laravel - php variable parsing
Aug 18, 2016 am 09:15 AM
For example, now I have the variable $arr, which is an array
<code>$arr = [ 'news' => [ 'data' => [ 0 => [ 'title' => '名字', 'content' => '内容' ], ], ], ]; </code>
Some frameworks or template engines have parsing functions. The value of title
can be obtained through arr.news.data[0].title
, and the value can be modified.
Then I want to know what its principle is, and how to use this expression efficiently, safely and simply to get
and set
the values in the array?
What I can think of is to use text processing, but it should not be very safe and efficient. Ask the teacher for guidance.
Reply content:
For example, now I have the variable $arr, which is an array
<code>$arr = [ 'news' => [ 'data' => [ 0 => [ 'title' => '名字', 'content' => '内容' ], ], ], ]; </code>
Some frameworks or template engines have parsing functions. The value of title
can be obtained through arr.news.data[0].title
, and the value can be modified.
Then I want to know what its principle is, and how to use this expression efficiently, safely and simply to get
and set
the values in the array?
What I can think of is to use text processing, but it should not be very safe and efficient. Ask the teacher for guidance.
Most template engines are processed in a pre-compiled manner, that is, the input template data will be converted into standard PHP statements such as variables, loops, conditions and other symbols, and then executed.
In addition, these frameworks or template engines are all open source. If you have time to ask people here, you will already understand if you look at the code yourself.
Let me find you a code.
<code> public static function getValue($array, $key, $default = null) { if ($key instanceof \Closure) { return $key($array, $default); } if (is_array($key)) { $lastKey = array_pop($key); foreach ($key as $keyPart) { $array = static::getValue($array, $keyPart); } $key = $lastKey; } if (is_array($array) && (isset($array[$key]) || array_key_exists($key, $array)) ) { return $array[$key]; } if (($pos = strrpos($key, '.')) !== false) { $array = static::getValue($array, substr($key, 0, $pos), $default); $key = substr($key, $pos + 1); } if (is_object($array)) { // this is expected to fail if the property does not exist, or __get() is not implemented // it is not reliably possible to check whether a property is accessable beforehand return $array->$key; } elseif (is_array($array)) { return (isset($array[$key]) || array_key_exists($key, $array)) ? $array[$key] : $default; } else { return $default; } }</code>

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
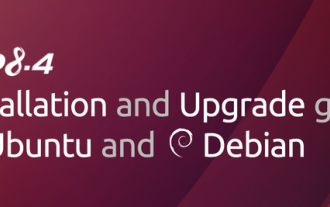
PHP 8.4 Installation and Upgrade guide for Ubuntu and Debian
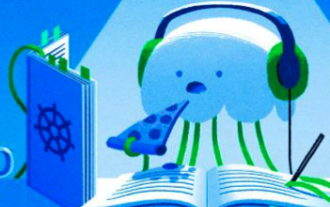
How To Set Up Visual Studio Code (VS Code) for PHP Development
