Usage of inner classes and anonymous inner classes
1. Inner classes:
(1) Methods with the same name of the inner class
The inner class can call methods of the outer class. If the inner class has a method with the same name, it must be called using the "OuterClass.this.MethodName()" format (where OuterClass Replace MethodName with the actual external class name and its method; this is the keyword, indicating a reference to the external class); if the internal class does not have a method with the same name, you can directly call the method of the external class.
But peripheral classes cannot directly call private methods of inner classes, and external classes cannot directly call private methods of other classes. Note: The permission of the inner class to directly use the outer class has nothing to do with whether the method is static. It depends on whether the inner class has a method with the same name.
package innerclass; public class OuterClass { private void outerMethod() { System.out.println("It's Method of OuterClass"); } public static void main(String[] args) { OuterClass t = new OuterClass(); OuterClass.Innerclass in = t.new Innerclass(); in.innerMethod(); } class Innerclass { public void innerMethod() { OuterClass.this.outerMethod();// 内部类成员方法与外部类成员方法同名时,使用this调用外部类的方法 outerMethod();// 内部类没有同名方法时执行外部类的方法 } private void outerMethod() { System.out.println("It's Method of Innerclass"); } } }
The output result is:
It's Method of OuterClass It's Method of Innerclass
(2) Instantiation of inner classes
Instantization of inner classes is different from ordinary classes. Ordinary classes can be instantiated whenever needed, while inner classes must be instantiated after the outer class is instantiated Only then can it be instantiated and establish a relationship with the external class
So in the non-static method in the external class, the inner class object can be instantiated
private void outerMethod() { System.out.println("It's Method of OuterClass"); Innerclass in = new Innerclass();//在外部类的outerMethod方法中实例化内部类是可以啊 }
But in the static method, you need to pay attention! ! ! ! You cannot directly new the inner class in the static method, otherwise an error will occur:
No enclosing instance of type OuterClass is accessible. Must qualify the allocation with an enclosing instance of type OuterClass (e.g. x.new A() where x is an instance of OuterClass).
This is because the static method can be used before the class is instantiated. It is called through the class name. At this time, the dynamic inner class has not been instantiated yet. How to use it? You cannot call something that does not exist. .
If you want to create a new inner class in the Static method, you can declare the inner class as Static
public class OuterClass { private void outerMethod() { System.out.println("It's Method of OuterClass"); } public static void main(String[] args) { Innerclass in = new Innerclass(); in.innerMethod(); } static class Innerclass {//把内部类声明为static public void innerMethod() { System.out.println("It's Method of innerMethod"); } } }
Of course, the static method is generally not used, but this method is recommended: x.new A(), where x is the external class An instance of OuterClass, A is the inner class Innerclass
package innerclass; public class OuterClass { private void outerMethod() { System.out.println("It's Method of OuterClass"); } public static void main(String[] args) { OuterClass.Innerclass in = new OuterClass().new Innerclass();//使用x.new A()的方式 in.innerMethod(); } class Innerclass { public void innerMethod() { System.out.println("It's Method of innerMethod"); } } }
x.new A(), where x is an instance of the outer class OuterClass, A is the class class Innerclass, of course it can be split as follows, so it is obviously clear:
(3) When to use inner classes? The typical situation is that the inner class inherits from a certain class or implements a certain interface, and the code of the inner class operates the object of the outer class that created it. So you can think of inner classes as providing some kind of window into their outer classes.The most attractive reason for using inner classes is that each inner class can independently inherit from an implementation (of the interface), so no matter whether the outer class has inherited an implementation of an (interface), for the inner class All have no effect. Without the ability provided by inner classes to inherit from multiple concrete or abstract classes, some design and programming problems would be difficult to solve. From this perspective, inner classes make the multiple inheritance solution complete. Interfaces solve part of the problem, while inner classes effectively implement "multiple inheritance."
(4) Example of instantiating an inner class in a static method: (The inner class is placed in a static method)
public static void main(String[] args) { OuterClass out = new OuterClass();//外部实例 OuterClass.Innerclass in = out.new Innerclass();//外部实例.new 外部类 in.innerMethod(); }
per.speak() can be called, but per.say() cannot be called, because per is Person Object, if you want to call the method of a subclass, you can force downcasting to: ((Boy) per).say(); or directly change to Boy per = new Boy();. It can be found that if you want to call a custom method of an inner class, you must call it through the object of the inner class. So, if the anonymous inner class doesn't even have a name, how can it call the custom method of the inner class?
(2) Anonymous inner classes Anonymous inner classes are also inner classes without names. Because they have no names, anonymous inner classes can only be used once. They are usually used to simplify code writing, but using anonymous inner classes is also There is a prerequisite: you must inherit a parent class or implement an interface, but you can only inherit at most one parent class or implement an interface.
There are two more rules about anonymous inner classes:
1) Anonymous inner classes cannot be abstract classes, because when the system creates an anonymous inner class, it will immediately create an object of the inner class. Therefore, anonymous inner classes are not allowed to be defined as abstract classes.2) Anonymous inner classes do not define constructors (constructors). Because anonymous inner classes have no class name, they cannot define a constructor, but anonymous inner classes can define instance initialization blocks.
How to determine the existence of an anonymous class? ? I can't see the name, it feels like it's just an object created by new from the parent class, and there is no name for the anonymous class.
Let’s look at the pseudocode first
package javatest2; public class JavaTest2 { public static void main(String[] args) { class Boy implements Person { public void say() {// 匿名内部类自定义的方法say System.out.println("say方法调用"); } @Override public void speak() {// 实现接口的的方法speak System.out.println("speak方法调用"); } } Person per = new Boy(); per.speak();// 可调用 per.say();// 不能调用 } } interface Person { public void speak(); }
Generally speaking, when new an object, there should be a semicolon after the parentheses, that is, the statement of new object ends. But it's different when there are anonymous inner classes. The parentheses are followed by braces, and the braces contain the specific implementation method of the new object. Because we know that an abstract class cannot be new directly. There must be an implementation class before we can new its implementation class. The above pseudocode indicates that new is the implementation class of Father, and this implementation class is an anonymous inner class. In fact, splitting the anonymous inner class above can be:
abstract class Father(){ .... } public class Test{ Father f1 = new Father(){ .... } //这里就是有个匿名内部类 }
Let’s look at an example first to experience the usage of anonymous inner classes:
运行结果:eat something
可以看到,我们直接将抽象类Person中的方法在大括号中实现了,这样便可以省略一个类的书写。并且,匿名内部类还能用于接口上
public class JavaTest2 { public static void main(String[] args) { Person per = new Person() { public void say() {// 匿名内部类自定义的方法say System.out.println("say方法调用"); } @Override public void speak() {// 实现接口的的方法speak System.out.println("speak方法调用"); } }; per.speak();// 可调用 per.say();// 出错,不能调用 } } interface Person { public void speak(); }
这里per.speak()是可以正常调用的,但per.say()不能调用,为什么呢?注意Person per = new Person()创建的是Person的对象,而非匿名内部类的对象。其实匿名内部类连名字都没有,你咋实例对象去调用它的方法呢?但继承父类的方法和实现的方法是可以正常调用的,本例子中,匿名内部类实现了接口Person的speak方法,因此可以借助Person的对象去调用。
若你确实想调用匿名内部类的自定义的方法say(),当然也有方法:
(1)类似于speak方法的使用,先在Person接口中声明say()方法,再在匿名内部类中覆写此方法。
(2)其实匿名内部类中隐含一个匿名对象,通过该方法可以直接调用say()和speak()方法;代码修改如下:
public class JavaTest2 { public static void main(String[] args) { new Person() { public void say() {// 匿名内部类自定义的方法say System.out.println("say方法调用"); } @Override public void speak() {// 实现接口的的方法speak System.out.println("speak方法调用"); } }.say();// 直接调用匿名内部类的方法 } } interface Person { public void speak(); }
更多内部类和匿名内部类的用法相关文章请关注PHP中文网!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


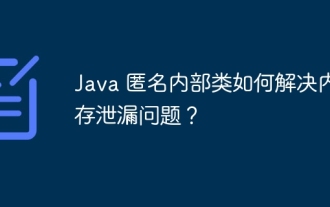
Anonymous inner classes can cause memory leaks. The problem is that they hold a reference to the outer class, preventing the outer class from being garbage collected. Solutions include: 1. Use weak references. When the external class is no longer held by a strong reference, the garbage collector will immediately recycle the weak reference object; 2. Use soft references. The garbage collector will recycle the weak reference object when it needs memory during garbage collection. Only then the soft reference object is recycled. In actual combat, such as in Android applications, the memory leak problem caused by anonymous inner classes can be solved by using weak references, so that the anonymous inner class can be recycled when the listener is not needed.
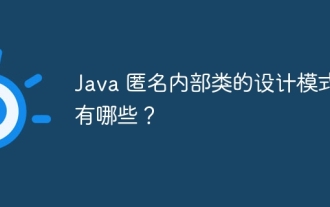
Anonymous inner classes are special inner classes in Java that have no explicit name and are created through the new expression. They are mainly used to implement specific interfaces or extend abstract classes and are used immediately after creation. Common anonymous inner class design patterns include: Adapter pattern: converts one interface into another interface. Strategy Pattern: Defining and Replacement Algorithms. Observer pattern: Register observers and handle events. It is very useful in practical applications, such as sorting a TreeSet by string length, creating anonymous threads, etc.
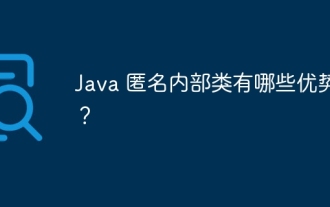
Anonymous inner classes are used in Java as special inner classes that facilitate subclassing, simplifying code, and handling events (such as button clicks). Practical cases include: Event handling: Use anonymous inner classes to add click event listeners for buttons. Data transformation: Sort collections using Collections.sort method and anonymous inner class as comparator.
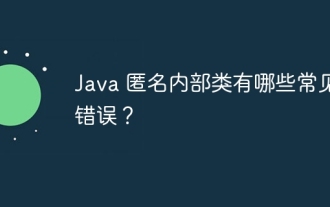
Anonymous inner class usage error: Accessing an out-of-scope variable using catching an undeclared exception in a non-thread-safe environment
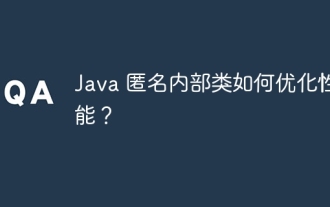
The performance problem of anonymous inner classes is that they are recreated every time they are used, which can be optimized through the following strategies: 1. Store anonymous inner classes in local variables; 2. Use non-static inner classes; 3. Use lambda expressions. Practical tests show that lambda expression optimization has the best effect.
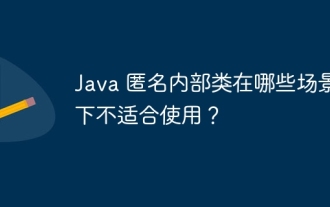
Anonymous inner classes are not suitable for use when: need to access private members, need multiple instances, need inheritance, need to access generic types
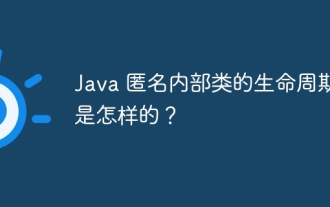
The lifetime of an anonymous inner class is determined by its scope: Method-local inner class: Valid only within the scope of the method that created it. Constructor inner class: bound to the outer class instance and released when the outer class instance is released. Static inner classes: loaded and unloaded at the same time as external classes.
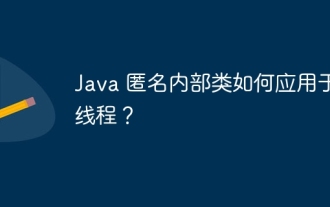
Anonymous inner classes simplify the creation of multi-threaded code, eliminating the need for naming and enabling instant definition and use of thread classes. The main advantage is to simplify the code, while the limitation is that it cannot be extended. Use when you need to quickly create one or two threads. Keep the code short. If more complex logic is required, a separate class file should be created.
