Image processing class (improved version)
<?php #封装一个image类 class Image{ private static $info; #图片基本信息 private static $image; #内存中的图片 public function __construct($src){ #判断文件 if(!is_file($src)){ return false; } #如果不是文件,直接返回 //获取图片信息 $info = getimagesize($src); self::$info=array( 'width'=>$info[0], 'height'=>$info[1], 'type'=>image_type_to_extension($info[2],false), 'mime'=>$info['mime'] ); //获取图片信息 $type=self::$info['type']; $fun ="imagecreatefrom{$type}"; self::$image = $fun($src); } /** * @param int $width $height 应该在配置文件中声明使用,可取消参数 * @return 缩略图 图片资源 * 缩略图的形成与使用 */ public function thumb($width ,$height){ //新建镇色彩图片 $image_thumb =imagecreatetruecolor($width ,$height); #获取图片的宽高比 $src_m = self::$info['width'] / self::$info['height']; #源文件空格比 $dst_m = $width / $height; #缩略图宽高比 #源文件图片是 N:1 型的 宽不变, 改变高 if($src_m > $dst_m ){ $cha_width = $width; $cha_height = ceil($width / $src_m) ; }else{ #源文件图片是 1:N 型的 高不变,改变宽 $cha_width = floor($height * $src_m) ; $cha_height = $height ; } #对缩略图的其实位置进行重置 $dst_x = ($width - $cha_width) /2 ; $dst_y = ($height - $cha_height) /2 ; imagecopyresampled($image_thumb ,self::$image , $dst_x , $dst_y ,0 , 0, $cha_width , $cha_height ,self::$info['width'],self::$info['height']); #生成缩略图 self::$image =$image_thumb; // #显示缩略图图片 // self::show(self::$image); #保存缩略图 self::save(self::getNewName()); //销毁图片 imagedestroy($this->image_thumb); #返回缩略图名字 return self::getNewName(); } #水印的生成坐标 private static function setLocal($pos){ #对 pos 参数进行判断 , 指定相应的水印生成坐标 #水印图片在config 文件中记录 $conf['mark'] switch ($pos) { case 1: $x = 0; $y = 0; break ; case 2: default: $x = self::$info['width']-50; $y = self::$info['height']-50; #不该是20 这个定制, 应该改成水印图片的宽高 } return $local=array('x' => $x ,'y'=>$y); } #添加文字水印 public function fontMark($content ,$font_url ,$size,$angle){ #字体颜色 $col = imagecolorallocatealpha(self::$image,mt_rand(200,255),mt_rand(200,255),mt_rand(200,255),20); #获取水印输出位置坐标 $local = self::setLocal(2); imagettftext(self::$image,$size,$angle,$local['x'],$local['y'],$col,$font_url,$content); #显示缩略图图片 self::show(self::$image); #保存文字水印 没有添加保存路径 self::save(self::getNewName()); #返回水印图片的名字 return self::getNewName(); } #添加图片水印 public function imageMark($url ,$alpha){ $info= getimagesize($url); #获取图片信息 $type=image_type_to_extension($info[2],false); $fun = "imagecreatefrom{$type}"; #获取水印输出位置坐标 $local = self::setLocal(2); $water = $fun($url); #水印图片 imagecopymerge(self::$image, $water, $local['x'], $local['y'],0 , 0,$info[0] , $info[1], 30); #销毁图片水印 imagedestroy($water); #显示缩略图图片 self::show(self::$image); #保存图片水印 没有添加保存路径 self::save(self::getNewName()); #返回水印图片的名字 return self::getNewName(); } #生成随机的 图片名字 /** * @return string 返回一个新的名字 * */ private static function getNewName(){ #获取一个时间 $str = time(); #获取随机字符串 $string = "qwertyuiopasdfghjklzxcvbnmQWERTYUIOASDFGHJKZXCVBNM1234567890"; for($i=0 ; $i<6 ; $i++){ $str .= $string[mt_rand(0 , strlen($string)-1)]; } return $str.self::$info['type']; } #在浏览器中输出图片 private static function show(){ header("content-type:".self::$info['mime']); $funs ="image".self::$info['type']; $funs(self::$image); } #把图片保存到硬盘 private static function save($newname){ $funs="image".self::$info['type']; $funs(self::$image,$newname.".".self::$info['type']); #在此处加入配置文件的生成目录 } #销毁图片 public function __destruct(){ imagedestroy(self::$image); } }
The above introduces the image processing class (improved version), including aspects of the content. I hope it will be helpful to friends who are interested in PHP tutorials.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
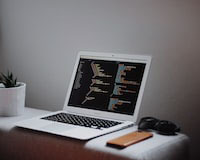
The reason for the error is NameResolutionError(self.host,self,e)frome, which is an exception type in the urllib3 library. The reason for this error is that DNS resolution failed, that is, the host name or IP address attempted to be resolved cannot be found. This may be caused by the entered URL address being incorrect or the DNS server being temporarily unavailable. How to solve this error There may be several ways to solve this error: Check whether the entered URL address is correct and make sure it is accessible Make sure the DNS server is available, you can try using the "ping" command on the command line to test whether the DNS server is available Try accessing the website using the IP address instead of the hostname if behind a proxy
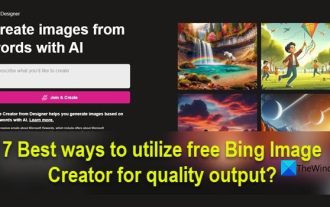
This article will introduce seven ways to get high-quality output using the free BingImageCreator. BingImageCreator (now known as ImageCreator for Microsoft Designer) is one of the great online artificial intelligence art generators. It generates highly realistic visual effects based on user prompts. The more specific, clear, and creative your prompts are, the better the results will be. BingImageCreator has made significant progress in creating high-quality images. It now uses Dall-E3 training mode, showing a higher level of detail and realism. However, its ability to consistently produce HD results depends on several factors, including fast
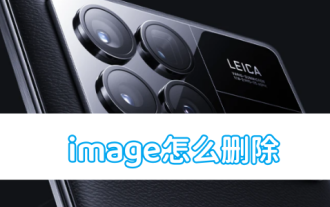
How to delete images on Xiaomi mobile phones? You can delete images on Xiaomi mobile phones, but most users don’t know how to delete images. Next is the tutorial on how to delete images on Xiaomi mobile phones brought by the editor. Interested users can come and join us. Let's see! How to delete images on Xiaomi mobile phone 1. First open the [Album] function in Xiaomi mobile phone; 2. Then check the unnecessary pictures and click the [Delete] button in the lower right corner; 3. Then click [Album] at the top to enter the special area , select [Recycle Bin]; 4. Then directly click [Empty Recycle Bin] as shown in the figure below; 5. Finally, directly click [Permanent Delete] to complete.
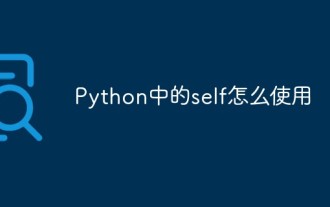
Before introducing the usage of self in Python, let’s first introduce the classes and instances in Python. We know that the most important concepts of object-oriented are classes and instances. Classes are abstract templates, such as abstract things like students. , can be represented by a Student class. Instances are specific "objects" created based on classes. Each object inherits the same methods from the class, but its data may be different. 1. Take the Student class as an example. In Python, the class is defined as follows: classStudent(object):pass(Object) indicates which class the class inherits from. The Object class is all
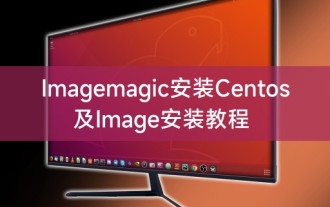
LINUX is an open source operating system. Its flexibility and customizability make it the first choice of many developers and system administrators. In the LINUX system, image processing is a very important task, and Imagemagick and Image are Two very popular image processing tools, this article will introduce you to how to install Imagemagick and Image in Centos system, and provide detailed installation tutorials. Imagemagic installation Centos tutorial Imagemagick is a powerful image processing toolset, which can perform various image operations under the command line. The following are the steps to install Imagemagick on Centos system: 1
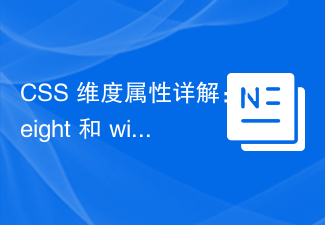
Detailed explanation of CSS dimension properties: height and width In front-end development, CSS is a powerful style definition language. Among them, height and width are the two most basic dimension attributes, used to define the height and width of the element. This article will analyze these two properties in detail and provide specific code examples. 1. Height attribute The height attribute is used to define the height of an element. You can use pixel, percentage or
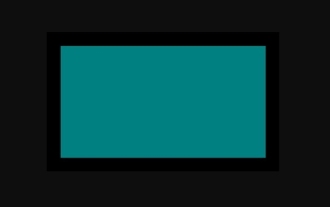
The imagefilledrectangle() function draws a filled rectangle. Syntax imagefilledrectangle($img,$x1,$y1,$x2,$y2,$color) Parameters image Use imagecreatetruecolor() to create a blank image. x1The x coordinate of point 1. y1 The y coordinate of point 1. x2 x coordinate of point 2. y2 The y coordinate of point 2. color fill color. Return value imagefilledrectangle() function returns successfully
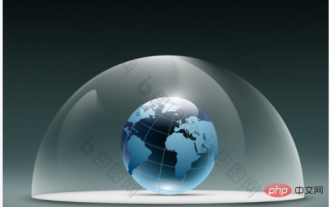
When everyone learns object-oriented Python, they will always encounter an existence that is difficult to understand: self. Who is this self? Why does every class instance method have a parameter self, and what does it do? "Let us draw the conclusion first: after the class is instantiated, self represents the instance (object) itself." I want to understand. The simplest way to use self is to use self as the "ID card of the instance (object)." Python classes cannot be used directly. They can only exert their functions by creating instances (objects). Each instance (object) It is unique in that it can call methods and properties of the class. Classes are like soul possession, allowing instances (objects) to have their own (self) functions. beginner
