


When uploading pictures, org.apache.http.client.HttpResponseException: Not Foun will be reported.
The above error will be reported as soon as the picture is uploaded. It is wrong, but the picture has an address. The URL address is correct, but there is a picture with an address, but it cannot be uploaded. The breakpoint returns 404.
Reply content:
The above error will be reported as soon as the picture is uploaded. It is wrong, but the picture has an address. The URL address is correct, but there is a picture with an address, but it cannot be uploaded. The breakpoint returns 404.
404 indicates that there is a client problem that causes the server to be unable to process, https://segmentfault.com/a/11...
It is recommended to deprecate httpclient and use HttpURLConnection:
<code>import java.io.ByteArrayOutputStream; import java.io.DataOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.net.HttpURLConnection; import java.net.URL; import java.util.HashMap; import java.util.List; import java.util.Map; public class HttpConnector { public static final int TIMEOUT_MS = 10 * 1000; public static String performRequest(String url, File file, Map<String, String> additionalHeaders) { String response = null; try { HashMap<String, String> map = new HashMap<String, String>(); if (additionalHeaders != null) map.putAll(additionalHeaders); URL parsedUrl = new URL(url); HttpURLConnection connection = openConnection(parsedUrl, file); for (String headerName : map.keySet()) { connection.addRequestProperty(headerName, map.get(headerName)); } int responseCode = connection.getResponseCode(); if (responseCode == -1) { // -1 is returned by getResponseCode() if the response code could not be retrieved. // Signal to the caller that something was wrong with the connection. throw new IOException("Could not retrieve response code from HttpUrlConnection."); } String charset = "utf-8"; String contentEncoding = null; String contentType = null; for (Map.Entry<String, List<String>> header : connection.getHeaderFields().entrySet()) { if (header.getKey() != null) { List<String> headerValueList = header.getValue(); if (headerValueList != null && headerValueList.size() > 0) { StringBuffer headerValueSB = new StringBuffer(); for (int i = 0; i < headerValueList.size(); i++) { headerValueSB.append(headerValueList.get(i)); if (i != headerValueList.size() - 1) { headerValueSB.append(";"); } } if (header.getKey().equals("Content-Type")) { contentType = headerValueSB.toString(); } else if (header.getKey().equals("Content-Encoding")) { contentEncoding = headerValueSB.toString(); } } } } charset = parseCharset(contentType, contentEncoding, charset); if (responseCode == 200) { //成功 response = entityFromConnection(connection, charset); } } catch (Exception e) { // e.printStackTrace(); } return response; } /** * Create an {@link HttpURLConnection} for the specified {@code url}. */ protected static HttpURLConnection createConnection(URL url) throws IOException { return (HttpURLConnection) url.openConnection(); } /** * Opens an {@link HttpURLConnection} with parameters. * * @param url * @return an open connection * @throws IOException */ private static HttpURLConnection openConnection(URL url, File file) throws IOException { HttpURLConnection connection = createConnection(url); connection.setConnectTimeout(TIMEOUT_MS); connection.setReadTimeout(TIMEOUT_MS); connection.setUseCaches(false); connection.setDoInput(true); connection.setRequestMethod("POST"); addBodyIfExists(connection, file); return connection; } private static String parseCharset(String contentType, String contentEncoding, String defaultCharset) { if (contentType != null) { String[] params = contentType.split(";"); for (int i = 1; i < params.length; i++) { String[] pair = params[i].trim().split("="); if (pair.length == 2) { if (pair[0].equals("charset")) { return pair[1]; } } } } if (contentEncoding != null && !contentEncoding.equals("")) { return contentEncoding; } return defaultCharset; } private static String entityFromConnection(HttpURLConnection connection, String charset) throws IOException { String data = null; InputStream inputStream; try { inputStream = connection.getInputStream(); } catch (IOException ioe) { inputStream = null; } if (inputStream != null) { data = readData(inputStream, charset); } return data; } private static String readData(InputStream inSream, String charsetName) throws IOException { ByteArrayOutputStream outStream = new ByteArrayOutputStream(); byte[] buffer = new byte[1024]; int len = -1; while ((len = inSream.read(buffer)) != -1) { outStream.write(buffer, 0, len); } byte[] data = outStream.toByteArray(); outStream.close(); inSream.close(); return new String(data, charsetName); } private static void addBodyIfExists(HttpURLConnection connection, File file) { try { if (file != null) { connection.setDoOutput(true); connection.addRequestProperty("Content-Type", "application/octet-stream; charset=utf-8"); DataOutputStream out = new DataOutputStream(connection.getOutputStream()); FileInputStream fStream = new FileInputStream(file); /* 设定每次写入1024bytes */ try { int bufferSize = 1024; byte[] buffer = new byte[bufferSize]; int length = -1; /* 从文件读取数据到缓冲区 */ while ((length = fStream.read(buffer)) != -1) { /* 将数据写入DataOutputStream中 */ out.write(buffer, 0, length); } } finally { fStream.close(); out.flush(); out.close(); } } } catch (IOException e) { e.printStackTrace(); } } }</code>
Call: String response = HttpConnector.performRequest(uploadUrl, file, null);

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
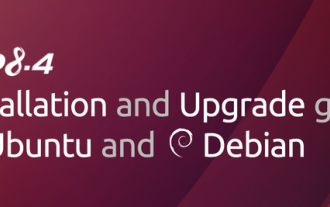
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
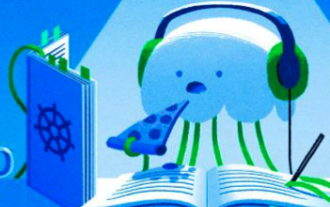
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
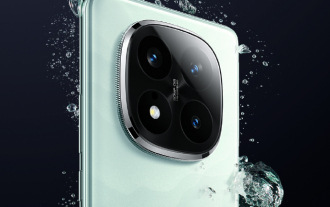
The Redmi Note 14 Pro Plus is now official as a direct successor to last year'sRedmi Note 13 Pro Plus(curr. $375 on Amazon). As expected, the Redmi Note 14 Pro Plus heads up the Redmi Note 14 series alongside theRedmi Note 14and Redmi Note 14 Pro. Li
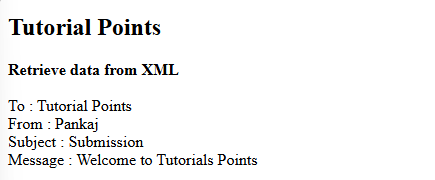
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
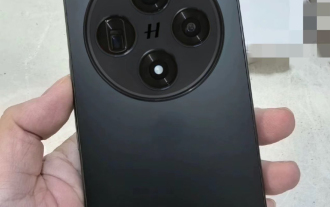
Historically, Oppo has refreshed its flagship 'Find X' series in late winter or early spring, save for the original Find X that it announced in June 2018. To that end, the Find X7 and Find X7 Ultra are barely more than six months old at this point. H
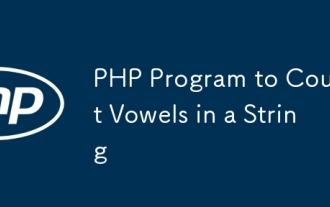
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
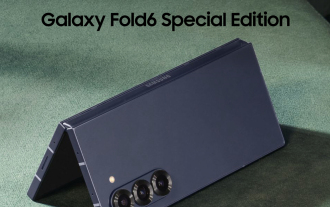
The launch of Samsung's long-awaited 'Special Edition' foldable has taken another twist. In recent weeks, rumours about the so-called Galaxy Z Fold Special Edition went rather quiet. Instead, the focus has shifted to the Galaxy S25 series, including
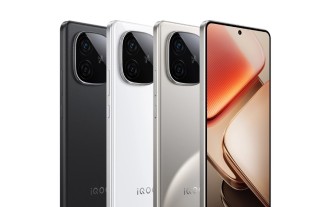
TheZ9 Turbo+has now been unleashed on Vivo's online Chinese store at 2,199 yuan (~$313) for a 12GB RAM/256GB internal storage base model, whereas theRedmiK70 Extreme Editionstarted at 2,599 yuan (~$370) with the same configuration: in fact, its newiQ
