Vuex 2.0 about Vue.js 2.0 The knowledge base you need to update
Application Structure
In fact, Vuex has no restrictions on how to organize your code structure. On the contrary, it enforces a series of high-level principles:
1. Application-level status is concentrated in the store.
2. The only way to change the state is to submit mutations, which is a synchronous transaction.
3. Asynchronous logic should be encapsulated in action.
As long as you follow these rules, it's up to you how you structure your project. If your store file is very large, just split it into action, mutation and getter files.
For slightly more complex applications, we may need to use modules. The following is a simple project structure:
├── index.html
├── main.js
├── api
│ └── ... # Initiate API request here
├── components
│ ├─ ─ app.Vuep│ └ ── ...
└ ─
└ ─ ─ ─ i ├ ├ ─ — Actions.js # ├ ─ ─ ─ ─ ─ ─ ─ ─ Mutations
└── modules
├── cart.js └── cart.js └── products.js # products module
For more, check out the shopping cart example.
Modules
Due to the use of a single state tree, all the state of the application is contained in one large object. However, as the scale of our application continued to grow, this Store became very bloated.
In order to solve this problem, Vuex allows us to divide the store into modules. Each module contains its own state, mutation, action and getter, even nested modules. This is how it is organized:
const moduleA = { state: { ... }, mutations: { ... }, actions: { ... }, getters: { ... } } const moduleB = { state: { ... }, mutations: { ... }, actions: { ... } } const store = new Vuex.Store({ modules: { a: moduleA, b: moduleB } }) store.state.a // -> moduleA's state store.state.b // -> moduleB's state
Module local state
The mutations and getters methods of the module are the first to receive parameters Is the local state of the module.
const moduleA = { state: { count: 0 }, mutations: { increment: (state) { // state 是模块本地的状态。 state.count++ } }, getters: { doubleCount (state) { return state.count * 2 } } }
Similarly, in the module's actions, context.state exposes the local state, and context.rootState exposes the root state.
const moduleA = { // ... actions: { incrementIfOdd ({ state, commit }) { if (state.count % 2 === 1) { commit('increment') } } } }
In the getters of the module, the root state is also exposed as the third parameter.
const moduleA = { // ... getters: { sumWithRootCount (state, getters, rootState) { return state.count + rootState.count } } }
Namespace
Please note that the actions, mutations and getters within the module are still registered in the global namespace - this will allow multiple modules to respond to the same mutation/action type. You can add a prefix or suffix to the module name to set the namespace to avoid naming conflicts. If your Vuex module is reusable and the execution environment is unknown, then you should do this. Distance, we want to create a todos module:
// types.js // 定义 getter、 action 和 mutation 的常量名称 // 并且在模块名称上加上 `todos` 前缀 export const DONE_COUNT = 'todos/DONE_COUNT' export const FETCH_ALL = 'todos/FETCH_ALL' export const TOGGLE_DONE = 'todos/TOGGLE_DONE' // modules/todos.js import * as types from '../types' // 用带前缀的名称来定义 getters, actions and mutations const todosModule = { state: { todos: [] }, getters: { [types.DONE_COUNT] (state) { // ... } }, actions: { [types.FETCH_ALL] (context, payload) { // ... } }, mutations: { [types.TOGGLE_DONE] (state, payload) { // ... } } }
Register a dynamic module
You can use the store.registerModule method to register a module after the store is created:
store.registerModule('myModule', { // ... })
module’s store.state. myModule is exposed as the module's state.
Other Vue plug-ins can attach a module to the application store, and then use Vuex's state management function through dynamic registration. For example, the vuex-router-sync library integrates vue-router and vuex by managing the routing state of the application in a dynamically registered module.
You can also use store.unregisterModule(moduleName) to remove dynamically registered modules. But you cannot use this method to remove static modules (that is, modules declared when the store is created).
Plugins
Vuex’s store receives the plugins option, which exposes hooks for each mutation. A Vuex plug-in is a simple method that accepts sotre as the only parameter:
const myPlugin = store => { // 当 store 在被初始化完成时被调用 store.subscribe((mutation, state) => { // mutation 之后被调用 // mutation 的格式为 {type, payload}。 }) }
Then use it like this:
const store = new Vuex.Store({ // ... plugins: [myPlugin] })
Submit Mutations within the plug-in
Plugins cannot directly modify the state - this is Like your components, they can only be changed by mutations.
By submitting mutations, the plug-in can be used to synchronize the data source to the store. For example, in order to synchronize the websocket data source to the store (this is just an example to illustrate the usage, in practice, the createPlugin method will be appended with more options to complete complex tasks).
export default function createWebSocketPlugin (socket) { return store => { socket.on('data', data => { store.commit('receiveData', data) }) store.subscribe(mutation => { if (mutation.type === 'UPDATE_DATA') { socket.emit('update', mutation.payload) } }) } }
const plugin = createWebSocketPlugin(socket) const store = new Vuex.Store({ state, mutations, plugins: [plugin] })
Generate status snapshot
Sometimes the plug-in wants to get the status "snapshot" and the changes before and after the status change. In order to implement these functions, a deep copy of the state object is required:
const myPluginWithSnapshot = store => { let prevState = _.cloneDeep(store.state) store.subscribe((mutation, state) => { let nextState = _.cloneDeep(state) // 对比 prevState 和 nextState... // 保存状态,用于下一次 mutation prevState = nextState }) }
** The plug-in that generates the state snapshot can only be used during the development phase. Use Webpack or Browserify and let the build tool handle it for us:
const store = new Vuex.Store({ // ... plugins: process.env.NODE_ENV !== 'production' ? [myPluginWithSnapshot] : [] })
The plug-in will be enabled by default. To ship to production, you need to use Webpack's DefinePlugin or Browserify's envify to convert process.env.NODE_ENV !== 'production' to false.
Built-in Logger plug-in
如果你正在使用 vue-devtools,你可能不需要。
Vuex 带来一个日志插件用于一般的调试:
import createLogger from 'vuex/dist/logger' const store = new Vuex.Store({ plugins: [createLogger()] })
createLogger 方法有几个配置项:
const logger = createLogger({ collapsed: false, // 自动展开记录 mutation transformer (state) { // 在记录之前前进行转换 // 例如,只返回指定的子树 return state.subTree }, mutationTransformer (mutation) { // mutation 格式 { type, payload } // 我们可以按照想要的方式进行格式化 return mutation.type } })
日志插件还可以直接通过

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


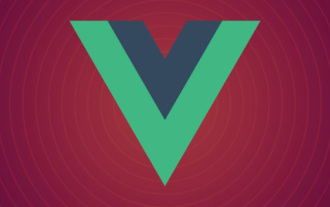
When using the Vue framework to develop front-end projects, we will deploy multiple environments when deploying. Often the interface domain names called by development, testing and online environments are different. How can we make the distinction? That is using environment variables and patterns.
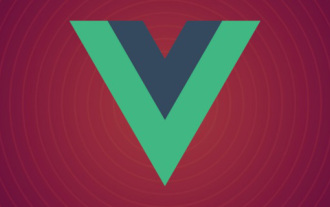
The difference between componentization and modularization: Modularization is divided from the perspective of code logic; it facilitates code layered development and ensures that the functions of each functional module are consistent. Componentization is planning from the perspective of UI interface; componentization of the front end facilitates the reuse of UI components.
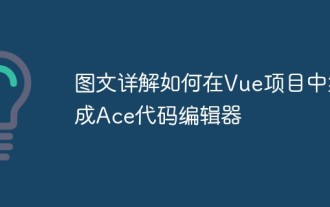
Ace is an embeddable code editor written in JavaScript. It matches the functionality and performance of native editors like Sublime, Vim, and TextMate. It can be easily embedded into any web page and JavaScript application. Ace is maintained as the main editor for the Cloud9 IDE and is the successor to the Mozilla Skywriter (Bespin) project.
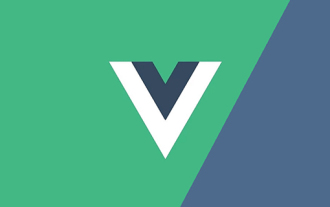
Foreword: In the development of vue3, reactive provides a method to implement responsive data. This is a frequently used API in daily development. In this article, the author will explore its internal operating mechanism.
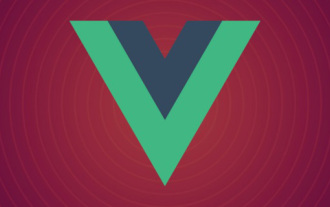
Vue.js has become a very popular framework in front-end development today. As Vue.js continues to evolve, unit testing is becoming more and more important. Today we’ll explore how to write unit tests in Vue.js 3 and provide some best practices and common problems and solutions.
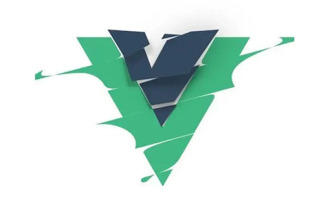
How to handle exceptions in Vue3 dynamic components? The following article will talk about Vue3 dynamic component exception handling methods. I hope it will be helpful to everyone!
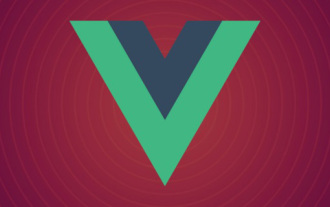
In Vue.js, developers can use two different syntaxes to create user interfaces: JSX syntax and template syntax. Both syntaxes have their own advantages and disadvantages. Let’s discuss their differences, advantages and disadvantages.
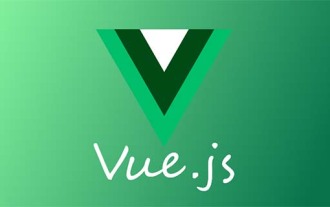
In the actual development project process, sometimes it is necessary to upload relatively large files, and then the upload will be relatively slow, so the background may require the front-end to upload file slices. It is very simple. For example, 1 A gigabyte file stream is cut into several small file streams, and then the interface is requested to deliver the small file streams respectively.
