Use of document.cookie in JavaScript
We already know that there is a cookie attribute in the document object. But what are cookies? "Some Web sites store information on your hard drive in small text files called cookies." - MSIE Help. Generally speaking, cookies are created by CGI or similar files, programs, etc. that are more advanced than HTML, but javascript also provides very comprehensive access rights to cookies.
We first need to learn the basic knowledge of Cookies.
Every cookie is like this:
Each cookie has an expiration date. Once the computer clock passes the expiration date, the cookie will be deleted. We cannot delete a Cookie directly, but we can delete it indirectly by setting the expiration date earlier than the current time.
Each web page, or each site, has its own cookies. These cookies can only be accessed by web pages under this site. Web pages from other sites or unauthorized areas under the same site cannot be accessed. of. Each "group" of cookies has a specified total size (approximately 2KB per "group"). Once the maximum total size is exceeded, the earliest expired cookies will be deleted first to allow new cookies to "settle in".
Now let’s learn to use documents.cookie attribute.
If you use the documents.cookie attribute directly, or use some method, such as assigning a value to a variable, to get the value of documents.cookie, we can know how many Cookies there are in the current document and the name of each Cookie. , and its value. For example, adding "document.write(documents.cookie)" to a document, the result shows:
name=kevin; email=kevin@kevin.com; lastvisited=index.html
This means that the document contains 3 Cookies: name, email and lastvisited, their values are kevin, kevin@kevin.com and index.html respectively. As you can see, the two Cookies are separated by semicolons and spaces, so we can use the cookieString.split('; ') method to get an array of each Cookie (first use var cookieString = documents.cookie) .
The way to set a Cookie is to assign a value to documents.cookie. Unlike assignment in other cases, assigning a value to documents.cookie will not delete the original Cookies, but will only add Cookies or change the original Cookies. The format of the assignment:
documents.cookie = 'cookieName=' + escape('cookievalue') + ';expires=' + expirationDateObj.toGMTString();
Are you feeling dizzy? cookieName represents the name of the cookie, cookievalue represents the value of the cookie, and expirationDateObj represents the date object name that stores the expiration date. If the expiration date does not need to be specified, the second line is not needed. If the expiration date is not specified, the browser defaults to expiration after closing the browser (that is, closing all windows).
First, escape() method: why must it be used? Because the requirement for the cookie value is "only characters that can be used in URL encoding". We know that the "escape()" method encodes the string according to the URL encoding method, then we only need to use an "escape()" method to process the value output to the Cookie, and use "unescape()" to process the value output from the Cookie. The value received is foolproof. And the most common use of these two methods is to deal with Cookies. In fact, setting a Cookie is as simple as "documents.cookie = 'cookieName=cookievalue'", but in order to avoid characters that are not allowed to appear in the URL in the cookievalue, it is better to use escape().
Then the semicolon in front of “expires”: Just notice it. It's the semicolon and nothing else.
Finally, the toGMTString() method: The expiration date of the cookie is set in GMT format. Time in other formats has no effect.
Now let’s do it in practice. Set a cookie with "name=rose" to expire after 3 months.
var expires = new Date();
expires.setTime(expires.getTime() + 3 * 30 * 24 * 60 * 60 * 1000);
/* Three months x one month is treated as 30 days x one day 24 Hour
x One hour 60 minutes This is because we know that rose is a valid URL-encoded string, that is, 'rose' == escape('rose'). Generally speaking, if you don't use escape() when setting a cookie, you don't need to use unescape() when getting a cookie.
Let’s do it again: write a function to find the value of a specified cookie.
function getCookie(cookieName) {
var cookieString = documents.cookie;
var start = cookieString.indexOf(cookieName + '=');
// The reason for adding the equal sign is to avoid having "
// The same string as cookieName.
if (start == -1) // Not found
return null;
start += cookieName.length + 1;
var end = cookieString.indexOf(';', start);
if (end == - 1) return unescape(cookieString.substring(start));
return unescape(cookieString.substring(start, end));
}
This function uses some methods of the string object, if you don’t remember (you are It’s not that I have no memory.) Please check it out quickly. All if statements in this function do not include else. This is because if the condition is true, the program will run return statements. If return is encountered in the function, the operation will be terminated, so there is no problem without adding else. This function returns the value of the Cookie when it finds the Cookie, otherwise it returns "null".
Now we need to delete the name=rose Cookie we just set.
var expires = new Date();
expires.setTime(expires.getTime() - 1);
documents.cookie = 'name=rose;expires=' + expires.toGMTString();
You can see, You only need to change the expiration date to be a little earlier than the current date (here it is 1 millisecond earlier), and then set the cookie in the same way to delete the cookie.
Attached is a js function written by a foreigner to operate cookies
///Set cookies
function setCookie(NameOfCookie, value, expiredays)
{
//@Parameters: three variables are used to set new Cookie:
//The name of the cookie, the stored cookie value,
// and the time when the cookie expires.
// These lines convert the number of days into a legal date
var ExpireDate = new Date ();
ExpireDate.setTime(ExpireDate.getTime() + (expiredays * 24 * 3600 * 1000));
// The following line is used to store cookies, just simply assign a value to "document.cookie".
// Note that the date is converted to GMT time through the toGMTstring() function.
document.cookie = NameOfCookie + "=" + escape(value) +
((expiredays == null) ? "" : "; expires=" + ExpireDate.toGMTString());
}
// /Get cookie value
function getCookie(NameOfCookie)
{
// First we check whether the cookie exists.
// If it does not exist, the length of document.cookie is 0
if (document.cookie.length > 0)
{
// Then we check whether the cookie name exists in document.cookie
// Because more than one cookie value is stored, even if the length of document.cookie is not 0, there is no guarantee that the cookie with the name we want exists.
//So we need this step to see if there is a cookie we want
//If the variable value of begin is -1, then it means it does not exist
begin = document.cookie.indexOf(NameOfCookie+"=");
if (begin != -1)
{
// Indicates the existence of our cookie.
begin += NameOfCookie.length+1;//The initial position of the cookie value
end = document.cookie.indexOf(";", begin );//End position
if (end == -1) end = document.cookie.length;//No; then end is the end position of the string
return unescape(document.cookie.substring(begin, end)); }
}
return null;
//The cookie does not exist, return null
}
///Delete cookie
function delCookie (NameOfCookie)
{
//This function checks whether the cookie is set, and if it is set, Adjust the expiration time to the past time;
//The rest is left to the operating system to clean up the cookies at the appropriate time
if (getCookie(NameOfCookie)) {www.2cto.com
document.cookie = NameOfCookie + "= " +
"; expires=Thu, 01-Jan-70 00:00:01 GMT";
}
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


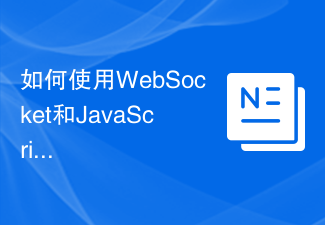
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
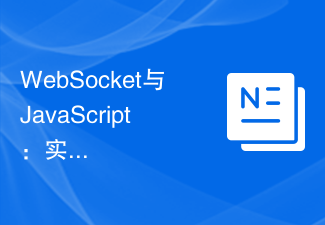
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
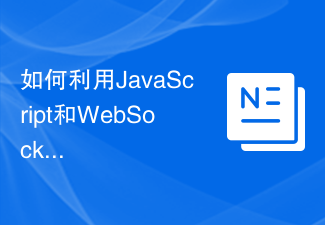
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
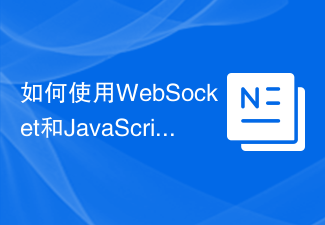
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
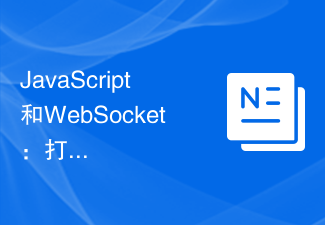
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
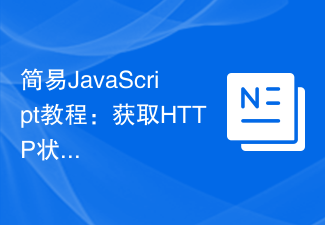
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
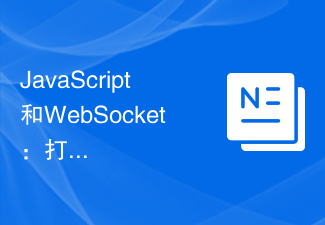
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
