Some easily confused methods in js
There are some very long function names in JavaScript, which can cause confusion when using them. I would like to sort them out to deepen my impression.
①Object.getOwnPropertyDescriptor - Read the property descriptor of a specific property of an object (value / writable / enumerable / configurable)
This method accepts two parameters: (the object where the property is located, the property name of its descriptor to be read ), the return value is an object.
var o = Object.getOwnPropertyDescriptor({x : 1}, 'x'); //{value:1, writable:true, enumerable:true, configurable:true}alert(o); // [object Object]
This method can only obtain its own property descriptor, and cannot obtain the characteristics of inherited properties:
var o = Object.getOwnPropertyDescriptor({}, 'toString');<br> alert(o); //undefined
②Object.defineProperty - Set the characteristics of an object (single) property or let the new property have certain characteristics
This The method accepts three parameters: (the object to be modified, the attribute to be created or modified, and the attribute descriptor object).
var o = {}; Object.defineProperty(o, 'x', { value : 1, writable : true, enumerable : false, configurable : true }); alert(o.x); // 1 Object.defineProperty(o, 'x', {writable : false}); o.x = 2; //不可行,不会报错,但不会修改, o.x = 1; Object.defineProperty(o, 'x', {value : 2}); alert(o.x); // 2
③Object.defineProperties - Set the characteristics of an object (multiple) properties or let the new properties have certain characteristics.
This method accepts two parameters: (modified object, mapping table-including all new or modified properties) property name and property descriptor).
Object.defineProperties({}, { _year : { value : 2016, writable : true, enumerable : true, configurable : true }, edition : { value : 1 }, year : { get : function(){ return this._year; }, set : function(newValue){ if(newValue > 2004){ this._year = newValue; this.edition += newValue - 2004; } } } });
The above defines two data attributes (_year and edition) and an accessor attribute (year) in an empty object, where the two attributes are all created at the same time.
④isPrototypeOf - Determine the relationship between the object and the prototype
function Person(){} var friend = new Person(); alert(Person.prototype.isPrototypeOf(friend)); //true
Because there is a pointer to Person.prototype inside the friend object, it returns true.
⑤Object.getPrototypeOf - Conveniently get the prototype of an object
function Person(){} Person.prototype.name = 'Tom'; var friend = new Person(); alert(Object.getPrototypeOf(friend) == Person.prototype); // true alert(Object.getPrototypeOf(friend).name); // Tom
A new method in ES5, supported by IE9+.
⑥hasOwnProperty - Detect whether a property exists in an instance or a prototype
function Person(){} Person.prototype.name = 'Tom'; Person.prototype.sayName = function(){ alert(this.name); }; var frient1 = new Person(); frient1.name = 'Jery'; var frient2 = new Person(); alert(frient1.hasOwnProperty('name')); alert(frient2.hasOwnProperty('name'));
Using hasOwnProperty() you can easily know whether you are accessing an instance property or a prototype property.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


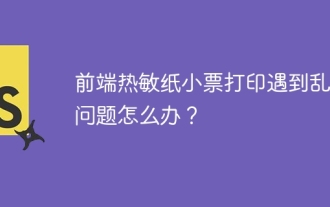
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
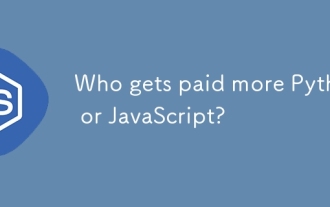
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
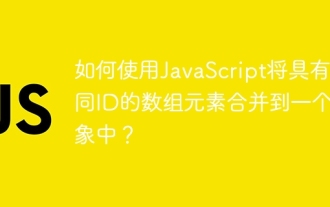
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
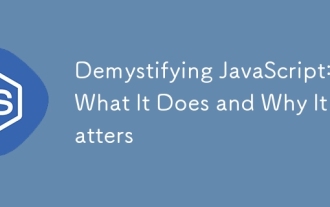
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
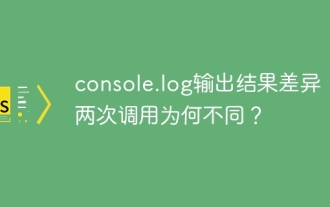
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
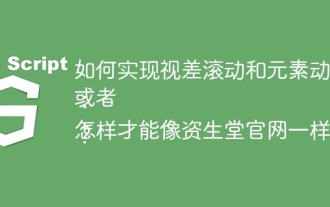
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
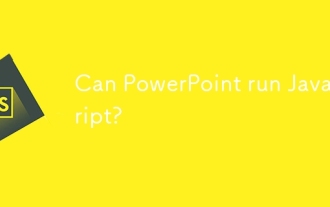
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
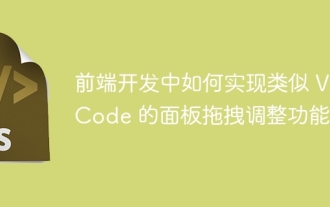
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
