Example of native implementation of FastClick event in js
Note: I have not been learning JavaScript for a long time. I have been working on mobile web pages and WeChat applications on the web recently. Since I have recently used functions similar to fastclick, I used touchstart and touchend events to simulate them in the original program. Now I am trying to encapsulate them. The following two problematic solutions were obtained. Share it with everyone, and ask for guidance from the master
In mobile web app development, the 300ms delay of the click event will cause slow response, especially in low-end machines. Using touchstart or touchend events will conflict with the default wheel event, which is not what we expect.
So, I did it myself, had enough food and clothing, and wrote a native js code for a quick click event (considering the environment of web app development, we do not need to consider compatibility with browsers such as IE for the time being).
Implementation method 1 is as follows:
function FastClickEvent(handler){ var fastclick = { handler : handler, bind : function(query){ var targetList = document.querySelectorAll(query); for(var i=0,len=targetList.length;i<len;i++) { targetList[i].addEventListener('touchstart',handleEvent); targetList[i].addEventListener('touchend',handleEvent); } }, unbind : function(query){ var targetList = document.querySelectorAll(query); for(var i=0,len=targetList.length;i<len;i++) { targetList[i].removeEventListener('touchstart',handleEvent); targetList[i].removeEventListener('touchend',handleEvent); } } } var touchX = 0 ,touchY = 0; function handleEvent(event){ switch(event.type) { case 'touchstart': touchX = event.touches[0].clientX; touchY = event.touches[0].clientY; break; case 'touchend': var x = event.changedTouches[0].clientX; var y = event.changedTouches[0].clientY; if(Math.abs(touchX-x)<5||Math.abs(touchY-y)<5) fastclick.handler(event); break; } }; return fastclick; };
Principle: Based on the change in position when continuous touchstart and touchend events occur, determine whether it is a click
Call: Use a handler function to register a FastClickEvent event. Then bind the registered FastClickEvent event to the corresponding element through the bind method. As follows:
var handler = function(event){ console.log(event.target.id+" fastclicked"); } var fastClick = new FastClickEvent(handler); fastClick.bind("div");
In this code, we register the fastclick handler event for all div elements. Call fastClick.unbind to unbind an element.
But there is a problem with this code, in order to allow the handleEvent event to access touchX, touchY. I used a closure method, which means that every time a new FastClickEvent event object is created, a repeated handleEvent function must be injected into the memory. As for the repeated touchX and touchY, there is no need to say more.
Newbie help: I originally wanted to write the handleEvent function into the prototype, but a problem arises is that the this object of handleEvent (event) is windows, that is to say, I cannot get the touchX, touchY and handler objects, causing an access error. .
There is a relatively simple solution, which is to only register one fastClickEvent event, and then determine the response content in the handler based on the actual value of event.target (that is, the object where the event occurs).
However, this means you must be very familiar with all fastclick events.
The advantage of using this method is that since you only have one handleEvent function, basically, before the page is released, unless you don’t want to trigger the fastclick event anymore, you don’t need to unbind the fastclick event of any element (even if If you unbind it, the handler function still exists in the memory). Moreover, you can easily use bind(query) to add the fastclick event of any dynamically generated element, as long as you have written the corresponding handler in the handler function.
If you want to add multiple fastclick events, and you may need to register them in multiple places, you only need to create a new FastClickEvent object and bind it to the corresponding element.
The following is a method of using the EventTarget class. First, let’s take a look at the EventTarget
function EventTarget(){ this.handlers = {}; } EventTarget.prototype = { constructor: EventTarget, addHandler : function(type,handler){ if(typeof this.handlers[type] == "undefined"){ this.handlers[type]=[]; } this.handlers[type].push(handler); }, fire : function(event){ if(!event.target){ event.target = this; } if(this.handlers[event.type] instanceof Array){ var handlers = this.handlers[event.type]; for(var i=0,len=handlers.length;i<len;i++){ handlers[i](event); } } }, removeHandler : function(type,handler){ if(this.handlers[type] instanceof Array){ var handlers = this.handlers[type]; for(var i=0,len=handler.length;i<len;i++){ if(handlers[i]==handler){ break; } } handlers.splice(i,1); } } }
class, which is an interface used to add, remove and implement custom classes. Refer to "JavaScript Advanced Programming Third Edition" P616-617
So, how to turn this class into our fastclick event interface?
Define a global variable and use this variable to complete all fastclick event registration, deletion and addition
var FastClick = function(){ var fastclick = new EventTarget(), touchX = 0 , touchY = 0; function handleEvent(event){ switch(event.type) { case 'touchstart': touchX = event.touches[0].clientX; touchY = event.touches[0].clientY; break; case 'touchend': var x = event.changedTouches[0].clientX; var y = event.changedTouches[0].clientY; if(Math.abs(touchX-x)<5||Math.abs(touchY-y)<5) fastclick.fire({type:'fastclick',target:event.target}); break; } }; fastclick.bind = function(query) { var targetList = document.querySelectorAll(query); for(var i=0,len=targetList.length;i<len;i++) { targetList[i].addEventListener('touchstart',handleEvent); targetList[i].addEventListener('touchend',handleEvent); } } Fastclick.unbind = function(query){ var targetList = document.querySelectorAll(query); for(var i=0,len=targetList.length;i<len;i++) { targetList[i].removeEventListener('touchstart',handleEvent); targetList[i].removeEventListener('touchend',handleEvent); } } return fastclick; }();
This global variable FastClick can be used to add any fastclick event.
Let’s talk about how to call it.
Add event function:
FastClick.addHandler('fastclick',function(event){});
Delete event function: //Anonymous events cannot be deleted
FastClick.removeHandler('fastclick',handler);
Binding elements
FastClick.bind("div");
Unbinding
FastClick.unbind("div");
Using this method, we also need to predict the event.target in the handler event , because although this method can add multiple fastclick events, the events are executed one by one in sequence during execution, which means that functions you do not want to execute may be executed. The benefit of
is that multiple fastclick events can be registered and executed without binding again.
For example,
FastClick.bind("div"); FastClick.addHandler(handler1); FastClick.addHandler(handler2);
Then, when a quick click event occurs on any div element, handler1 and handler2 will be executed sequentially.
If we call removeHandler to delete handler1 or handler2, the corresponding function will no longer be executed.
In addition, it should be noted that in the handler function, this object is the array FastClick.handlers['fastclick']. Generally, we use event.target to obtain the object where the event occurred.
Using this method basically overcomes the problems of the above method. Moreover, repeating new for this object does not make much sense unless you don’t want to predict the event.target and generate a lot of FaskClick classes, but this Obviously it's not efficient.
Newbie help: How can I implement a binding execution function for a specific element, that is: I can call FastClick.bind(query,handler); to implement the fastclick event of adding a handler to elements that meet the query conditions.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


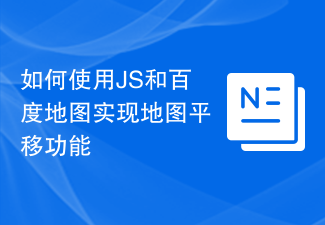
How to use JS and Baidu Map to implement map pan function Baidu Map is a widely used map service platform, which is often used in web development to display geographical information, positioning and other functions. This article will introduce how to use JS and Baidu Map API to implement the map pan function, and provide specific code examples. 1. Preparation Before using Baidu Map API, you first need to apply for a developer account on Baidu Map Open Platform (http://lbsyun.baidu.com/) and create an application. Creation completed
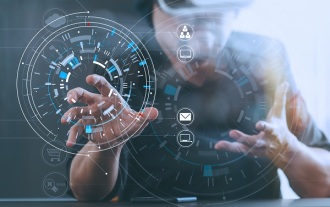
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
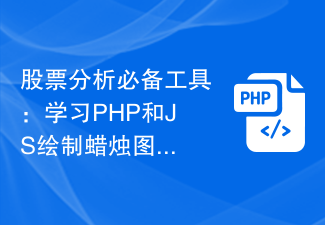
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
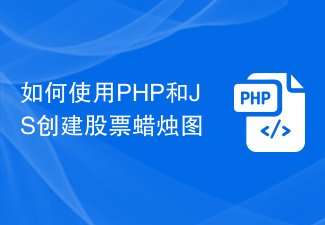
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
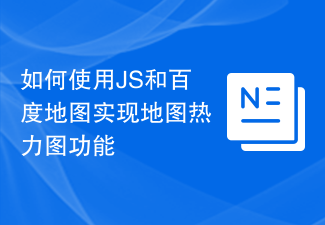
How to use JS and Baidu Maps to implement the map heat map function Introduction: With the rapid development of the Internet and mobile devices, maps have become a common application scenario. As a visual display method, heat maps can help us understand the distribution of data more intuitively. This article will introduce how to use JS and Baidu Map API to implement the map heat map function, and provide specific code examples. Preparation work: Before starting, you need to prepare the following items: a Baidu developer account, create an application, and obtain the corresponding AP
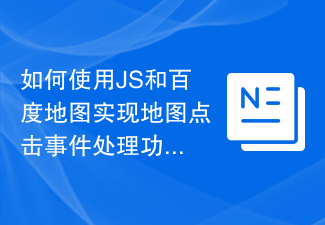
Overview of how to use JS and Baidu Maps to implement map click event processing: In web development, it is often necessary to use map functions to display geographical location and geographical information. Click event processing on the map is a commonly used and important part of the map function. This article will introduce how to use JS and Baidu Map API to implement the click event processing function of the map, and give specific code examples. Steps: Import the API file of Baidu Map. First, import the file of Baidu Map API in the HTML file. This can be achieved through the following code:
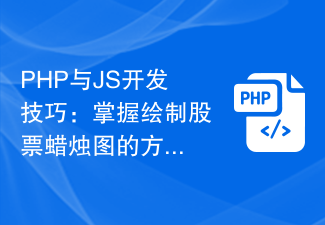
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
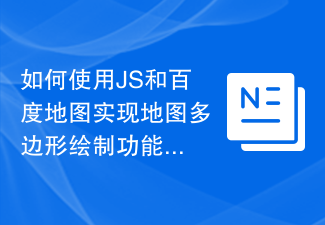
How to use JS and Baidu Maps to implement map polygon drawing function. In modern web development, map applications have become one of the common functions. Drawing polygons on the map can help us mark specific areas for users to view and analyze. This article will introduce how to use JS and Baidu Map API to implement map polygon drawing function, and provide specific code examples. First, we need to introduce Baidu Map API. You can use the following code to import the JavaScript of Baidu Map API in an HTML file
