How to determine sim card status and read contact information in android
When writing a program, sometimes you may need to obtain some contact information in the SIM card. Before obtaining the SIM card contacts, we usually determine the status of the SIM card first, and then obtain its information after finding the SIM card. With the following code, we can read some information of the contacts in the SIM card.
import android.app.Activity; import android.content.Context; import android.content.Intent; import android.database.Cursor; import android.net.Uri; import android.os.Bundle; import android.telephony.TelephonyManager; import android.widget.TextView; public class PhoneTest extends Activity { private TextView mTextView; protected Cursor mCursor = null; private TelephonyManager mTelephonyManager; private String mString = ""; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); mTextView = (TextView)findViewById(R.id.text); mTextView.setTextSize(20.3f); isSimExist(); if(getSimState() == TelephonyManager.SIM_STATE_READY){ mString += " 卡存在\n"; getSimContacts("content://icc/adn"); //一般用这一条,如果这条不行的话可以试试下面的一条。 getSimContacts("content://sim/adn");//此种读法在我们手机里不能读取,所以,还是用上个uri比较好。 } mTextView.setText(mString); } private void getSimContacts(String str){ Intent intent = new Intent(); intent.setData(Uri.parse(str)); Uri uri = intent.getData(); mCursor = getContentResolver().query(uri, null, null, null, null); if(mCursor == null){ mString += "不能从" + str + "读数据\n"; return ; } mString += "第一列:" + mCursor.getColumnName(0) + "\n"; mString += "第二列:" + mCursor.getColumnName(1) + "\n"; mString += "第三列:" + mCursor.getColumnName(2) + "\n"; mString += "第四列:" + mCursor.getColumnName(3) + "\n"; mString += "列数:" + mCursor.getColumnCount() + "\n"; mString += "行数:" + mCursor.getCount() + "\n"; if (mCursor != null) { while (mCursor.moveToNext()) { // 取得联系人名字 int nameFieldColumnIndex = mCursor.getColumnIndex("name"); mString += mCursor.getString(nameFieldColumnIndex)+" "; // 取得电话号码 int numberFieldColumnIndex = mCursor .getColumnIndex("number"); mString += mCursor.getString(numberFieldColumnIndex)+" "; // 取得邮箱 int emailsFieldColumnIndex = mCursor .getColumnIndex("emails"); mString += mCursor.getString(emailsFieldColumnIndex)+" "; // 取得id int idFieldColumnIndex = mCursor .getColumnIndex("_id"); mString += mCursor.getString(idFieldColumnIndex)+"\n"; } } mString += mCursor + "\n"; mCursor.close(); } private int getSimState(){ return mTelephonyManager.getSimState(); } private void isSimExist(){ mTelephonyManager = (TelephonyManager) getSystemService(Context.TELEPHONY_SERVICE); int simState = mTelephonyManager.getSimState(); switch (simState) { case TelephonyManager.SIM_STATE_ABSENT: mString = "无卡"; // do something break; case TelephonyManager.SIM_STATE_NETWORK_LOCKED: mString = "需要NetworkPIN解锁"; // do something break; case TelephonyManager.SIM_STATE_PIN_REQUIRED: mString = "需要PIN解锁"; // do something break; case TelephonyManager.SIM_STATE_PUK_REQUIRED: mString = "需要PUN解锁"; // do something break; case TelephonyManager.SIM_STATE_READY: mString = "良好"; // do something break; case TelephonyManager.SIM_STATE_UNKNOWN: mString = "未知状态"; // do something break; } mTextView.setText(mString); } }

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
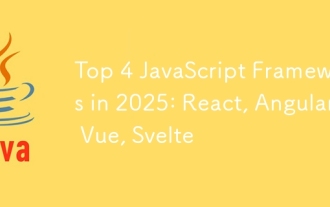
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
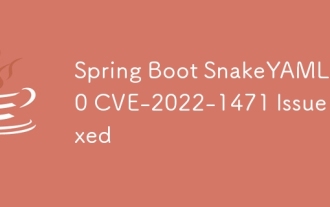
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
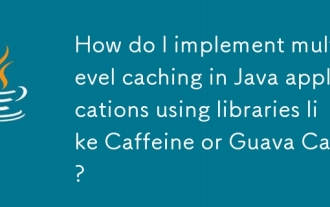
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
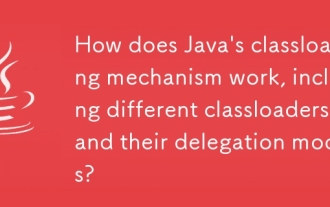
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
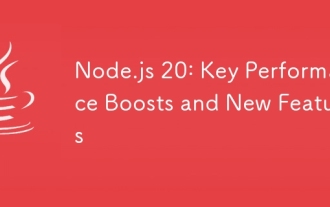
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
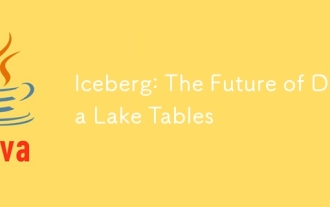
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
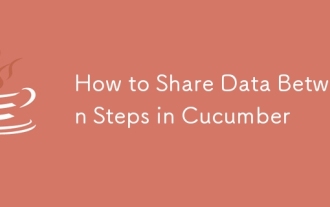
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
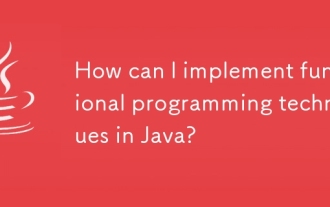
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
