Common classes in Java
API: Application Program Interface, application programming interface. In layman’s terms, it is a set of class libraries provided by the system.
Find the lang package in the API documentation, which contains many commonly used classes.
1.Basic type
Basic data type Class type
byte Byte
short Short
int Integer
long
boolean Boolean
These eight categories Types have their own attributes and methods respectively. Let’s give an example:
Integer class
Attributes:
Maximum value of int: Integer.MAX_VALUE
Minimum value of int: Integer.MIN_VALUE
Method:
Convert an integer to a string: Integer.toString(100);
Convert an integer to a string corresponding to the base system: Integer.toString(a,2);
Convert a purely numeric string to an integer: Integer.valueOf ("12345");
Convert a pure numeric string to an integer: Integer.valueOf("1100011",2);
Convert a pure numeric string to an integer: Integer.parseInt("12345");
Convert a purely numeric string to an integer: Integer.parseInt("1100011",2);
Convert an integer to binary: Integer.toBinaryString(a);
Convert an integer to hexadecimal: Integer.toHexString(a);
Convert an integer to octal: Integer.toOctalString(a);
Example:
1. Convert the positive integer 90 to binary, octal, hexadecimal
java code uPublic class commonly used category { Public Static Void Main (String [] ARGS) { int a = 90; String s = Integer.tobinaryString (a); // A Convert to binary System.out.println(s); println(s1); String s2=Integer.toHexString(a);//Convert a to hexadecimal System.out.println(s2); String s3=Integer.toString(a, 2);//Convert a is output as a string in binary form.Attribute: E
PI
Method: Math .abs(t); //The absolute value of a
Math.max(a,b); //The maximum value of a
Math.min(a,b); //The minimum value of a
Math. pow(a,b); //The b power of a
Math.sqrt(a); //The square root of a
Math.random(); //Randomly obtain a number between 0.0 and 1.0
3. System class
attributes: System.err.println();
System.out.println();
System.in
method: System.exit(0); .
System.currentTimeMillis(); //Get the current system time
System.arraycopy(Object src,int srcPos,Object dest,int destPos, int length); //Copy the array
Parameter 1: Source array
parameter 2: The starting subscript position in the source array
Parameter 3: The target array
Parameter 4: The starting subscript position in the target data
制 Parameter 5: Number of copies Example: int [] a = {1, 2, 3, 4, 5}; int [] b = new int [10]; , 1, b, 4, 3); Java code import java.util.Scanner; public class Common class 1 { public static void main(String[]args ) { Er n Scanner sc = New Scanner (System.in); System.out.println ("Please enter the division and divide number:); int a = sc.nextint (); int b = sc.nextInt(); int[]array1={2,9,11,5,8,3}; int[]array2=new int[6]; if(b==0){ System.err.println ("The dividend cannot be 0");System.out.println(c);
long x=System.currentTimeMillis();
System.out.println("The current time is "+x);
System.arraycopy(array1, 1, array2, 2, 4);
System. out.print("The data in array array2 is ");
;
There are several different sets of standards.
Commonly used character set standards:
ISO-8859 Western European character set, excluding full-width characters
GB2312/GBK Simplified Chinese character set
Big5 Traditional Chinese character set
UTF-8 Character set based on Unicode encoding
ANSI means using the local default character set standard
Construction method
String(byte[] bytes)
String(byte[] bytes, "Character set encoding")
String(byte[] bytes,start( Starting subscript), length (take a few))
String(char[] c)
String(char[] c,start,length)
Method:
Convert string to byte array byte bs[]=s.getBytes();//Get a string array
The string is converted into a byte array according to the specified character set byte bs[]=s.getBytes("UTF-8");
Convert the string into a character array Char c[]=s.toCharArray();
Copy some characters in the string to the character array getChars(int srcBegin, int srcEnd, char[] dst, int dstBegin);
Get the character at the specified position char c=s.charAt(subscript);
Compare two strings in dictionary order compareTo(String anotherString);
Compare two strings in dictionary order A string, regardless of case compareToIgnoreCase(String str);
Determine whether the string contains another string contains(CharSquence(parent class) s);
Determine whether the string is based on a certain ends with a suffix endWith(String s);
Determine whether the string starts with a certain prefix startsWith(String s);
Determine whether the two strings are equal equals(String s);
Determine whether two strings are equal, ignoring case equalsInnoreCase(String s);
Determine the position of the substring in the large string indexOf(s1);
Determine the position of the substring in the large string The last occurrence position in s.lastIndexOf(s1);
Get the character length of the string .length(); The length of the array .length;
will replace the string s.replace("oldChar","newChar");
will replace the first string s.replaceFrist("oldChar","newChar");
intercept characters String s.substring(startIndex(start index));
Convert the replacement string s.substring(startIndex,endIndex(end index));
to lowercase toLowerCase(s1); Uppercase toUpperCase(s1);
Remove the blank characters at the beginning and end of the string trim();
Convert other types of data into string type String.valueOf (any type);
Cut the string String[] s=s1.split();
String class I will give an example, using two methods to display Monday to Friday
Java code
public class lianxi5 {
public static void main(String[] args) {
String z="" ; use ’ s ’ using use using ‐ ‐ ‐ ‐
char c=s.charAt (i); z = z+c;
}
for (int j = 0; j & lt; str.Length (); j ++) {
t = str.charat (j);
System.out.print(z+t+" ");
}
} System.out.println();
System.out.println(); for(int i=0;i t= str.charAt(j); System.out.print(st+t+" "); Wednesday Thursday Friday Saturday Sunday Monday Tuesday Wednesday Thursday Friday Saturday

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
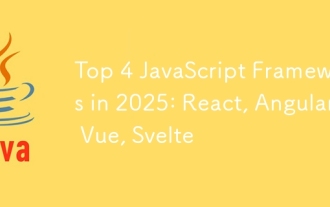
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
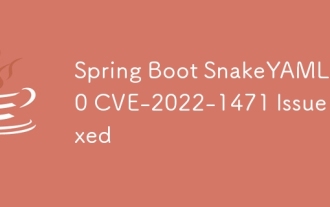
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
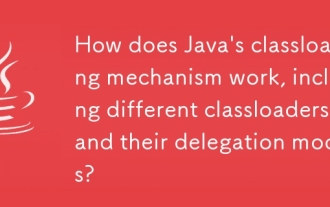
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
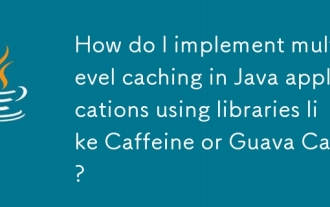
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
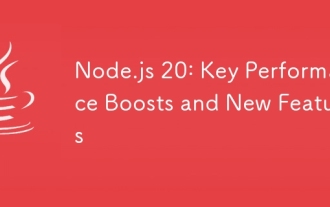
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
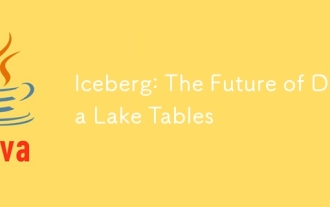
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
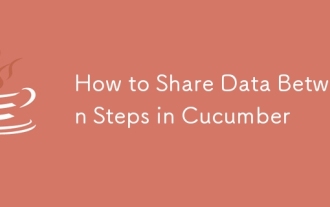
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
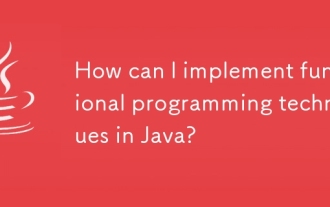
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
