The difference between rewriting and overloading methods in java
Overloading: The method names are the same, but the parameters must be different (different parameters can result in different types, different orders, and different numbers). Overriding (also called overwriting): The subclass inherits the method of the parent class and reimplements the method.
Precautions for using method rewriting:
1. When rewriting a method, there must be an inheritance relationship
2. When rewriting a method, the method name and formal parameters must be consistent.
3. When rewriting a method, the permission modifier of the subclass needs to be greater than or equal to the permission modifier of the parent class.
4. When rewriting a method, the return value type of the subclass must be less than or equal to the return value type of the parent class
5. When rewriting a method, the exception type of the subclass must be less than or equal to the exception type of the parent class.
Here we mainly use the third point to test:
public class a{ public static class People { public void fun(){}; } static class Student extends People { protected void fun(){ System.out.println("dfdfd"); } } public static void main(String[] args){ Student p = new Student(); p.fun(); } }
An error is reported when compiling, as shown in the picture:
The reason is that the permissions of the methods of the parent class are public, and the subclasses are changed to protected, which reduces permission, so it can only be greater than or equal to:
public class a{ public static class People { protected void fun(){}; } static class Student extends People { public void fun(){ System.out.println("dfdfd"); } } public static void main(String[] args){ Student p = new Student(); p.fun(); } }
Run successfully

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


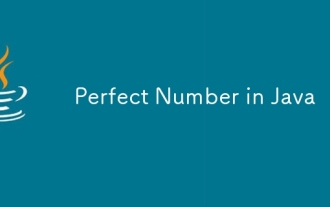
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
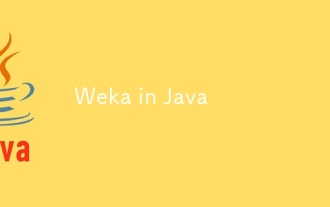
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
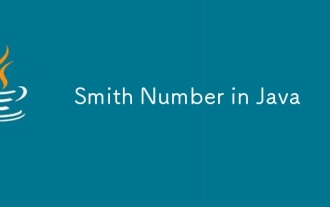
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
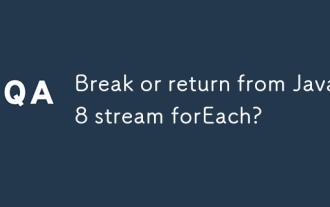
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
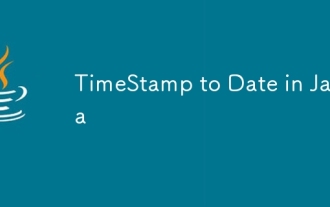
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
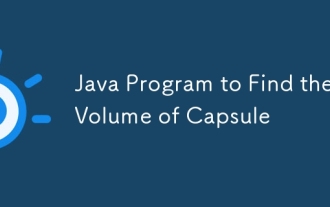
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
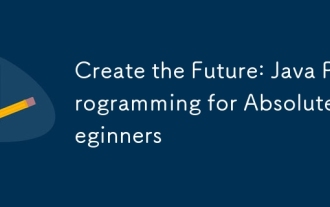
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
