Detailed explanation of form validation in AngularJS
AngularJS自带了很多验证,什么必填,最大长度,最小长度...,这里记录几个有用的正则式验证
1.使用angularjs的表单验证
正则式验证
只需要配置一个正则式,很方便的完成验证,理论上所有的验证都可以用正则式完成
//javascript $scope.mobileRegx = "^1(3[0-9]|4[57]|5[0-35-9]|7[01678]|8[0-9])\\d{8}$"; $scope.emailRegx = "^[a-z]([a-z0-9]*[-_]?[a-z0-9]+)*@([a-z0-9]*[-_]?[a-z0-9]+)+[\.][a-z]{2,3}([\.][a-z]{2})?$"; $scope.pwdRegx = "[a-zA-Z0-9]*"; <div class="form-group"> <input class="form-control" name="mobile" type="text" ng-model="account.mobile" required ng-pattern="mobileRegx"/> <span class="error" ng-show="registerForm.$submitted && registerForm.mobile.$error.required">*手机号不能为空</span> <span class="error" ng-show="registerForm.$submitted && registerForm.mobile.$error.pattern">*手机号地址不合法</span> </div> <input type="text" ng-pattern="/[a-zA-Z]/" />
数字
设置input的type=number即可
<div class="form-group col-md-6"> <label for="password"> 预估时间 </label> <div class="input-group"> <input required type="number" class="form-control" ng-model="task.estimated_time" name="time" /> <span class="input-group-addon">分钟</span> </div> <span class="error" ng-show="newTaskForm.$submitted && newTaskForm.time.$error.required">*请预估时间</span> </div>
邮箱
<input type="email" name="email" ng-model="user.email" />
URL
<input type="url" name="homepage" ng-model="user.facebook_url" />
效果
效果是实时的,很带感
2.使用ngMessages验证表单
angular.module('ngMessagesExample', ['ngMessages']); <form name="myForm"> <label> Enter your name: <input type="text" name="myName" ng-model="name" ng-minlength="5" ng-maxlength="20" required /> </label> <pre class="brush:php;toolbar:false">myForm.myName.$error = {{ myForm.myName.$error | json }}
简直是第二次解放啊。。。
2.动态生成的表单的验证
如果你有一部分form的内容是动态生成的,数量和名字都不确定的时候,该如何验证这些生成的元素呢?其实维护一个动态的form本来就是个问题。但是在angular中,只需要记住一点:准备好你的数据,其他的交给angular。像下面这个例子,你只需要维护好一个数组,然后利用ng-repeat就可以快速的呈现到页面上。
其实动态的form和一般的form验证都是一致的,只是动态的form需要使用myForm[inout_name].$error的形式访问
<!-- 动态显示状态的负责人 --> <div class="form-group col-md-6" ng-repeat="sta in task.status_table | orderBy : sta.sequence_order"> <label>{{sta.status_name}} 负责人</label> <select required class="form-control" ng-model="sta.user_id" ng-options="qa.id as qa.last_name+' '+qa.last_name for qa in taskUserList" name="{{sta.status_name}}"> </select> <div ng-messages="newTaskForm[sta.status_name].$error" ng-if="newTaskForm.$submitted || newTaskForm[sta.status_name].$touched"> <p class="error" ng-message="required">*请选择负责人</p> </div> </div>
3. 必填项加亮显
有些表单我们不希望粗暴的在下面添加文字信息提示验证,而是希望更简洁的方式,如:加亮边框,突出显示
需要做的只是在required验证没通过的时候添加class,改变元素的样式即可
<form name="loginForm" novalidate ng-submit="loginPost()" class="navbar-form navbar-right" ng-hide="login"> <fake-autocomplete></fake-autocomplete> <div class="form-group"> <input name="user_name" required maxlength="50" type="text" class="form-control" placeholder="手机号或邮箱" ng-model="account.user_name" ng-class="{warn:loginForm.$submitted && loginForm.user_name.$error.required}"> </div> <div class="form-group"> <input name="password" required type="password" maxlength="50" placeholder="密码" ng-model="account.password" ng-class="{'form-control':1,warn:loginForm.$submitted && loginForm.password.$error.required}"> </div> <button type="submit" class="btn btn-default"><span class="glyphicon glyphicon-lock"></span> 登录</button> </form> .warn { border-color: #f78d8d !important; outline: 0 !important; -webkit-box-shadow: inset 0 1px 1px rgba(0, 0, 0, .075), 0 0 8px rgb(239, 154, 154) !important; box-shadow: inset 0 1px 1px rgba(0, 0, 0, .075), 0 0 8px rgba(239, 154, 154) !important; }

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Javascript is a very unique language. It is unique in terms of the organization of the code, the programming paradigm of the code, and the object-oriented theory. The issue of whether Javascript is an object-oriented language that has been debated for a long time has obviously been There is an answer. However, even though Javascript has been dominant for twenty years, if you want to understand popular frameworks such as jQuery, Angularjs, and even React, just watch the "Black Horse Cloud Classroom JavaScript Advanced Framework Design Video Tutorial".
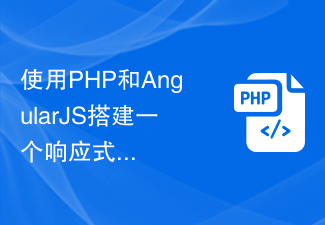
In today's information age, websites have become an important tool for people to obtain information and communicate. A responsive website can adapt to various devices and provide users with a high-quality experience, which has become a hot spot in modern website development. This article will introduce how to use PHP and AngularJS to build a responsive website to provide a high-quality user experience. Introduction to PHP PHP is an open source server-side programming language ideal for web development. PHP has many advantages, such as easy to learn, cross-platform, rich tool library, development efficiency
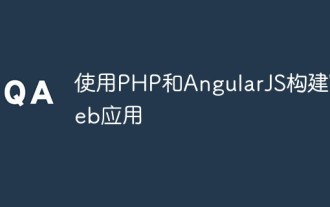
With the continuous development of the Internet, Web applications have become an important part of enterprise information construction and a necessary means of modernization work. In order to make web applications easy to develop, maintain and expand, developers need to choose a technical framework and programming language that suits their development needs. PHP and AngularJS are two very popular web development technologies. They are server-side and client-side solutions respectively. Their combined use can greatly improve the development efficiency and user experience of web applications. Advantages of PHPPHP
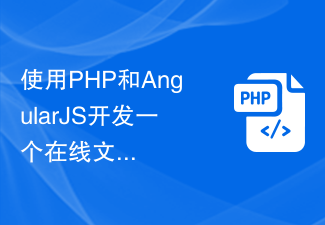
With the popularity of the Internet, more and more people are using the network to transfer and share files. However, due to various reasons, using traditional methods such as FTP for file management cannot meet the needs of modern users. Therefore, establishing an easy-to-use, efficient, and secure online file management platform has become a trend. The online file management platform introduced in this article is based on PHP and AngularJS. It can easily perform file upload, download, edit, delete and other operations, and provides a series of powerful functions, such as file sharing, search,
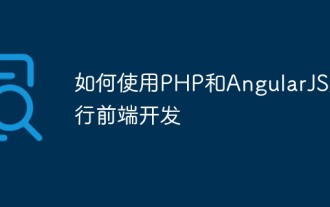
With the popularity and development of the Internet, front-end development has become more and more important. As front-end developers, we need to understand and master various development tools and technologies. Among them, PHP and AngularJS are two very useful and popular tools. In this article, we will explain how to use these two tools for front-end development. 1. Introduction to PHP PHP is a popular open source server-side scripting language. It is suitable for web development and can run on web servers and various operating systems. The advantages of PHP are simplicity, speed and convenience
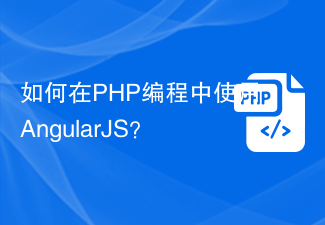
With the popularity of web applications, the front-end framework AngularJS has become increasingly popular. AngularJS is a JavaScript framework developed by Google that helps you build web applications with dynamic web application capabilities. On the other hand, for backend programming, PHP is a very popular programming language. If you are using PHP for server-side programming, then using PHP with AngularJS will bring more dynamic effects to your website.
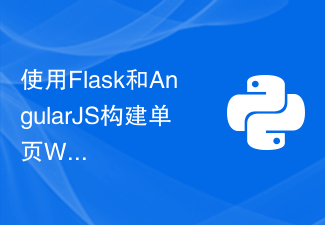
With the rapid development of Web technology, Single Page Web Application (SinglePage Application, SPA) has become an increasingly popular Web application model. Compared with traditional multi-page web applications, the biggest advantage of SPA is that the user experience is smoother, and the computing pressure on the server is also greatly reduced. In this article, we will introduce how to build a simple SPA using Flask and AngularJS. Flask is a lightweight Py
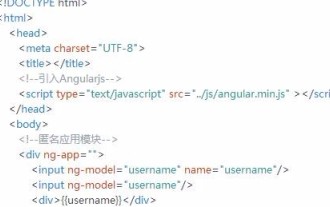
The content of this article is about the basic introduction to AngularJS. It has certain reference value. Now I share it with you. Friends in need can refer to it.
