


A new way to create javascript objects Object.create()_javascript skills
What is Object.create()?
Object.create(proto [, propertiesObject ]) is a new object creation method proposed in E5. The first parameter is the prototype to be inherited. If it is not a sub-function, you can pass a null. The second parameter is The parameter is the attribute descriptor of the object. This parameter is optional.
For example:
function Car (desc) { this.desc = desc; this.color = "red"; } Car.prototype = { getInfo: function() { return 'A ' + this.color + ' ' + this.desc + '.'; } }; //instantiate object using the constructor function var car = Object.create(Car.prototype); car.color = "blue"; alert(car.getInfo());
The result is:A blue undefined.
1. Detailed explanation of propertiesObject parameters: (the default is false)
Data attributes:
- writable: whether it can be written arbitrarily
- configurable: whether it can be deleted and whether it can be modified
- enumerable: whether it can be enumerated using for in
- value: value
Access properties:
- get(): access
- set(): Set
2. Example: Just look at the example to know how to use it.
<!DOCTYPE html> <html> <head> <title>yupeng's document </title> <meta charset="utf-8"/> </head> <body> <script type="text/javascript"> var obj = { a:function(){ console.log(100) }, b:function(){ console.log(200) }, c:function(){ console.log(300) } } var newObj = {}; newObj = Object.create(obj,{ t1:{ value:'yupeng', writable:true }, bar: { configurable: false, get: function() { return bar; }, set: function(value) { bar=value } } }) console.log(newObj.a()); console.log(newObj.t1); newObj.t1='yupeng1' console.log(newObj.t1); newObj.bar=201; console.log(newObj.bar) function Parent() { } var parent = new Parent(); var child = Object.create(parent, { dataDescriptor: { value: "This property uses this string as its value.", writable: true, enumerable: true }, accessorDescriptor: { get: function () { return "I am returning: " + accessorDescriptor; }, set: function (val) { accessorDescriptor = val; }, configurable: true } }); child.accessorDescriptor = 'YUPENG'; console.log(child.accessorDescriptor); var Car2 = function(){ this.name = 'aaaaaa' } //this is an empty object, like {} Car2.prototype = { getInfo: function() { return 'A ' + this.color + ' ' + this.desc + '.'; } }; var newCar = new Car2(); var car2 = Object.create(newCar, { //value properties color: { writable: true, configurable:true, value: 'red' }, //concrete desc value rawDesc: { writable: true, configurable:true, value: 'Porsche boxter' }, // data properties (assigned using getters and setters) desc: { configurable:true, get: function () { return this.rawDesc.toUpperCase(); }, set: function (value) { this.rawDesc = value.toLowerCase(); } } }); car2.color = 'blue'; console.log(car2.getInfo()); car2.desc = "XXXXXXXX"; console.log(car2.getInfo()); console.log(car2.name); </script> </body> </html>
The result is:
100
yupeng
yupeng1
201
I am returning: YUPENG
A blue PORSCHE BOXTER.
A blue XXXXXXXX.
aaaaaa
The above is a detailed introduction to Object.create(), a new object creation method in JavaScript. I hope it will be helpful to everyone's learning.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
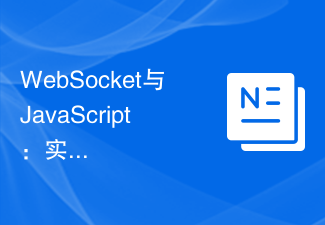
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
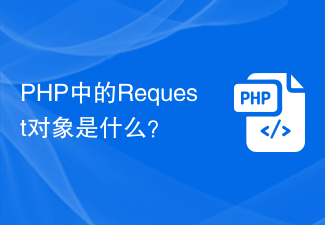
The Request object in PHP is an object used to handle HTTP requests sent by the client to the server. Through the Request object, we can obtain the client's request information, such as request method, request header information, request parameters, etc., so as to process and respond to the request. In PHP, you can use global variables such as $_REQUEST, $_GET, $_POST, etc. to obtain requested information, but these variables are not objects, but arrays. In order to process request information more flexibly and conveniently, you can
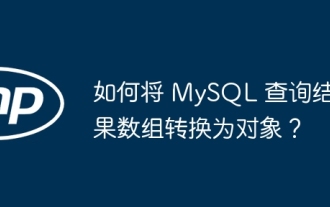
Here's how to convert a MySQL query result array into an object: Create an empty object array. Loop through the resulting array and create a new object for each row. Use a foreach loop to assign the key-value pairs of each row to the corresponding properties of the new object. Adds a new object to the object array. Close the database connection.
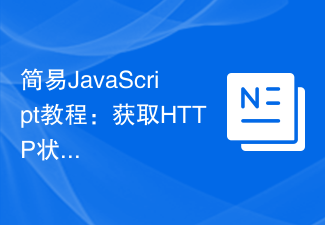
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
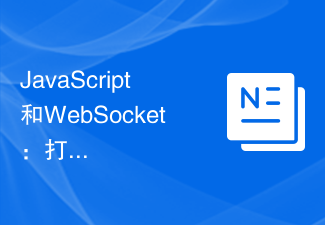
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
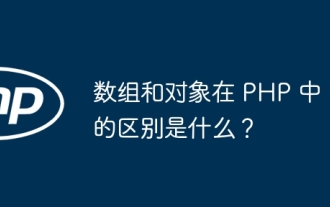
In PHP, an array is an ordered sequence, and elements are accessed by index; an object is an entity with properties and methods, created through the new keyword. Array access is via index, object access is via properties/methods. Array values are passed and object references are passed.
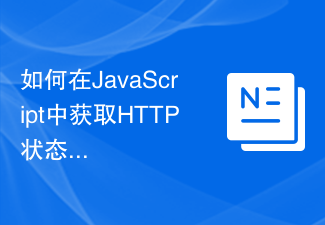
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
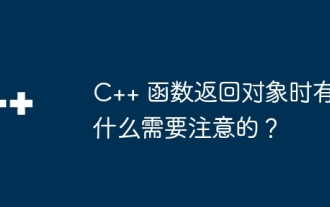
In C++, there are three points to note when a function returns an object: The life cycle of the object is managed by the caller to prevent memory leaks. Avoid dangling pointers and ensure the object remains valid after the function returns by dynamically allocating memory or returning the object itself. The compiler may optimize copy generation of the returned object to improve performance, but if the object is passed by value semantics, no copy generation is required.
