[nginx source code analysis]ngx memory pool implementation
Memory pool function:
ngx_create_pool ngx_destroy_pool ngx_reset_pool ngx_palloc ngx_pnalloc ngx_palloc_block ngx_palloc_large ngx_pool_cleanup_add
Memory pool creation
ngx_pool_t * ngx_create_pool(size_t size, ngx_log_t *log) { ngx_pool_t *p; p = ngx_memalign(NGX_POOL_ALIGNMENT, size, log);//按照16字节对齐分配一块size大小内存 if (p == NULL) { return NULL; } p->d.last = (u_char *) p + sizeof(ngx_pool_t); //last指向数据部分开始(跳过header ngx_pool_t)结构 p->d.end = (u_char *) p + size; //end指向末尾结构 p->d.next = NULL; //next是对小块内存链表管理指针 p->d.failed = 0; //此块内存分配失败次数 size = size - sizeof(ngx_pool_t); //去掉ngx_pool_t内存池的实际大小 p->max = (size < NGX_MAX_ALLOC_FROM_POOL) ? size : NGX_MAX_ALLOC_FROM_POOL;//最大内存池节点大小为4095 p->current = p; //current指向p p->chain = NULL; p->large = NULL; p->cleanup = NULL; p->log = log; return p; }
The initialization structure is as shown below:

The green line indicates the structure members, for example, the members of ngx_pool_data_t include last, end, next, failed
The black line Represents the address pointed by the pointer
1 max selects the smallest one between size-sizeof(ngx_pool_t) and 4095
2 log calls the ngx_log_t structure created by ngx_log_init in main (that is, the global ngx_log object)
Principle:
The starting position where nginx actually allocates space from this memory pool starts from d.last. As the memory pool space is allocated externally, the pointer of this field will move backward.
Memory pool allocation
void * ngx_palloc(ngx_pool_t *pool, size_t size) { u_char *m; ngx_pool_t *p; //如果申请的内存大小小于创建的内存池节点大小(当然是去掉ngx_pool_t后) if (size <= pool->max) { p = pool->current; do { m = ngx_align_ptr(p->d.last, NGX_ALIGNMENT);//首先对齐last指针 if ((size_t) (p->d.end - m) >= size) { p->d.last = m + size; return m; } p = p->d.next; } while (p); return ngx_palloc_block(pool, size); } return ngx_palloc_large(pool, size); }
ngx_palloc() tries to allocate space from the pool memory There are two situations when allocating space of size size in the pool.
In the first case, if the allocated size is smaller than ngx_pool_t.max (small block allocation, code 127-140), you first need to align the last 16 bits. If there is still space in the application, then use this memory node (code 130) ) at the same time moves last to point to the allocated address, and returns the requested memory to the caller. If there is no space that can be allocated when traversing the small memory node from current (that is, traversing the linked list until the memory pool node next is empty). You need to allocate a memory pool node and call ngx_palloc_block(pool,size)
This function mainly allocates a memory pool node, then inserts this node into the end of the linked list, and updates the pool->current pointer at the same time. The update algorithm is to traverse the linked list. If it is found If this node has failed to be allocated 6 times, current will point to the next node. If all nodes have failed more than 6 times, current will point to the newly allocated node.
The core code is as follows:
current = pool->current;//初始化 for (p = current; p->d.next; p = p->d.next) {//遍历 if (p->d.failed++ > 4) {//分配超过6次 current = p->d.next; } } p->d.next = new;//插入新节点 pool->current = current ? current : new;//更新current

In the second case, allocate a large block of memory and call malloc directly to allocate it. After allocation, traverse the pool->large linked list. If there is a large memory pool node and alloc is empty, then Hang the newly allocated memory on this node. If there is no such node, then allocate and insert the head of the linked list of p->large.
Clear callback function
where each node is
struct ngx_pool_cleanup_s { ngx_pool_cleanup_pt handler;//回调handler void *data; //回调函数参数 ngx_pool_cleanup_t *next; //指向下一个节点 };
ngx_pool_cleanup_t * ngx_pool_cleanup_add(ngx_pool_t *p, size_t size) { ngx_pool_cleanup_t *c; c = ngx_palloc(p, sizeof(ngx_pool_cleanup_t));//分配一个节点 if (c == NULL) { return NULL; } if (size) { c->data = ngx_palloc(p, size); if (c->data == NULL) { return NULL; } } else { c->data = NULL; } c->handler = NULL; c->next = p->cleanup;//插入链表头 p->cleanup = c;//插入链表头部 ngx_log_debug1(NGX_LOG_DEBUG_ALLOC, p->log, 0, "add cleanup: %p", c); return c; }
The above introduces [nginx source code analysis] ngx memory pool implementation, including aspects of the content. I hope it will be helpful to friends who are interested in PHP tutorials.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


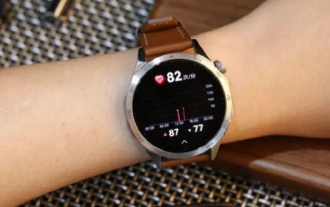
Many users will choose the Huawei brand when choosing smart watches. Among them, Huawei GT3pro and GT4 are very popular choices. Many users are curious about the difference between Huawei GT3pro and GT4. Let’s introduce the two to you. . What are the differences between Huawei GT3pro and GT4? 1. Appearance GT4: 46mm and 41mm, the material is glass mirror + stainless steel body + high-resolution fiber back shell. GT3pro: 46.6mm and 42.9mm, the material is sapphire glass + titanium body/ceramic body + ceramic back shell 2. Healthy GT4: Using the latest Huawei Truseen5.5+ algorithm, the results will be more accurate. GT3pro: Added ECG electrocardiogram and blood vessel and safety

Use Java's File.length() function to get the size of a file. File size is a very common requirement when dealing with file operations. Java provides a very convenient way to get the size of a file, that is, using the length() method of the File class. . This article will introduce how to use this method to get the size of a file and give corresponding code examples. First, we need to create a File object to represent the file we want to get the size of. Here is how to create a File object: Filef
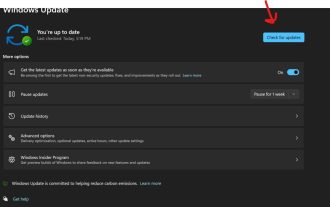
Why Snipping Tool Not Working on Windows 11 Understanding the root cause of the problem can help find the right solution. Here are the top reasons why the Snipping Tool might not be working properly: Focus Assistant is On: This prevents the Snipping Tool from opening. Corrupted application: If the snipping tool crashes on launch, it might be corrupted. Outdated graphics drivers: Incompatible drivers may interfere with the snipping tool. Interference from other applications: Other running applications may conflict with the Snipping Tool. Certificate has expired: An error during the upgrade process may cause this issu simple solution. These are suitable for most users and do not require any special technical knowledge. 1. Update Windows and Microsoft Store apps
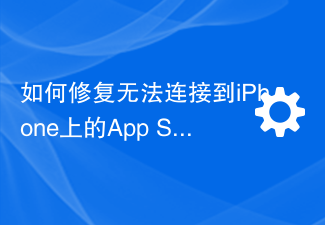
Part 1: Initial Troubleshooting Steps Checking Apple’s System Status: Before delving into complex solutions, let’s start with the basics. The problem may not lie with your device; Apple's servers may be down. Visit Apple's System Status page to see if the AppStore is working properly. If there's a problem, all you can do is wait for Apple to fix it. Check your internet connection: Make sure you have a stable internet connection as the "Unable to connect to AppStore" issue can sometimes be attributed to a poor connection. Try switching between Wi-Fi and mobile data or resetting network settings (General > Reset > Reset Network Settings > Settings). Update your iOS version:
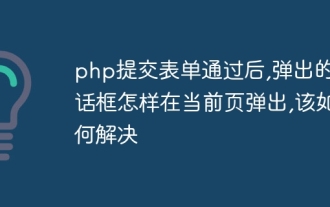
php提交表单通过后,弹出的对话框怎样在当前页弹出php提交表单通过后,弹出的对话框怎样在当前页弹出而不是在空白页弹出?想实现这样的效果:而不是空白页弹出:------解决方案--------------------如果你的验证用PHP在后端,那么就用Ajax;仅供参考:HTML code
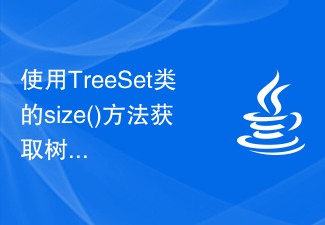
Title: Use the size() method of the TreeSet class to obtain the number of elements in the tree collection. Introduction TreeSet is an ordered collection in the Java collection framework. It implements the SortedSet interface and uses the red-black tree data structure to implement it. TreeSet can be sorted according to the natural order of elements, or by using a Comparator custom comparator. This article will introduce how to use the size() method of the TreeSet class to obtain the number of elements in the tree collection and provide
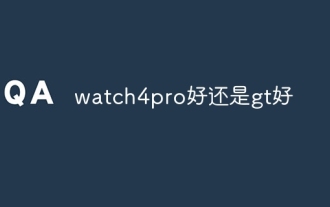
Watch4pro and gt each have different features and applicable scenarios. If you focus on comprehensive functions, high performance and stylish appearance, and are willing to bear a higher price, then Watch 4 Pro may be more suitable. If you don’t have high functional requirements and pay more attention to battery life and reasonable price, then the GT series may be more suitable. The final choice should be decided based on personal needs, budget and preferences. It is recommended to carefully consider your own needs before purchasing and refer to the reviews and comparisons of various products to make a more informed choice.
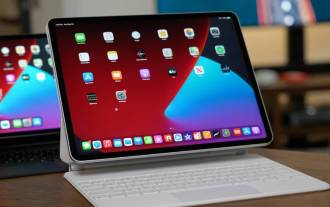
How to Optimize iPad Battery Life with iPadOS 17.4 Extending battery life is key to the mobile device experience, and the iPad is a good example. If you feel like your iPad's battery is draining too quickly, don't worry, there are a number of tricks and tweaks in iPadOS 17.4 that can significantly extend the run time of your device. The goal of this in-depth guide is not just to provide information, but to change the way you use your iPad, enhance your overall battery management, and ensure you can rely on your device for longer without having to charge it. By adopting the practices outlined here, you take a step toward more efficient and mindful use of technology that is tailored to your individual needs and usage patterns. Identify major energy consumers
