The difference between string pointer and character array
Character arrays and character pointer variables can be used to store and operate strings. But there is a difference between the two. You should pay attention to the following issues when using it:
1. The string pointer variable itself is a variable used to store the first address of the string. The string itself is stored in a continuous memory space headed by the first address and ends with ‘
View Code #include<iostream.h> #include<ctype.h> /******************************************************************************/ /* * Convert a string to lower case */ int strlower(char *string) { if(string==NULL) { return -1; } while(*string) { if(isupper(*string)) *string=tolower(*string); string++; } *string='\0'; return 0; } /*char *strlower(char *string) { char *s; if (string == NULL) { return NULL; } s = string; while (*s) { if (isupper(*s)) { *s = (char) tolower(*s); } s++; } *s = '\0'; return string; } */ void main() { char *test="ABCDEFGhijklmN"; strlower(test); cout<<test<<endl; }
其中,如果采用char *test=”ABCDEFGhijklmN”;会产生运行时错误。Char test[]=”ABCDEFGhijklmN”则程序正常运行,原因如前所述。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


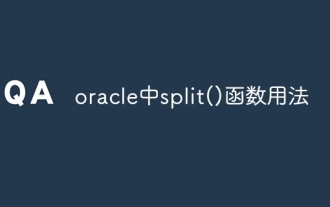
The SPLIT() function splits a string into an array by a specified delimiter, returning a string array where each element is a delimiter-separated portion of the original string. Usage includes: splitting a comma-separated list of values into an array, extracting filenames from paths, and splitting email addresses into usernames and domains.
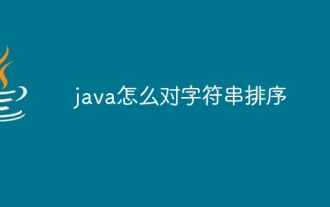
Ways to sort strings in Java: Use the Arrays.sort() method to sort an array of strings in ascending order. Use the Collections.sort() method to sort a list of strings in ascending order. Use the Comparator interface for custom sorting of strings.
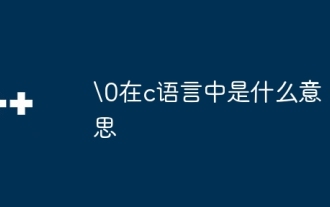
In C language, \0 is the end mark of a string, called the null character or terminator. Since strings are stored in memory as byte arrays, the compiler recognizes the end of the string via \0, ensuring that strings are handled correctly. \0 How it works: The compiler stops reading characters when it encounters \0, and subsequent characters are ignored. \0 itself does not occupy storage space. Benefits include reliable string handling, improved efficiency (no need to scan the entire array to find the end), and ease of comparison and manipulation.
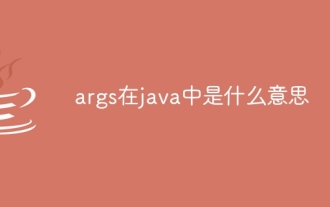
args stands for command line arguments in Java and is an array of strings containing the list of arguments passed to the program when it is started. It is only available in the main method, and its default value is an empty array, with each parameter accessible by index. args is used to receive and process command line arguments to configure or provide input data when a program starts.
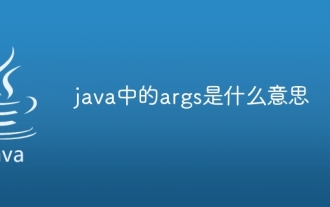
args is a special parameter array of the main method in Java, used to obtain a string array of command line parameters or external input. By accessing the args array, the program can read these arguments and process them as needed.
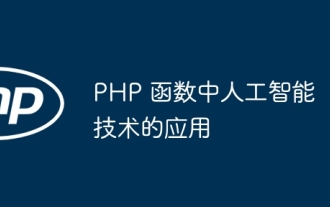
AI technology has been combined with PHP functions to enhance the functionality of the application. Specific AI applications include: using machine learning algorithms to classify text, such as Naive Bayes. Perform in-depth text analysis using natural language processing techniques such as word segmentation and stemming.
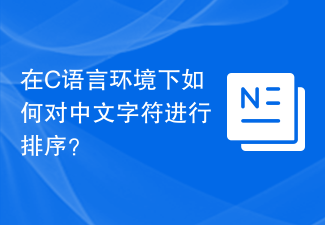
How to implement Chinese character sorting function in C language programming software? In modern society, the Chinese character sorting function is one of the essential functions in many software. Whether in word processing software, search engines or database systems, Chinese characters need to be sorted to better display and process Chinese text data. In C language programming, how to implement the Chinese character sorting function? One method is briefly introduced below. First of all, in order to implement the Chinese character sorting function in C language, we need to use the string comparison function. Ran
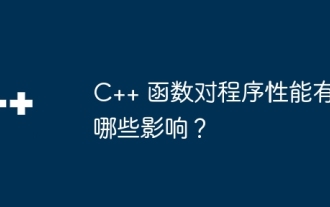
The impact of functions on C++ program performance includes function call overhead, local variable and object allocation overhead: Function call overhead: including stack frame allocation, parameter transfer and control transfer, which has a significant impact on small functions. Local variable and object allocation overhead: A large number of local variable or object creation and destruction can cause stack overflow and performance degradation.
