List of Python built-in functions
1. Mathematical operations
abs(-5) # Equivalent to 2**3, if it is pow (2, 3, 5), equivalent to 2**3 % 5
cmp(2.3, 3.2) ,2,9]) # Find the maximum value
min([9,2,-4,2]) # Find the minimum value
sum([2,-1,9,12]) # Find the sum
2. Type Convert
int("5") # Convert to integer
float(2) # Convert to floating point number float
long("23") # Convert to long integer
str(2.3) # Convert to string
complex(3, 9) # Return the complex number 3 + 9i The character corresponding to 65
unichr(65) # The unicode corresponding to the value 65 Character lBool (0) # Convert to the corresponding authentic values. In Python, 0 is equivalent to false. In python, the following objects are equivalent to false: ** [], (), {}, 0, none , 0.0, '' **
bin(56) 6) # Return A string representing the octal number 56
list((1,2,3)) ,-1) , "hello!"]) # Whether all elements are equivalent to True value
any(["", 0, False, [], None]) # Whether any element is equivalent to True value
sorted([1 )Reversed ([1,5,3]) # Returns the sequence of the backflow, which is [3,5,1]
4, class, object, attributes
# define class
Class me (Object):
DEF TEST ( self): me, "test") # Return the test attribute
setattr(me, "test", new_test) # Set the test attribute to new_test
delattr(me, "test") # Delete the test attribute
isinstance(me, Me) # me Whether the object is an object generated by the Me class (an instance)
issubclass(Me, object) # Whether the Me class is a subclass of the object class
5. Compilation and execution
repr(me) # Return the string expression of the object
compile ("Print ('hello')", 'test.py', 'exec') # compile string becomes CODE object
eval ("1 + 1") # Explanation string expression. The parameter can also be the code object returned by compile()
exec("print('Hello')") # Interpret and execute the string, print('Hello'). The parameter can also be the code object returned by compile()
6, others
input("Please input:") # Return to local namespace

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


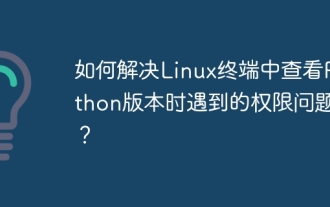
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
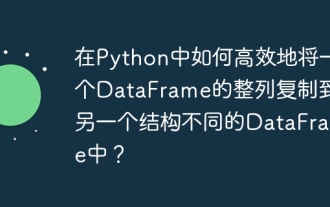
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
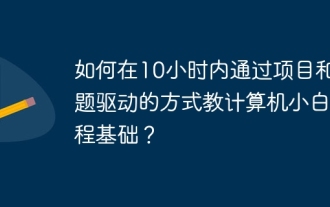
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
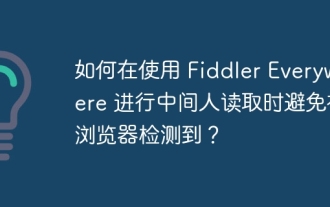
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
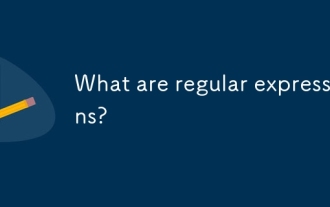
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
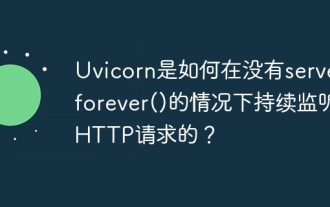
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
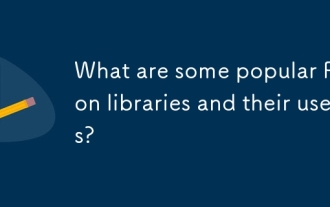
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
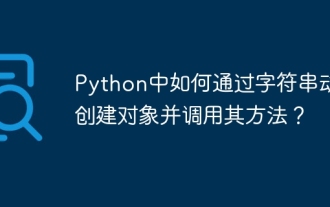
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
