Vue.js computed properties computed and watch(5)
Binding expressions in templates is very convenient, but they are really only used for simple operations. Templates are used to describe the structure of views. Putting too much logic into a template can make it overweight and difficult to maintain. that's why Vue.js limits binding expressions to one expression. If the logic of more than one expression is required, **computed properties** should be used.
The computed attribute of the Vue instance
<div class="test"> <p>原始的信息{{message}}</p> <p>计算后的信息{{ComputedMessage}}</p> </div>
js code
var myVue = new Vue({ el: ".test", data: { message:12 }, computed:{ ComputedMessage:function () { return this.message+10; } } });
The interface will display 12 and 22
The above method is a buffering implementation effect. This implementation method depends on its buffering, calculated The property will only be revalued when the relevant dependency (message) changes. This means that as long as the message does not change, accessing ComputedMessage multiple times will not re-calculate this property. .
The calculated ComputedMessage property always depends on the message
The same effect is achieved by calling the function
<div class="test"> <p>原始的信息{{message}}</p> <p>计算后的信息{{MessageFunction()}}</p> </div>
js code
var myVue = new Vue({ el: ".test", data: { message:12 }, methods:{ MessageFunction:function () { return this.message+10; } } });
The result obtained is the same as the above result, but it will be re-rendered every time be recalled.
So when using the above two methods, you must first determine whether you need to use caching
Use the watch of the vue instance
I don’t understand this
But using the computed attribute is more convenient and faster
<div class="test"> <p>原始的信息{{fullName}}</p> </div>
js code
var myVue = new Vue({ el: ".test", data: { firstName:"fur", lastName:"bool" }, computed:{ fullName:function () { return this.firstName+this.lastName } } });
And you can set the computed attribute setter and getter which are available by default.
Demonstrates the calling process of set and get
<div class="test"> <p>原始的信息{{fullName}}</p> <button @click="fu">test</button> </div>
js code
var myVue = new Vue({ el: ".test", data: { firstName:"fur", lastName:"bool", fullName:"sasas dsdsd dsds" }, computed:{ fullName:{ get:function () { console.log("get") return this.firstName+this.lastName }, set:function(value){ var names=value.split(" "); this.firstName=names[0]; this.lastName=names[names.length-1]; console.log("set"); } } }, methods:{ fu:function () { myVue.fullName="sasas dsdsd dsds"; console.log(myVue.firstName); //sasas console.log(myVue.lastName); //dsds } } });
will first output get;
When clicking the button to assign a value to fullName, first call set and then call the get method.
Customized Watcher
Although calculated properties are very suitable in most cases, sometimes it is necessary to customize a watcher. This is because you want to perform asynchronous operations and other operations when responding to data changes. It is very useful
The above is the Vue.js calculated properties computed and watch. For more information, please pay attention to the PHP Chinese website (www .php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


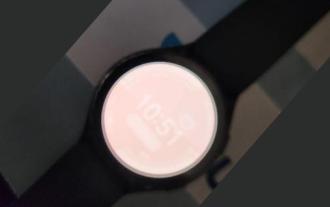
You may have encountered the problem of green lines appearing on the screen of your smartphone. Even if you have never seen it, you must have seen related pictures on the Internet. So, have you ever encountered a situation where the smart watch screen turns white? On April 2, CNMO learned from foreign media that a Reddit user shared a picture on the social platform, showing the screen of the Samsung Watch series smart watches turning white. The user wrote: "I was charging when I left, and when I came back, it was like this. I tried to restart, but the screen was still like this during the restart process." Samsung Watch smart watch screen turned white. The Reddit user did not specify the smart watch. Specific model. However, judging from the picture, it should be Samsung Watch5. Previously, another Reddit user also reported
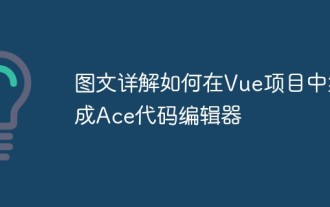
Ace is an embeddable code editor written in JavaScript. It matches the functionality and performance of native editors like Sublime, Vim, and TextMate. It can be easily embedded into any web page and JavaScript application. Ace is maintained as the main editor for the Cloud9 IDE and is the successor to the Mozilla Skywriter (Bespin) project.
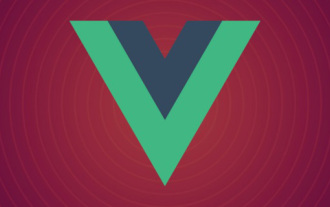
Vue.js has become a very popular framework in front-end development today. As Vue.js continues to evolve, unit testing is becoming more and more important. Today we’ll explore how to write unit tests in Vue.js 3 and provide some best practices and common problems and solutions.
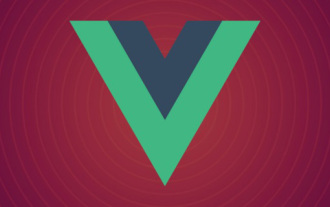
In Vue.js, developers can use two different syntaxes to create user interfaces: JSX syntax and template syntax. Both syntaxes have their own advantages and disadvantages. Let’s discuss their differences, advantages and disadvantages.
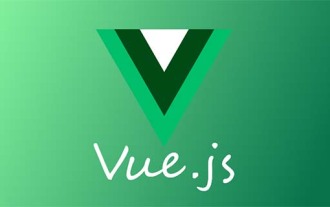
In the actual development project process, sometimes it is necessary to upload relatively large files, and then the upload will be relatively slow, so the background may require the front-end to upload file slices. It is very simple. For example, 1 A gigabyte file stream is cut into several small file streams, and then the interface is requested to deliver the small file streams respectively.
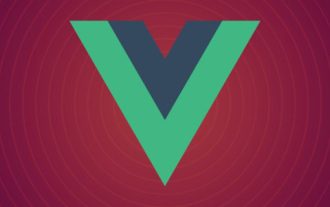
When we used Amap, the official recommended many cases and demos to us, but these cases all used native methods to access and did not provide demos of vue or react. Many people have written about vue2 access on the Internet. However, in this article, we will take a look at how vue3 uses the commonly used Amap API. I hope it will be helpful to everyone!
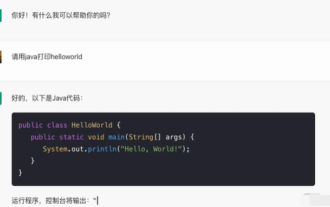
When I was working on the chatgpt mirror site, I found that some mirror sites did not have typewriter cursor effects, but only text output. Did they not want to do it? I want to do it anyway. So I studied it carefully and realized the effect of typewriter plus cursor. Now I will share my solution and renderings~
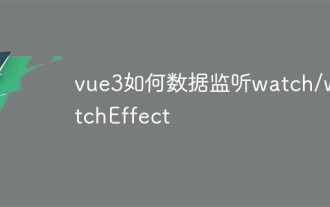
We all know that the function of the listener is to trigger every time the reactive state changes. In the combined API, we can use the watch() function and watchEffect() function. When you change the reactive state, it may be triggered at the same time. Trigger Vue component updates and listener callbacks. By default, user-created listener callbacks will be called before the Vue component is updated. This means that the DOM you access in the listener callback will be the state it was in before it was updated by Vue. So, let’s take a look, how can we make good use of them? What's the difference between them? The watch() function watch needs to listen to a specific data source, such as listening to a ref. The first parameter of watch can be
