php type constraints
PHP 5 can use type constraints. Function parameters can be specified as objects (specify the class name in the function prototype), interfaces, arrays (from PHP 5.1) or callable (from PHP 5.4). However, if you use NULL as the default value of the parameter, you can still use NULL as the actual parameter when calling the function.
If a class or interface specifies a type constraint, so do all its subclasses or implementations.
Type constraints cannot be used for scalar types such as int or string. Traits are not allowed either.
Example #1 Type constraint example
<?php //如下面的类 class MyClass { /** * 测试函数 * 第一个参数必须为 OtherClass 类的一个对象 */ public function test(OtherClass $otherclass) { echo $otherclass->var; } /** * 另一个测试函数 * 第一个参数必须为数组 */ public function test_array(array $input_array) { print_r($input_array); } /** * 第一个参数必须为递归类型 */ public function test_interface(Traversable $iterator) { echo get_class($iterator); } /** * 第一个参数必须为回调类型 */ public function test_callable(callable $callback, $data) { call_user_func($callback, $data); } } // OtherClass 类定义 class OtherClass { public $var = 'Hello World'; } ?>
When the parameters of the function call are inconsistent with the defined parameter types, a catchable fatal error will be thrown.
<?php // 两个类的对象 $myclass = new MyClass; $otherclass = new OtherClass; // 致命错误:第一个参数必须是 OtherClass 类的一个对象 $myclass->test('hello'); // 致命错误:第一个参数必须为 OtherClass 类的一个实例 $foo = new stdClass; $myclass->test($foo); // 致命错误:第一个参数不能为 null $myclass->test(null); // 正确:输出 Hello World $myclass->test($otherclass); // 致命错误:第一个参数必须为数组 $myclass->test_array('a string'); // 正确:输出数组 $myclass->test_array(array('a', 'b', 'c')); // 正确:输出 ArrayObject $myclass->test_interface(new ArrayObject(array())); // 正确:输出 int(1) $myclass->test_callable('var_dump', 1); ?>
Type constraints are not only used in class member functions, but also in functions:
<?php // 如下面的类 class MyClass { public $var = 'Hello World'; } /** * 测试函数 * 第一个参数必须是 MyClass 类的一个对象 */ function MyFunction (MyClass $foo) { echo $foo->var; } // 正确 $myclass = new MyClass; MyFunction($myclass); ?>
Type constraints allow NULL values:
<?php /* 接受 NULL 值 */ function test(stdClass $obj = NULL) {} test(NULL); test(new stdClass); ?>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
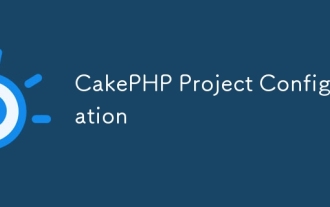
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
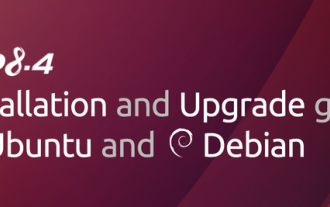
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
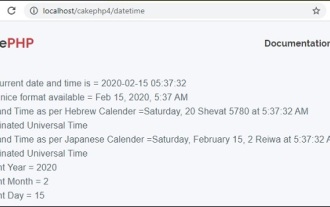
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
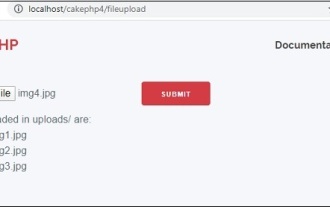
To work on file upload we are going to use the form helper. Here, is an example for file upload.
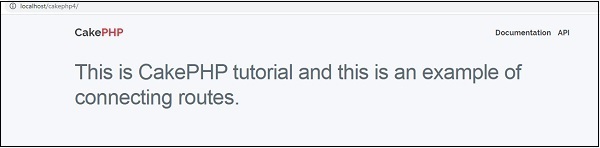
In this chapter, we are going to learn the following topics related to routing ?
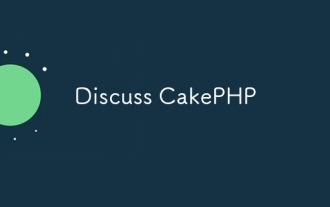
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
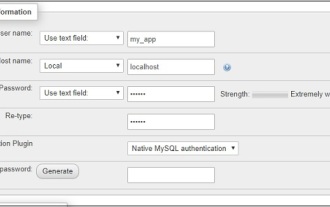
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
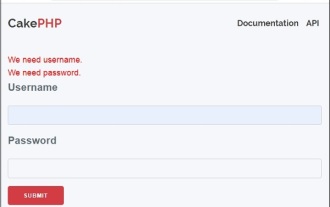
Validator can be created by adding the following two lines in the controller.
