Python FTP transfer
Accessing FTP is nothing more than two things: upload and download. Recently, I need to download a large number of files from ftp in the project, and then I tried to experiment with my own ftp operation class, as follows (PS: There is something wrong with this paragraph, please do not copy and use it. You can refer to it to test your own ftp class)
import os from ftplib import FTP class FTPSync(): def __init__(self, host, usr, psw, log_file): self.host = host self.usr = usr self.psw = psw self.log_file = log_file def __ConnectServer(self): try: self.ftp = FTP(self.host) self.ftp.login(self.usr, self.psw) self.ftp.set_pasv(False) return True except Exception: return False def __CloseServer(self): try: self.ftp.quit() return True except Exception: return False def __CheckSizeEqual(self, remoteFile, localFile): try: remoteFileSize = self.ftp.size(remoteFile) localFileSize = os.path.getsize(localFile) if localFileSize == remoteFileSize: return True else: return False except Exception: return None def __DownloadFile(self, remoteFile, localFile): try: self.ftp.cwd(os.path.dirname(remoteFile)) f = open(localFile, 'wb') remoteFileName = 'RETR ' + os.path.basename(remoteFile) self.ftp.retrbinary(remoteFileName, f.write) if self.__CheckSizeEqual(remoteFile, localFile): self.log_file.write('The File is downloaded successfully to %s' + '\n' % localFile) return True else: self.log_file.write('The localFile %s size is not same with the remoteFile' + '\n' %localFile) return False except Exception: return False def __DownloadFolder(self, remoteFolder, localFolder): try: fileList = [] self.ftp.retrlines('NLST', fileList.append) for remoteFile in fileList: localFile = os.path.join(localFolder, remoteFile) return self.__DownloadFile(remoteFile, localFile) except Exception: return False def SyncFromFTP(self, remoteFolder, localFolder): self.__DownloadFolder(remoteFolder, localFolder) self.log_file.close() self.__CloseServer()

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


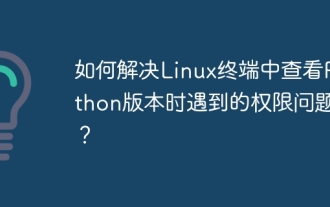
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
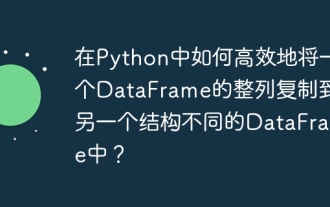
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
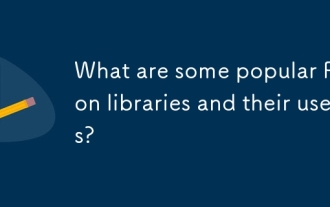
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
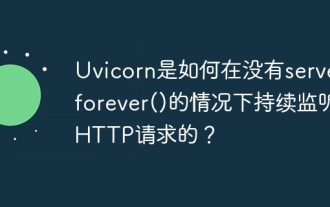
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
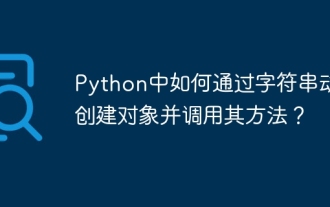
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
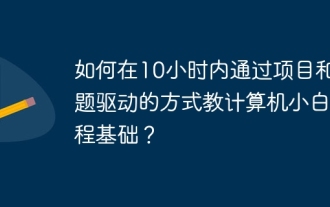
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
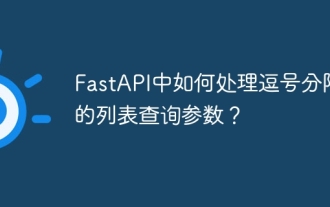
Fastapi ...
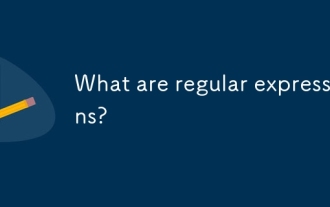
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
