SmartWiki develops Laravel caching extension
Because the SmartWiki demo site is deployed on Alibaba Cloud, Alibaba Cloud has a 128M free Memcache service. After configuring it according to the Memcached configuration method, I found that Laravel reported an error. Check the log and the error location is addServer error and cannot connect to Alibaba Cloud. Memcache.
I was helpless, so I wrote a script according to the Alibaba Cloud installation manual and put it on the server. As a result, I could connect and write.
The script provided by Alibaba Cloud is as follows:
<?php $connect = new Memcached; //声明一个新的memcached链接 $connect->setOption(Memcached::OPT_COMPRESSION, false); //关闭压缩功能 $connect->setOption(Memcached::OPT_BINARY_PROTOCOL, true); //使用binary二进制协议 $connect->addServer('00000000.ocs.aliyuncs.com', 11211); //添加OCS实例地址及端口号 //$connect->setSaslAuthData('aaaaaaaaaa, 'password'); //设置OCS帐号密码进行鉴权,如已开启免密码功能,则无需此步骤 $connect->set("hello", "world"); echo 'hello: ',$connect->get("hello"); print_r( $connect->getVersion()); $connect->quit();
Look at laravel's Memcached driver. The code to create the Memcached object in /vendor/laravel/framework/src/Illuminate/Cache/MemcachedConnector.php is as follows:
public function connect(array $servers) { $memcached = $this->getMemcached(); // For each server in the array, we'll just extract the configuration and add // the server to the Memcached connection. Once we have added all of these // servers we'll verify the connection is successful and return it back. foreach ($servers as $server) { $memcached->addServer( $server['host'], $server['port'], $server['weight'] ); } $memcachedStatus = $memcached->getVersion(); if (! is_array($memcachedStatus)) { throw new RuntimeException('No Memcached servers added.'); } if (in_array('255.255.255', $memcachedStatus) && count(array_unique($memcachedStatus)) === 1) { throw new RuntimeException('Could not establish Memcached connection.'); } return $memcached; }
You can see Laravel's Memcached does not set the option of the setOption method. It only involves the simplest connection establishment, and then calls getVersion to test whether it is connected. Alibaba Cloud's demo code sets the options to turn off compression and use the binary binary protocol.
There is no choice but to extend the functions of Memcached yourself to implement custom options. The extended cache in laravel can be extended using Cache::extend. The extension code is as follows:
Cache::extend('MemcachedExtend', function ($app) { $memcached = $this->createMemcached($app); // 从配置文件中读取缓存前缀 $prefix = $app['config']['cache.prefix']; // 创建 MemcachedStore 对象 $store = new MemcachedStore($memcached, $prefix); // 创建 Repository 对象,并返回 return new Repository($store); });
/** * 创建Memcached对象 * @param $app * @return mixed */ protected function createMemcached($app) { // 从配置文件中读取 Memcached 服务器配置 $servers = $app['config']['cache.stores.MemcachedExtend.servers']; // 利用 Illuminate\Cache\MemcachedConnector 类来创建新的 Memcached 对象 $memcached = new \Memcached; foreach ($servers as $server) { $memcached->addServer( $server['host'], $server['port'], $server['weight'] ); } // 如果服务器上的 PHP Memcached 扩展支持 SASL 认证 if (ini_get('memcached.use_sasl') && isset($app['config']['cache.storess.MemcachedExtend.memcached_user']) && isset($app['config']['cache.storess.MemcachedExtend.memcached_pass'])) { // 从配置文件中读取 sasl 认证用户名 $user = $app['config']['cache.storess.MemcachedExtend.memcached_user']; // 从配置文件中读取 sasl 认证密码 $pass = $app['config']['cache.storess.MemcachedExtend.memcached_pass']; // 指定用于 sasl 认证的账号密码 $memcached->setSaslAuthData($user, $pass); } //扩展 if (isset($app['config']['cache.stores.MemcachedExtend.options'])) { foreach ($app['config']['cache.stores.MemcachedExtend.options'] as $key => $option) { $memcached->setOption($key, $option); } } $memcachedStatus = $memcached->getVersion(); if (! is_array($memcachedStatus)) { throw new RuntimeException('No Memcached servers added.'); } if (in_array('255.255.255', $memcachedStatus) && count(array_unique($memcachedStatus)) === 1) { throw new RuntimeException('Could not establish Memcached connection.'); } return $memcached; }
The cache extension code requires creating a ServiceProvider to register the service provider. The service provider is the center of Laravel application startup. Your own application and all Laravel's core services are started through the service provider.
But what do we mean by “startup”? Typically, this means registering things, including registering service container bindings, event listeners, middleware, and even routes. Service providers are the center of application configuration.
If you open the config/app.php file that comes with Laravel, you will see a providers array. Here are all the service provider classes to be loaded by the application. Of course, many of them are lazy loaded, which means that they are not loaded every time. They will be loaded every request, and will only be loaded when they are actually used.
All service providers inherit from the IlluminateSupportServiceProvider class. Most service providers contain two methods: register and boot . In the register method, the only thing you have to do is bind the thing to the service container. Do not try to register event listeners, routes or any other functionality in it.
You can simply generate a new provider through the Artisan command make:provider:
php artisan make:provider MemcachedExtendServiceProvider
All service providers are registered through the configuration file config/app.php, which contains A providers array listing the names of all service providers. By default, all core service providers are listed. These service providers enable core Laravel components such as mail, queues, caches, etc.
To register your own service provider, just append it to the array:
'providers' => [ SmartWiki\Providers\MemcachedExtendServiceProvider::class // 在providers节点添加实现的provider ]
Also configure the Memcached configuration in config/cache.php:
'MemcachedExtend' => [ 'driver' => 'MemcachedExtend', 'servers' => [ [ 'host' => env('MEMCACHED_EXTEND_HOST', '127.0.0.1'), 'port' => env('MEMCACHED_EXTEND_PORT', 11211), 'weight' => 100, ], ], 'options' => [ \Memcached::OPT_BINARY_PROTOCOL => true, \Memcached::OPT_COMPRESSION => false ] ]
If you need to also store the Session in our extension We also need to call Session::extend in the cache to expand our Session storage:
Session::extend('MemcachedExtend',function ($app){ $memcached = $this->createMemcached($app); return new MemcachedSessionHandler($memcached); });
Then we can configure our extended cache in .env. The complete code is as follows:
<?php namespace SmartWiki\Providers; use Illuminate\Cache\Repository; use Illuminate\Cache\MemcachedStore; use Illuminate\Support\ServiceProvider; use Cache; use Session; use Symfony\Component\HttpFoundation\Session\Storage\Handler\MemcachedSessionHandler; use RuntimeException; class MemcachedExtendServiceProvider extends ServiceProvider { /** * Bootstrap the application services. * * @return void */ public function boot() { Cache::extend('MemcachedExtend', function ($app) { $memcached = $this->createMemcached($app); // 从配置文件中读取缓存前缀 $prefix = $app['config']['cache.prefix']; // 创建 MemcachedStore 对象 $store = new MemcachedStore($memcached, $prefix); // 创建 Repository 对象,并返回 return new Repository($store); }); Session::extend('MemcachedExtend',function ($app){ $memcached = $this->createMemcached($app); return new MemcachedSessionHandler($memcached); }); } /** * Register the application services. * * @return void */ public function register() { // } /** * 创建Memcached对象 * @param $app * @return mixed */ protected function createMemcached($app) { // 从配置文件中读取 Memcached 服务器配置 $servers = $app['config']['cache.stores.MemcachedExtend.servers']; // 利用 Illuminate\Cache\MemcachedConnector 类来创建新的 Memcached 对象 $memcached = new \Memcached; foreach ($servers as $server) { $memcached->addServer( $server['host'], $server['port'], $server['weight'] ); } // 如果服务器上的 PHP Memcached 扩展支持 SASL 认证 if (ini_get('memcached.use_sasl') && isset($app['config']['cache.storess.MemcachedExtend.memcached_user']) && isset($app['config']['cache.storess.MemcachedExtend.memcached_pass'])) { // 从配置文件中读取 sasl 认证用户名 $user = $app['config']['cache.storess.MemcachedExtend.memcached_user']; // 从配置文件中读取 sasl 认证密码 $pass = $app['config']['cache.storess.MemcachedExtend.memcached_pass']; // 指定用于 sasl 认证的账号密码 $memcached->setSaslAuthData($user, $pass); } //扩展 if (isset($app['config']['cache.stores.MemcachedExtend.options'])) { foreach ($app['config']['cache.stores.MemcachedExtend.options'] as $key => $option) { $memcached->setOption($key, $option); } } $memcachedStatus = $memcached->getVersion(); if (! is_array($memcachedStatus)) { throw new RuntimeException('No Memcached servers added.'); } if (in_array('255.255.255', $memcachedStatus) && count(array_unique($memcachedStatus)) === 1) { throw new RuntimeException('Could not establish Memcached connection.'); } return $memcached; } } SmartWikiCode

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


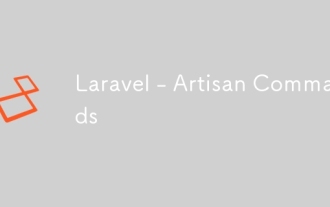
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
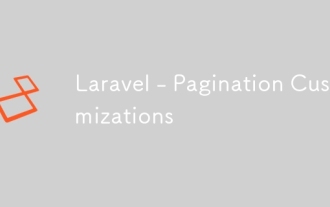
Laravel - Pagination Customizations - Laravel includes a feature of pagination which helps a user or a developer to include a pagination feature. Laravel paginator is integrated with the query builder and Eloquent ORM. The paginate method automatical
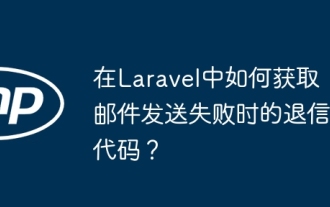
Method for obtaining the return code when Laravel email sending fails. When using Laravel to develop applications, you often encounter situations where you need to send verification codes. And in reality...
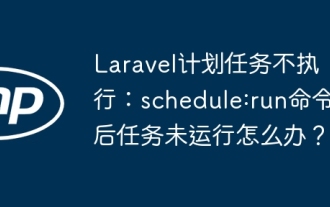
Laravel schedule task run unresponsive troubleshooting When using Laravel's schedule task scheduling, many developers will encounter this problem: schedule:run...
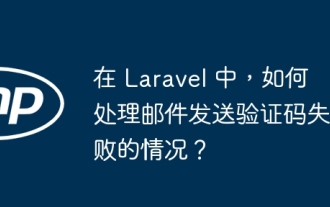
The method of handling Laravel's email failure to send verification code is to use Laravel...
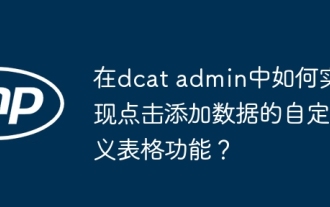
How to implement the table function of custom click to add data in dcatadmin (laravel-admin) When using dcat...
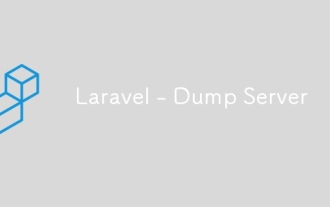
Laravel - Dump Server - Laravel dump server comes with the version of Laravel 5.7. The previous versions do not include any dump server. Dump server will be a development dependency in laravel/laravel composer file.
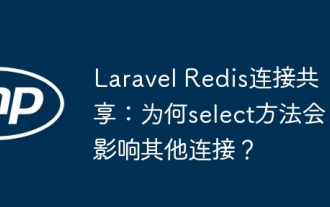
The impact of sharing of Redis connections in Laravel framework and select methods When using Laravel framework and Redis, developers may encounter a problem: through configuration...
