Better utilization of enums under Java 8
In our cloud usage analysis API, formatted analysis data is returned (here refers to generating analysis graphs). Recently, we added a feature that allows users to select a time period (initially only by day). The problem is that the time period of each day in the code is highly coupled...
For example, the following code:
private static List<DataPoint> createListWithZerosForTimeInterval(DateTime from, DateTime to, ImmutableSet<Metric<? extends Number>> metrics) { List<DataPoint> points = new ArrayList<>(); for (int i = 0; i <= Days.daysBetween(from, to).getDays(); i++) { points.add(new DataPoint().withDatas(createDatasWithZeroValues(metrics)) .withDayOfYear(from.withZone(DateTimeZone.UTC) .plusDays(i) .withTimeAtStartOfDay())); } return points; }
Note: Days, Minutes, Hours, Weeks and Months appear at the back of the code part. This code comes from the Joda-Time Java time and date API. Even the names of the methods don't reflect (their respective functionality). These names are firmly tied to the concept of days.
I have also tried using different time periods (such as months, weeks, hours). But I've seen bad switch/cases sneaking around in code.
You need to know that switch/case=sin has penetrated deeply into my heart. I already thought so during my two internship experiences during college. Therefore, I would avoid switch/case at all costs. This is mainly because they violate the open-closed principle. I deeply believe that following this principle is the best practice for writing object-oriented code. I'm not the only one who thinks this way, Robert C. Martin once said:
In many ways, the open-closed principle is the core of object-oriented design. Following this principle will reap tremendous benefits from object-oriented techniques, such as reusability and maintainability1.
I told myself: "We may be able to discover some new features using Java8 to avoid dangerous switch/case situations." Use Java 8's new functions (not that new, but you know what I mean). I decided to use an enum to represent the different available time periods.
public enum TimePeriod { MINUTE(Dimension.MINUTE, (from, to) -> Minutes.minutesBetween(from, to).getMinutes() + 1, Minutes::minutes, from -> from.withZone(DateTimeZone.UTC) .withSecondOfMinute(0) .withMillisOfSecond(0)), HOUR(Dimension.HOUR, (from, to) -> Hours.hoursBetween(from, to).getHours() + 1, Hours::hours, from -> from.withZone(DateTimeZone.UTC) .withMinuteOfHour(0) .withSecondOfMinute(0) .withMillisOfSecond(0)), DAY(Dimension.DAY, (from, to) -> Days.daysBetween(from, to).getDays() + 1, Days::days, from -> from.withZone(DateTimeZone.UTC) .withTimeAtStartOfDay()), WEEK(Dimension.WEEK, (from, to) -> Weeks.weeksBetween(from, to).getWeeks() + 1, Weeks::weeks, from -> from.withZone(DateTimeZone.UTC) .withDayOfWeek(1) .withTimeAtStartOfDay()), MONTH(Dimension.MONTH, (from, to) -> Months.monthsBetween(from, to).getMonths() + 1, Months::months, from -> from.withZone(DateTimeZone.UTC) .withDayOfMonth(1) .withTimeAtStartOfDay()); private Dimension<Timestamp> dimension; private BiFunction<DateTime, DateTime, Integer> getNumberOfPoints; private Function<Integer, ReadablePeriod> getPeriodFromNbOfInterval; private Function<DateTime, DateTime> getStartOfInterval; private TimePeriod(Dimension<Timestamp> dimension, BiFunction<DateTime, DateTime, Integer> getNumberOfPoints, Function<Integer, ReadablePeriod> getPeriodFromNbOfInterval, Function<DateTime, DateTime> getStartOfInterval) { this.dimension = dimension; this.getNumberOfPoints = getNumberOfPoints; this.getPeriodFromNbOfInterval = getPeriodFromNbOfInterval; this.getStartOfInterval = getStartOfInterval; } public Dimension<Timestamp> getDimension() { return dimension; } public int getNumberOfPoints(DateTime from, DateTime to) { return getNumberOfPoints.apply(from, to); } public ReadablePeriod getPeriodFromNbOfInterval(int nbOfInterval) { return getPeriodFromNbOfInterval.apply(nbOfInterval); } public DateTime getStartOfInterval(DateTime from) { return getStartOfInterval.apply(from); } }
With the enumeration, I was able to easily modify the code to allow the user to specify a time period for the chart data points.
The original call was like this:
for (int i = 0; i <= Days.daysBetween(from, to).getDays(); i++)
became the call like this:
for (int i = 0; i < timePeriod.getNumberOfPoints(from, to); i++)
The Usage Analytics service code that supports the getGraphDataPoints call has been completed and supports time periods. It is worth mentioning that it takes into account the open-closed principle I said before.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
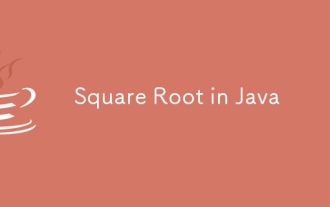
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
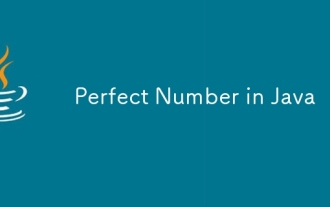
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
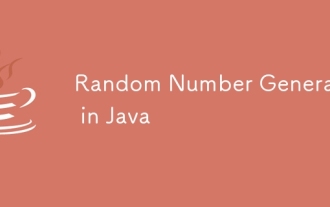
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
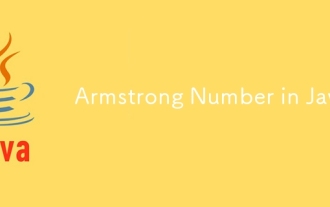
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
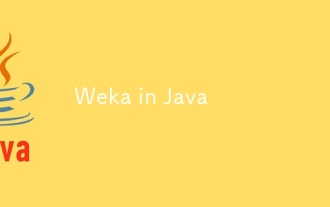
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
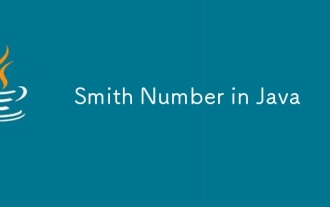
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
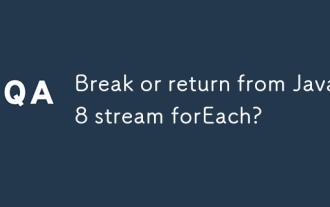
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
