Most users who encounter errors report using their personal accounts. Why does my organization ask me to change my PIN on Windows 11? It's possible that your account is associated with an organization, and your primary approach should be to verify this. Contacting your domain administrator can help! Additionally, misconfigured local policy settings or incorrect registry keys can cause errors. Right now
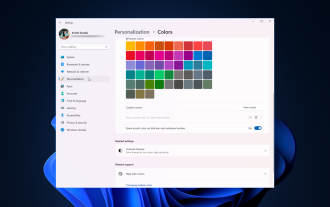
Windows 11 brings fresh and elegant design to the forefront; the modern interface allows you to personalize and change the finest details, such as window borders. In this guide, we'll discuss step-by-step instructions to help you create an environment that reflects your style in the Windows operating system. How to change window border settings? Press + to open the Settings app. WindowsI go to Personalization and click Color Settings. Color Change Window Borders Settings Window 11" Width="643" Height="500" > Find the Show accent color on title bar and window borders option, and toggle the switch next to it. To display accent colors on the Start menu and taskbar To display the theme color on the Start menu and taskbar, turn on Show theme on the Start menu and taskbar
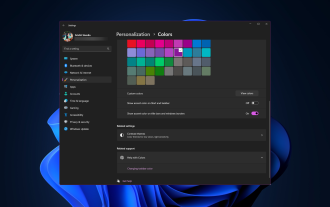
By default, the title bar color on Windows 11 depends on the dark/light theme you choose. However, you can change it to any color you want. In this guide, we'll discuss step-by-step instructions for three ways to change it and personalize your desktop experience to make it visually appealing. Is it possible to change the title bar color of active and inactive windows? Yes, you can change the title bar color of active windows using the Settings app, or you can change the title bar color of inactive windows using Registry Editor. To learn these steps, go to the next section. How to change title bar color in Windows 11? 1. Using the Settings app press + to open the settings window. WindowsI go to "Personalization" and then
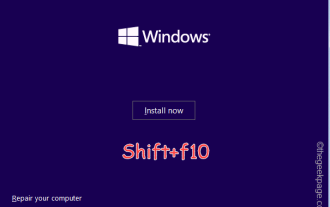
Do you see "A problem occurred" along with the "OOBELANGUAGE" statement on the Windows Installer page? The installation of Windows sometimes stops due to such errors. OOBE means out-of-the-box experience. As the error message indicates, this is an issue related to OOBE language selection. There is nothing to worry about, you can solve this problem with nifty registry editing from the OOBE screen itself. Quick Fix – 1. Click the “Retry” button at the bottom of the OOBE app. This will continue the process without further hiccups. 2. Use the power button to force shut down the system. After the system restarts, OOBE should continue. 3. Disconnect the system from the Internet. Complete all aspects of OOBE in offline mode
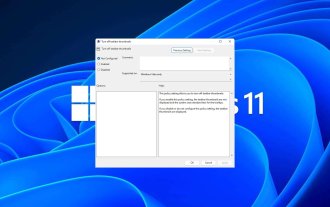
Taskbar thumbnails can be fun, but they can also be distracting or annoying. Considering how often you hover over this area, you may have inadvertently closed important windows a few times. Another disadvantage is that it uses more system resources, so if you've been looking for a way to be more resource efficient, we'll show you how to disable it. However, if your hardware specs can handle it and you like the preview, you can enable it. How to enable taskbar thumbnail preview in Windows 11? 1. Using the Settings app tap the key and click Settings. Windows click System and select About. Click Advanced system settings. Navigate to the Advanced tab and select Settings under Performance. Select "Visual Effects"
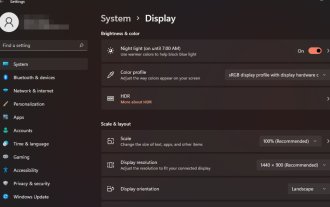
We all have different preferences when it comes to display scaling on Windows 11. Some people like big icons, some like small icons. However, we all agree that having the right scaling is important. Poor font scaling or over-scaling of images can be a real productivity killer when working, so you need to know how to customize it to get the most out of your system's capabilities. Advantages of Custom Zoom: This is a useful feature for people who have difficulty reading text on the screen. It helps you see more on the screen at one time. You can create custom extension profiles that apply only to certain monitors and applications. Can help improve the performance of low-end hardware. It gives you more control over what's on your screen. How to use Windows 11
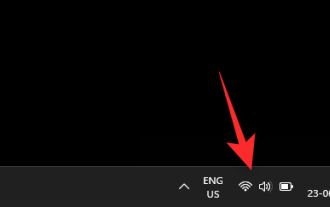
Screen brightness is an integral part of using modern computing devices, especially when you look at the screen for long periods of time. It helps you reduce eye strain, improve legibility, and view content easily and efficiently. However, depending on your settings, it can sometimes be difficult to manage brightness, especially on Windows 11 with the new UI changes. If you're having trouble adjusting brightness, here are all the ways to manage brightness on Windows 11. How to Change Brightness on Windows 11 [10 Ways Explained] Single monitor users can use the following methods to adjust brightness on Windows 11. This includes desktop systems using a single monitor as well as laptops. let's start. Method 1: Use the Action Center The Action Center is accessible
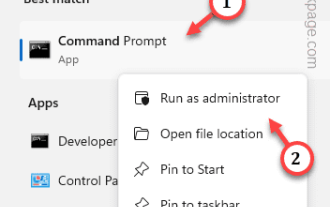
The activation process on Windows sometimes takes a sudden turn to display an error message containing this error code 0xc004f069. Although the activation process is online, some older systems running Windows Server may experience this issue. Go through these initial checks, and if they don't help you activate your system, jump to the main solution to resolve the issue. Workaround – close the error message and activation window. Then restart the computer. Retry the Windows activation process from scratch again. Fix 1 – Activate from Terminal Activate Windows Server Edition system from cmd terminal. Stage – 1 Check Windows Server Version You have to check which type of W you are using
