PHP infinite classification code problem
I tried to write an infinite classification, but there were some problems. After thinking about it for a long time, I felt that the code was fine, but the result was incorrect. Now everyone is confused....
After reading some chestnuts online, it seems that the writing method is different from mine.
The array structure is as follows:
<code>Array ( [0] => Array ( [id] => 1 [name] => 代码 [parent] => 0 ) [1] => Array ( [id] => 3 [name] => 动漫 [parent] => 0 ) [2] => Array ( [id] => 4 [name] => 治愈 [parent] => 3 ) [3] => Array ( [id] => 5 [name] => 励志 [parent] => 3 ) [4] => Array ( [id] => 6 [name] => 机战 [parent] => 3 ) [5] => Array ( [id] => 7 [name] => 百合 [parent] => 3 ) [6] => Array ( [id] => 8 [name] => 资源 [parent] => 0 ) [7] => Array ( [id] => 9 [name] => app [parent] => 8 ) [8] => Array ( [id] => 10 [name] => 软件 [parent] => 8 ) [9] => Array ( [id] => 11 [name] => 黑科技 [parent] => 8 ) )</code>
Among them id
is the unique ID of the category, parent
is the parent class ID
The code I wrote is as follows:
<code> function all($id=0){ static $_class = null; if(is_null($_class)) $_class = select(); //这个得出来的就是以上的数组结构,然后赋值给了`$_class`变量 $result = array(); foreach($_class as $k => $v){ if($v['parent'] == $id){ unset($_class[$k]); $v = array_merge($v, $this->all($v['id'])); $result['child'][] = $v; } } return $result; } print_r(all(0));</code>
Reply content:
I tried to write an infinite classification, but there were some problems. After thinking about it for a long time, I felt that the code was fine, but the result was incorrect. Now everyone is confused....
After reading some chestnuts online, it seems that the writing method is different from mine.
The array structure is as follows:
<code>Array ( [0] => Array ( [id] => 1 [name] => 代码 [parent] => 0 ) [1] => Array ( [id] => 3 [name] => 动漫 [parent] => 0 ) [2] => Array ( [id] => 4 [name] => 治愈 [parent] => 3 ) [3] => Array ( [id] => 5 [name] => 励志 [parent] => 3 ) [4] => Array ( [id] => 6 [name] => 机战 [parent] => 3 ) [5] => Array ( [id] => 7 [name] => 百合 [parent] => 3 ) [6] => Array ( [id] => 8 [name] => 资源 [parent] => 0 ) [7] => Array ( [id] => 9 [name] => app [parent] => 8 ) [8] => Array ( [id] => 10 [name] => 软件 [parent] => 8 ) [9] => Array ( [id] => 11 [name] => 黑科技 [parent] => 8 ) )</code>
Among them id
is the unique ID of the category, parent
is the parent class ID
The code I wrote is as follows:
<code> function all($id=0){ static $_class = null; if(is_null($_class)) $_class = select(); //这个得出来的就是以上的数组结构,然后赋值给了`$_class`变量 $result = array(); foreach($_class as $k => $v){ if($v['parent'] == $id){ unset($_class[$k]); $v = array_merge($v, $this->all($v['id'])); $result['child'][] = $v; } } return $result; } print_r(all(0));</code>
unset($_class[$k]);
Remove this line
Since you still use recursion to complete this, I provide 2 methods here, one is recursive and the other is in pointer form.
You may not be able to understand the second one, but to help you learn and make it easier for others, here is the code:
Use recursion
<code>// 呃,我真不忍心写出这个循环那么多遍的代码,求神解救我。 function _data_to_tree(&$items, $topid = 0, $with_id = TRUE) { $result = []; foreach($items as $v) if ($topid == $v['parent']) { $r = $v + ['children' => _data_to_tree($items, $v['id'], $with_id)]; if ($with_id) $result[$v['id']] = $r; else $result[] = $r; } return $result; } </code>
Use PHP’s pointer features
<code>function _data_to_tree($items, $topid = 0, $with_id = TRUE) { if ($with_id) foreach ($items as $item) $items[ $item['parent'] ]['children'][ $item['id'] ] = &$items[ $item['id'] ]; else foreach ($items as $item) $items[ $item['parent'] ]['children'][] = &$items[ $item['id'] ]; return isset($items[ $topid ]['children']) ? $items[ $topid ][ 'children' ] : []; }</code>
Use
Pass in your above array, for example, the topmost ID is 0
<code>$data = [ ['id' => 4, 'parent' => 1 , 'text' => 'Parent1'], ['id' => 1, 'parent' => 0 , 'text' => 'Root'], ['id' => 2, 'parent' => 1 , 'text' => 'Parent2'], ['id' => 3, 'parent' => 2 , 'text' => 'Sub1'], ]; print_r ( _data_to_tree($data, 0) ); </code>
Results
<code>Array ( [1] => Array ( [id] => 1 [parent] => 0 [text] => Root [children] => Array ( [4] => Array ( [id] => 4 [parent] => 1 [text] => Parent1 [children] => Array ( ) ) [2] => Array ( [id] => 2 [parent] => 1 [text] => Parent2 [children] => Array ( [3] => Array ( [id] => 3 [parent] => 2 [text] => Sub1 [children] => Array ( ) ) ) ) ) ) )</code>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
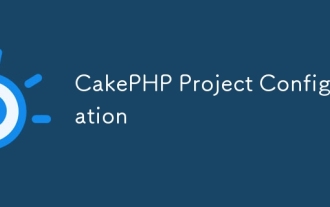
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
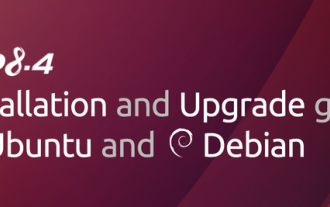
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
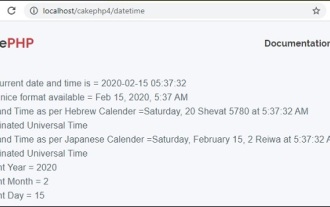
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
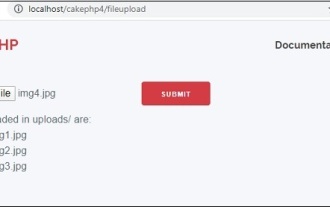
To work on file upload we are going to use the form helper. Here, is an example for file upload.
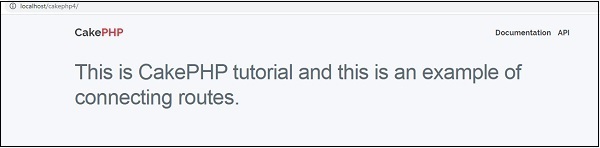
In this chapter, we are going to learn the following topics related to routing ?
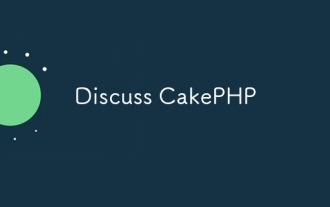
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
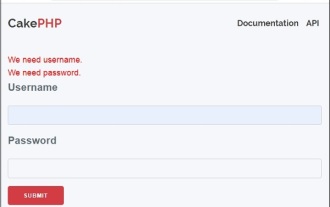
Validator can be created by adding the following two lines in the controller.
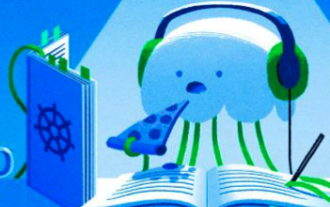
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
