JavaScript Taobao main image magnifying glass function
If a worker wants to do his job well, he must first sharpen his tools. To achieve a certain effect, we must first understand its principles.
The function of the magnifying glass is to obtain the position of the mouse in the small image, and then calculate the part of the large image that needs to be displayed based on the size ratio of the large and small images, and then use positioning to make the part to be displayed in the large image appear in the right border.
Then look at the code. It will be easier to understand based on the code.
html part
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>放大镜效果</title> <link rel="stylesheet" href="magnifier.css"> </head> <body> <div id="wrapper"> <!--小图--> <div id="img_min"> <!--图片--> <img src="/static/imghw/default1.png" data-src="test.jpg" class="lazy" alt="min"> <!--跟随鼠标的白块--> <p id="mousebg"></p> </div> <!--大图--> <div id="img_max"><img src="/static/imghw/default1.png" data-src="test.jpg" class="lazy" id="img2_img" alt="max"></div> </div> <script type="text/javascript" src="magnifier.js"></script> </body> </html>
css part
*{ margin: 0; padding: 0; } div{ position: relative; } div>div{ width: 300px; height: 300px; float: left; margin: 100px; overflow: hidden; } #img_min>img{ /*display: block;*/ width: 300px; } #img_max{ display: none; } #img_max>img{ position: absolute; top: 0; left: 0; display: block; width: 1500px; } #mousebg{ display: none; position: absolute; width: 60px; height: 60px; background-color: rgba(255,255,255,.7); top: 0; left: 0; }
The most important javascript part
window.onload = function () { var img1 = document.getElementById('img_min');//小图盒子 var img2 = document.getElementById('img_max');//大图盒子 var img2_img = document.getElementById('img2_img');//大图图片 var wrap = document.getElementById('wrapper'); var mousebg = document.getElementById('mousebg');//鼠标白块 var mul = 5; //当某一个模块dispaly:none的时候不能使用offsetWidth获取它的宽高 img1.onmouseover = function () { //鼠标进入 img2.style.display = 'block'; mousebg.style.display = 'block'; } img1.onmouseout = function () { //鼠标离开 img2.style.display = 'none'; mousebg.style.display = 'none'; } img1.onmousemove = function (event) { var _event = event||window.event;//兼容性处理 var mouseX = _event.clientX - wrap.offsetLeft - img1.offsetLeft; //计算鼠标相对与小图的位置 var mouseY = _event.clientY - wrap.offsetTop - img1.offsetTop; //特殊情况处理,分别靠近四条边的时候 if(mouseX<mousebg.offsetWidth/2){ mouseX = mousebg.offsetWidth/2; } if(mouseX>img1.offsetWidth-mousebg.offsetWidth/2){ mouseX = img1.offsetWidth-mousebg.offsetWidth/2; } if(mouseY<mousebg.offsetHeight/2){ mouseY = mousebg.offsetHeight/2; } if(mouseY>img1.offsetHeight-mousebg.offsetHeight/2){ mouseY = img1.offsetHeight-mousebg.offsetHeight/2; } //计算大图的显示范围 img2_img.style.left = -mul*mouseX+img2.offsetWidth/2+"px"; img2_img.style.top = -mul*mouseY+img2.offsetHeight/2+"px"; //使鼠标在白块的中间 mousebg.style.left = mouseX-mousebg.offsetWidth/2+"px"; mousebg.style.top = mouseY-mousebg.offsetHeight/2+"px"; } }
If you understand it after reading the code and comments, use Li Yunlong's words to say: "Oh, you kid tnd is really a genius." Then you can quickly browse through the following analysis part and it will be OK.
Analysis part:
The html and css parts are simple layout codes and will not be explained any more. The js part code is also relatively simple. Let’s directly explain the code of the mouse movement event part.
First, use a picture to explain the principle of obtaining the position of the mouse relative to the small picture:
You can see that through the operation in the code, the value we obtain is the value of the mouse relative to the upper left corner of img1.
After understanding this step, we can actually say that half of our work is done.
Then, we skip the processing of special cases and directly perform the basic operation of positioning the image on the right.
Because the offsetWidth, offsetHeight, style.width, and style.height properties are used. The ranges of style.width, style.height and offsetWidth and offsetHeight are the same. I will describe the other differences in detail in another blog. Let’s first use a picture to understand these attributes, and compare them with the above attributes (pictures are from the Internet, deleted)
Then we explain the code:
Use of pictures in the big picture frame on the right style.left is positioned in the large picture frame. The negative sign is because the movement direction of our mouse is exactly opposite to the movement direction of the picture in our large picture frame. mul is calculated based on the size of the large picture and the small picture. Proportion, what is calculated by -mul*mouseX is actually the relative position of the picture in the large frame, but at this time you will find that the position of your mouse on the right is in the upper left corner of the frame, so we need to add an img2 .offsetWidth/2 to center the image. Similarly, we can perform the same processing on the ordinate.
//计算大图的显示范围 img2_img.style.left = -mul*mouseX+img2.offsetWidth/2+"px"; img2_img.style.top = -mul*mouseY+img2.offsetHeight/2+"px";
Now we are going to deal with special situations. When you do the previous step, you will find that when the mouse moves to the edge, the small white block of the mouse sometimes runs out of the scope of the picture. So we have to process it and limit it to the scope of the picture. Because the mouse is in the middle of the white transparent block, we just limit the mouse to a position that is half the length/width of the white block above, below, left, and right of the picture border. .
//特殊情况处理,分别靠近四条边的时候 if(mouseX<mousebg.offsetWidth/2){ mouseX = mousebg.offsetWidth/2; } if(mouseX>img1.offsetWidth-mousebg.offsetWidth/2){ mouseX = img1.offsetWidth-mousebg.offsetWidth/2; } if(mouseY<mousebg.offsetHeight/2){ mouseY = mousebg.offsetHeight/2; } if(mouseY>img1.offsetHeight-mousebg.offsetHeight/2){ mouseY = img1.offsetHeight-mousebg.offsetHeight/2; }
When the distance to the left is less than half the width, we make mouseX equal to half the width, so that the white block will not continue to move. The same applies to the other three directions.
After completing this step, our effects are complete.
ps: Abstract places can be understood by drawing pictures.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
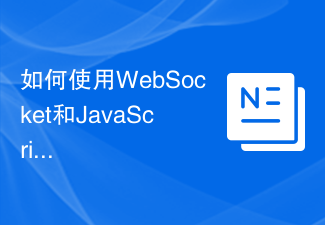
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
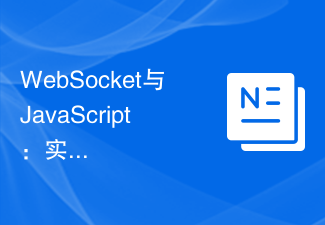
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
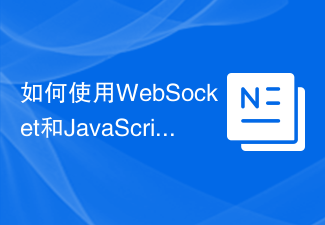
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
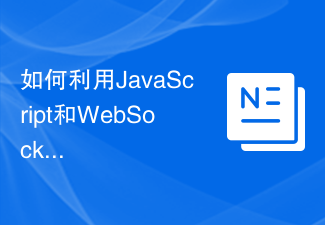
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
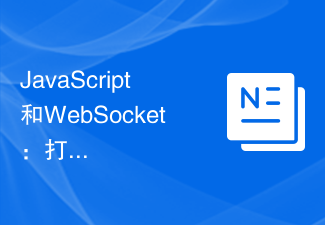
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
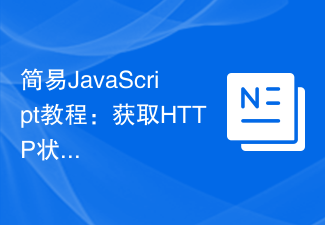
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
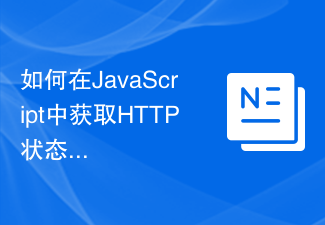
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
