How JavaScript timers work
Before reading the following content, read a small piece of code. If the reader can tell the purpose of the code, there is no need to read further, because you will understand it.
setTimeout(function(){ /* Some long block of code… */ setTimeout(arguments.callee, 10); }, 10); setInterval(function(){ /* Some long block of code… */ }, 10);
The timer is a very cool thing, but many people only know its syntax and lack understanding of its principles. A timer executes a piece of code asynchronously by setting a certain period of time (milliseconds). Because Javascript is a single-threaded language, timers provide the ability to execute code around this language limitation.
Today I will briefly explain how the timer works.
JavaScript provides three functions to build and operate timers
1 var id = setTimeout(fn, delay);
2 var id = setInterval(fn, delay);
3 clearInterval(id); clearTimeout( id);
I won’t go into details about the specific syntax, you can check the manual. In order to understand how timers work, one concept must be kept in mind: time delays are not guaranteed. What does it mean? Just because you write setTimeout(fn, 500) like this does not mean that fn will definitely be executed immediately after 500 milliseconds. The delay is likely to be longer. Because JavaScript is a single-threaded language, all asynchronous events (including timers, mouse events, or the completion of an XMLHttpRequest) will only be executed when there is a gap during program execution. It does not mean that it will be executed when you specify it. You must know the program Users are not omnipotent, and what you write will ultimately depend on the browser.
The picture below can illustrate the problem very well, thank you to the great John Resig.
Looking from top to bottom, the numbers on the left represent time (milliseconds), the text on the right represents the setting and triggering of a series of asynchronous events, and the code block in the middle. The top JavaScript code block may be the fragment you execute when the browser loads, which takes about 18 milliseconds. The Mouse Click Callback code block immediately below may be your callback function when a mouse event is triggered, which takes about 18 milliseconds. 11 milliseconds, and so on.
The single-threaded nature of JavaScript determines that only one block can be executed at a time, so when the first block of code is executed (it ran for a total of 18 milliseconds), it constructed two timers, during which the user may have clicked the mouse. (Have you ever had a webpage get messed up as soon as it was opened before it finished loading?) It stands to reason that the callback function should be executed immediately after the user clicks the mouse, but no, there is only one lane for JavaScript execution. Before the 18 milliseconds are completed, other code blocks can only be queued up if they want to be executed, and there is no room for you to overtake. Both timers have a delay of 10 milliseconds. As you can see from the picture, setTimeout is also triggered before the end of the 18 millisecond execution. There is no way to queue it up.
Finally, 18 milliseconds later, a divine thunder from the sky split the car in front into thin air. The two people in line behind could pass, but they had to go one by one and couldn't be side by side. So who would go through first? Are two people punching each other there? No, the browser has the final say. The browser says that the mouse click event passes first, and setTimeout can only continue to wait for 11 milliseconds. Pay attention to the picture. When the mouse event callback function is executed, another timer event is triggered (setInterval), waiting, and must be ranked behind setTimeout.
11 milliseconds have passed, and setTimeout has finally passed. Note that setInterval is triggered for the second time. Although it was queued the first time, if it is still queued as usual at this time, what will happen in the end? SetTimeout is executed. After that, two setIntervals will be executed continuously, and the delay you set will be useless. Therefore, the browser is relatively smart. When it processes setInterval, if it finds that there is already a queued one, it will directly kill the new one.
Look next, it is the queued setInterval's turn to be triggered for the first time and start executing. When it is executed, the third trigger comes again. This time there is no queue, so the browser does not kill it and gives you a chance to queue. , so you will find that there is no interval between the execution of these two setIntervals. If you are making a slideshow, you should think carefully about whether there is a problem with your code when encountering this situation.
Finally, no other factors interfere with setInterval (if the user is called away by MM), setInterval will be executed according to the steps you want.
At this point, the code at the beginning can be understood.
setTimeout(function(){ /* Some long block of code… */ setTimeout(arguments.callee, 10); }, 10); setInterval(function(){ /* Some long block of code… */ }, 10);
These two functions seem to have the same effect, but they are not. The first code block will always be executed with a delay of 10 milliseconds, although most of the time it is greater than 10 milliseconds. The second one tries to execute every 10 milliseconds, regardless of whether the previous trigger has been executed.
In summary, four points
• The JavaScript engine has only one thread, which will force certain asynchronous events to be queued
• There is a big difference between setTimeout and setInterval when executing asynchronous code
• If a timer is blocked from executing, it Will wait until encountering a code execution gap, usually longer than expected
• Intervals may be executed one after another, if the execution time of the callback function is greater than the interval

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


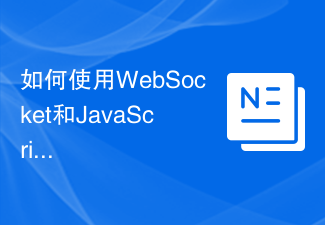
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
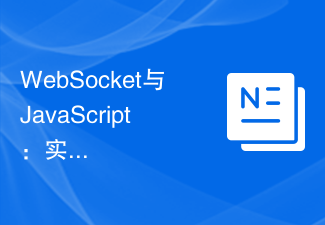
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
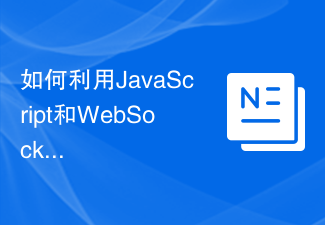
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
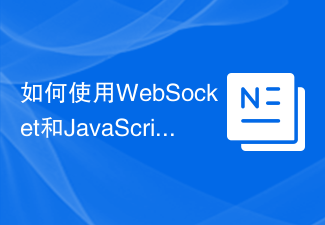
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
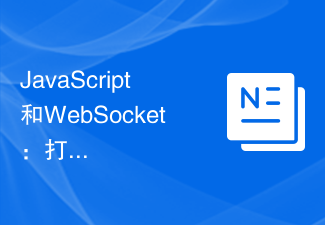
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
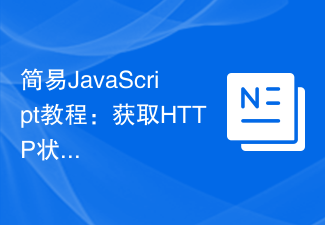
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
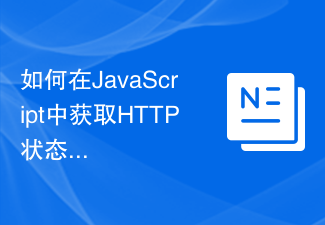
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
