


PHP character encoding conversion class, supports ANSI, Unicode, Unicode big endian, UTF-8, UTF-8+Bom mutual conversion
php character encoding conversion class, supports ANSI, Unicode, Unicode big endian, UTF-8, UTF-8+Bom and other conversions.
Four common text file encoding methods
ANSI encoding:
No file header (signature byte at the beginning of file encoding)
ANSI encoding alphanumeric occupies one byte, Chinese characters occupy two bytes
carriage return and line feed, single byte, hexadecimal representation is 0d 0a
UNICODE encoding:
file header, hexadecimal representation is FF FE
Each character is encoded with two bytes
carriage return and line feed character, double bytes, hexadecimal representation is 000d 000a
Unicode big endian encoding:
The hexadecimal representation of the file header is FE FF
The following encoding puts the high bit of the character in the front and the low bit in the back, which is exactly the opposite of the Unicode encoding
The carriage return and line feed character, Double byte, hexadecimal representation is 0d00 0a00
UTF-8 encoding:
File header, hexadecimal representation is EF BB BF
UTF-8 is Unicode A variable-length character encoding. Numbers, letters, carriage returns, and line feeds are all represented by one byte. Chinese characters occupy 3 bytes
carriage return and line feed, single byte, hexadecimal representation is 0d 0a
Conversion principle: First convert the character encoding to UTF-8, and then convert from UTF-8 to the corresponding character encoding.
CharsetConv.class.php
<?php /** 字符编码转换类, ANSI、Unicode、Unicode big endian、UTF-8、UTF-8+Bom互相转换 * Date: 2015-01-28 * Author: fdipzone * Ver: 1.0 * * Func: * public convert 转换 * private convToUtf8 把编码转为UTF-8编码 * private convFromUtf8 把UTF-8编码转换为输出编码 */ class CharsetConv{ // class start private $_in_charset = null; // 源编码 private $_out_charset = null; // 输出编码 private $_allow_charset = array('utf-8', 'utf-8bom', 'ansi', 'unicode', 'unicodebe'); /** 初始化 * @param String $in_charset 源编码 * @param String $out_charset 输出编码 */ public function __construct($in_charset, $out_charset){ $in_charset = strtolower($in_charset); $out_charset = strtolower($out_charset); // 检查源编码 if(in_array($in_charset, $this->_allow_charset)){ $this->_in_charset = $in_charset; } // 检查输出编码 if(in_array($out_charset, $this->_allow_charset)){ $this->_out_charset = $out_charset; } } /** 转换 * @param String $str 要转换的字符串 * @return String 转换后的字符串 */ public function convert($str){ $str = $this->convToUtf8($str); // 先转为utf8 $str = $this->convFromUtf8($str); // 从utf8转为对应的编码 return $str; } /** 把编码转为UTF-8编码 * @param String $str * @return String */ private function convToUtf8($str){ if($this->_in_charset=='utf-8'){ // 编码已经是utf-8,不用转 return $str; } switch($this->_in_charset){ case 'utf-8bom': $str = substr($str, 3); break; case 'ansi': $str = iconv('GBK', 'UTF-8//IGNORE', $str); break; case 'unicode': $str = iconv('UTF-16le', 'UTF-8//IGNORE', substr($str, 2)); break; case 'unicodebe': $str = iconv('UTF-16be', 'UTF-8//IGNORE', substr($str, 2)); break; default: break; } return $str; } /** 把UTF-8编码转换为输出编码 * @param String $str * @return String */ private function convFromUtf8($str){ if($this->_out_charset=='utf-8'){ // 输出编码已经是utf-8,不用转 return $str; } switch($this->_out_charset){ case 'utf-8bom': $str = "\xef\xbb\xbf".$str; break; case 'ansi': $str = iconv('UTF-8', 'GBK//IGNORE', $str); break; case 'unicode': $str = "\xff\xfe".iconv('UTF-8', 'UTF-16le//IGNORE', $str); break; case 'unicodebe': $str = "\xfe\xff".iconv('UTF-8', 'UTF-16be//IGNORE', $str); break; default: break; } return $str; } } // class end ?>
demo: unicode big endian converted to utf-8+bom
<?php require "CharsetConv.class.php"; $str = file_get_contents('source/unicodebe.txt'); $obj = new CharsetConv('unicodebe', 'utf-8bom'); $response = $obj->convert($str); file_put_contents('response/utf-8bom.txt', $response, true); ?>
Source code download address: Click to view
The above introduces the PHP character encoding conversion class, which supports ANSI, Unicode, Unicode big endian, UTF-8, UTF-8+Bom and other conversions, including relevant content. I hope it will be helpful to friends who are interested in PHP tutorials.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Unicode is a character encoding standard used to represent various languages and symbols. To convert Unicode encoding to Chinese characters, you can use Python's built-in functions chr() and ord().
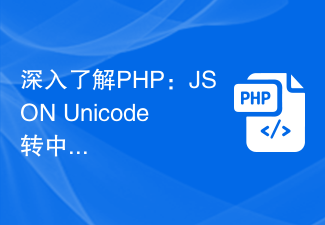
In-depth understanding of PHP: Implementation method of converting JSONUnicode to Chinese During development, we often encounter situations where we need to process JSON data, and Unicode encoding in JSON will cause us some problems in some scenarios, especially when Unicode needs to be converted When encoding is converted to Chinese characters. In PHP, there are some methods that can help us achieve this conversion process. A common method will be introduced below and specific code examples will be provided. First, let us first understand the Un in JSON
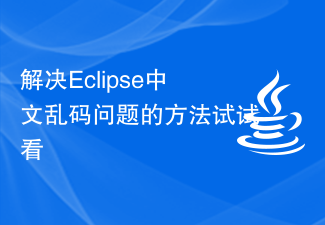
Are you troubled by Chinese garbled characters in Eclipse? To try these solutions, you need specific code examples 1. Background introduction With the continuous development of computer technology, Chinese plays an increasingly important role in software development. However, many developers encounter garbled code problems when using Eclipse for Chinese development, which affects work efficiency. Then, this article will introduce some common garbled code problems and give corresponding solutions and code examples to help readers solve the Chinese garbled code problem in Eclipse. 2. Common garbled code problems and solution files
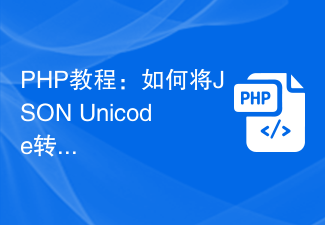
JSON (JavaScriptObjectNotation) is a lightweight data exchange format commonly used for data exchange between web applications. When processing JSON data, we often encounter Unicode-encoded Chinese characters (such as "u4e2du6587") and need to convert them into readable Chinese characters. In PHP, we can achieve this conversion through some simple methods. Next, we will detail how to convert JSONUnico
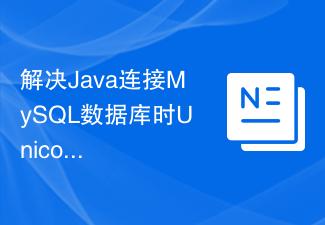
With the development of technologies such as big data and cloud computing, databases have become one of the important cornerstones of enterprise informatization. In applications developed in Java, connecting to MySQL database has become the norm. However, in this process, we often encounter a thorny problem - inconsistent Unicode character set encoding. This will not only affect our development efficiency, but also affect the performance and stability of the application. This article will introduce how to solve this problem and make Java connect to the MySQL database more smoothly. 1. Unicode

The differences between unicode and ascii include different encoding ranges, different storage spaces, and different compatibility. Detailed introduction: 1. The encoding range is different. The encoding range of ASCII is 0-127, which is mainly used to represent English letters. The encoding range of Unicode is much wider and can represent almost all language characters; 2. The storage space is different. ASCII usually Use 1 byte to store a character, while unicode may use 2 or more bytes to store a character; 3. Different compatibility, etc.
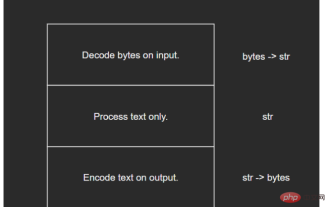
1The basic unit of Unicode computer storage is the byte, which is composed of 8 bits. Since English only consists of 26 letters plus a number of symbols, English characters can be stored directly in bytes. But other languages (such as Chinese, Japanese, Korean, etc.) have to use multiple bytes for encoding due to the large number of characters. With the spread of computer technology, non-Latin character encoding technology continues to develop, but there are still two major limitations: no multi-language support: the encoding scheme of one language cannot be used in another language and there is no unified standard: for example There are many encoding standards in Chinese such as GBK, GB2312, GB18030, etc. Since the encoding methods are not unified, developers need to convert back and forth between different encodings, and many errors will inevitably occur.
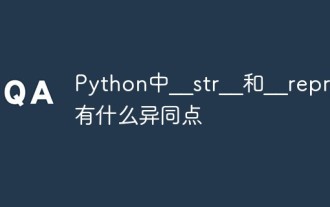
What are the similarities and differences between __str__ and __repr__? We all know the representation of strings. Python's built-in function repr() can express objects in the form of strings to facilitate our identification. This is the "string representation". repr() obtains the string representation of an object through the special method __repr__. If __repr__ is not implemented, when we print an instance of a vector to the console, the resulting string may be. >>>classExample:pass>>>print(str(Example()))>>>
