


Detailed explanation of the differences between ==, Equals, and ReferenceEquals in C#
Introduction to this article:
Equals, ==, in C# ReferenceEquals can be used to determine whether the individuals of two objects are equal. For the same basic value type, the comparison results of == and Equals() are the same; since ReferenceEquals() determines whether the references of two objects are equal, for values Type, because a boxing operation must be performed before each judgment, that is, a temporary object is generated every time, so false will always be returned.
1. == operator
1. Static equality symbol, corresponding to the existing !=. This symbol is an overloadable binary operator that can be used to compare whether two objects are equal.
2. It will automatically perform necessary type conversion as needed, and return true or false depending on whether the values of the two objects are equal.
3. For reference objects, compare their references (except for string reference types, string is a comparison value)
4. For value types, compare their values
5. Some built-in reference types overload the == symbol, such as string Just overload == so that it compares not the references of the two strings, but whether the two string literals are equal.
6. For example:
int i = 5; int j = 5; Console.WriteLine(i == j);//值类型比较代数值 输出True int m = 6; double n = 6.0; Console.WriteLine(m == n);//类型自动转换并比较数值 输出True object obj1 = new object(); object obj2 = new object(); Console.WriteLine(obj2==obj1);//引用类型比较引用 输出False
2. Equals
1. Used to compare whether the references of two objects are equal.
2. However, for value types, if the type is the same (no automatic type conversion will be performed), and the value is the same (each member of the struct must be the same), then Equals returns
true, otherwise return false.
3. For reference types, the default behavior is the same as that of ReferenceEquals. True is returned only when two objects point to the same Reference.
4. Equals can be overloaded as needed
5. Instance
int i = 5; int j = 5; Console.WriteLine(i.Equals(j));//值类型比较 输出True int m = 6; double n = 6.0; Console.WriteLine(m.Equals(n));//类型不会自动转换并比较数值 输出False object obj1 = new object(); object obj2 = new object(); Console.WriteLine(obj2.Equals(obj1));//引用类型比较 输出False Console.WriteLine(obj2.Equals(string.Empty));//输出False,比较量对象的类型不同直接返回False
3. ReferenceEquals
1. Static method of Object, compares whether the references of two objects are equal, value type and reference type It's all the same.
2. This method cannot be overridden in inherited classes. The prototype is: public static bool ReferenceEquals(object objA, object
objB);FCL has been implemented for us. It is to compare whether the memory address pointed to by the reference is the same.
3. For two value types, ReferenceEquals is always false because ReferenceEquals(object a,object
b) After the method, the value type is reboxed into a new reference type instance, and naturally there is no reference equality.
4. For two reference types, ReferenceEquals will compare whether they point to the same address.
5. Examples
int i = 5; int j = 5; Console.WriteLine(object.ReferenceEquals(i, j));//输出False int m = 6; double n = 6.0; Console.WriteLine(object.ReferenceEquals(m, n));//输出False object obj1 = new object(); object obj2 = new object(); Console.WriteLine(object.ReferenceEquals(obj1, obj2));//输出False
The above are the differences between ==, Equals, and ReferenceEquals. I hope it will be helpful to everyone's learning. For more related articles, please pay attention to the PHP Chinese website (www.php.cn) !

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


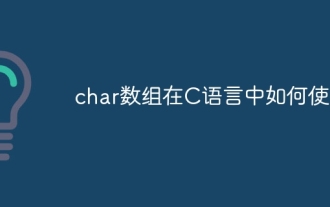
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
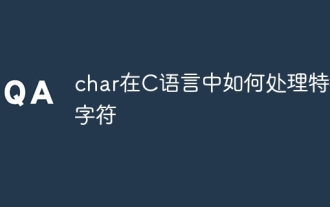
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
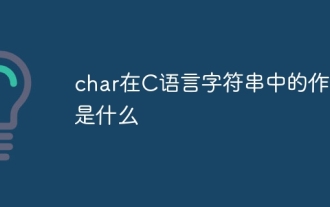
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
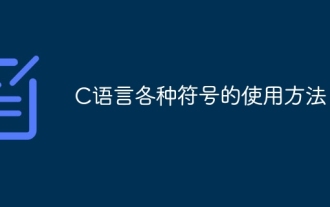
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
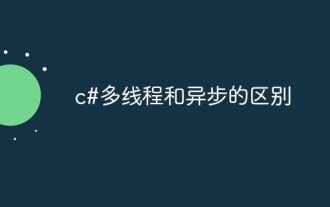
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
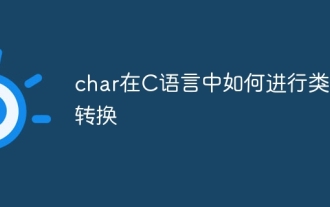
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
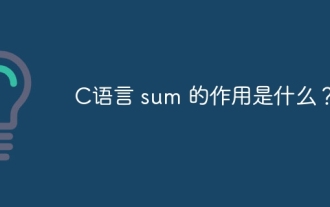
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
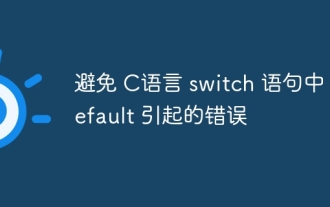
A strategy to avoid errors caused by default in C switch statements: use enums instead of constants, limiting the value of the case statement to a valid member of the enum. Use fallthrough in the last case statement to let the program continue to execute the following code. For switch statements without fallthrough, always add a default statement for error handling or provide default behavior.
