Conversion between bases in java
//Convert decimal to other bases
Integer.toHexString(10); //Convert 10 to hexadecimal and return the string type
Integer.toOctalString(10); //Convert 10 to octal and return String type
Integer.toBinaryString(10); //Convert 10 to binary and return string type
//Convert other bases to decimal
//Convert hexadecimal to decimal, for example: 0xFFFF
Integer.valueOf("FFFF",16).toString(); //The valueOf() method returns the Integer type, and calling toString() returns the string
Integer.parseInt("FFFF",16); // Returns the int basic data type
Integer.toString(0xFFFF); //This method can directly pass in the basic data type representing hexadecimal numbers, and the method returns a string
//Convert octal to decimal, for example: 017
Integer.valueOf("17",8).toString(); //The valueOf() method returns the Integer type, and calling toString() returns a string
Integer.parseInt("17",8); //Return int basic data type
Integer.toString(017); //This method can directly pass in the basic data type representing octal numbers, and the method returns a string
//Convert binary to decimal, for example: 0101
Integer. valueOf("0101",2).toString(); //The valueOf() method returns the Integer type, and calling toString() returns a string
Integer.parseInt("0101",2); //Returns the int basic data type
//For conversion between binary, octal and hexadecimal, you can first convert to decimal, and then use the corresponding method of converting from decimal to multi-decimal for conversion
//For example, convert hexadecimal 0xFF For binary
Integer.toBinaryString(Integer.valueOf("FF",16));
//or
Integer.toBinaryString(Integer.parseInt("FF",16));
//For To input a string representing hexadecimal, you need to intercept the numeric substring first, and then use the valueOf() or parseInt() method to convert it to decimal
//For example, enter 0xFF
String s = "0xFF";
Integer.valueOf(s.subString(2,s.length()),16);
//For the valueOf method, it can be used for boxing of basic data types and conversion between polydecimal and decimal.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
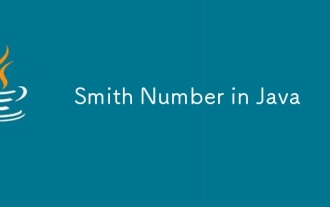
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
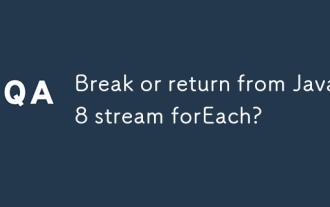
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
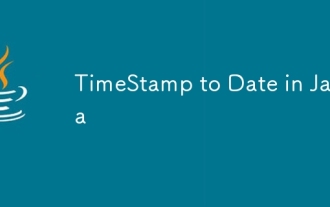
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
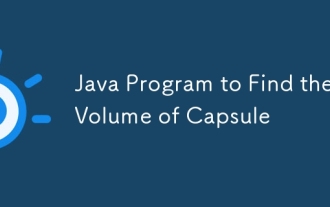
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
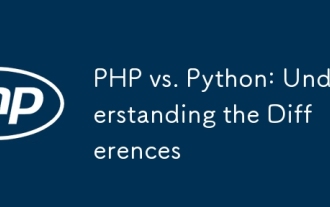
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
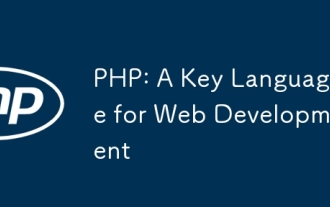
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
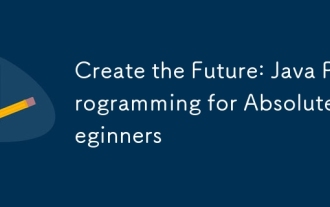
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
