Java: Define and start threads inside methods using anonymous inner classes
The following code shows how to define a Thread through an anonymous inner class in a method, Override its run() method, and then start the thread directly.
Such code can be used to perform a side task by starting a new thread within a class. Generally, such tasks are not the main design content of the class.
package com.zj.concurrency; public class StartFromMethod { private Thread t; private int number; private int count = 1; public StartFromMethod(int number) { this.number = number; } public void runTask() { if (t == null) { t = new Thread() { public void run() { while (true) { System.out.println("Thread-" + number + " run " + count + " time(s)"); if (++count == 3) return; } } }; t.start(); } } public static void main(String[] args) { for (int i = 0; i < 5; i++) new StartFromMethod(i).runTask(); } }
Result:
Thread-0 run 1 time(s)
Thread-0 run 2 time(s)
Thread-1 run 1 time(s)
Thread-1 run 2 time(s)
Thread-2 run 1 time(s)
Thread-2 run 2 time(s)
Thread-3 run 1 time(s)
Thread-3 run 2 time(s)
Thread-4 run 1 time(s)
Thread-4 run 2 time(s)
More Java: Use anonymous inner classes to define and start threads inside methods. For related articles, please pay attention to PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


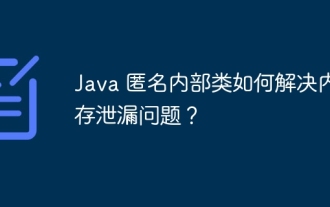
Anonymous inner classes can cause memory leaks. The problem is that they hold a reference to the outer class, preventing the outer class from being garbage collected. Solutions include: 1. Use weak references. When the external class is no longer held by a strong reference, the garbage collector will immediately recycle the weak reference object; 2. Use soft references. The garbage collector will recycle the weak reference object when it needs memory during garbage collection. Only then the soft reference object is recycled. In actual combat, such as in Android applications, the memory leak problem caused by anonymous inner classes can be solved by using weak references, so that the anonymous inner class can be recycled when the listener is not needed.
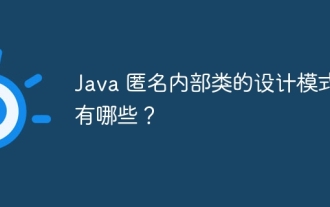
Anonymous inner classes are special inner classes in Java that have no explicit name and are created through the new expression. They are mainly used to implement specific interfaces or extend abstract classes and are used immediately after creation. Common anonymous inner class design patterns include: Adapter pattern: converts one interface into another interface. Strategy Pattern: Defining and Replacement Algorithms. Observer pattern: Register observers and handle events. It is very useful in practical applications, such as sorting a TreeSet by string length, creating anonymous threads, etc.
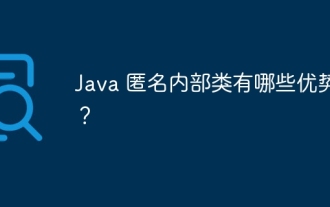
Anonymous inner classes are used in Java as special inner classes that facilitate subclassing, simplifying code, and handling events (such as button clicks). Practical cases include: Event handling: Use anonymous inner classes to add click event listeners for buttons. Data transformation: Sort collections using Collections.sort method and anonymous inner class as comparator.
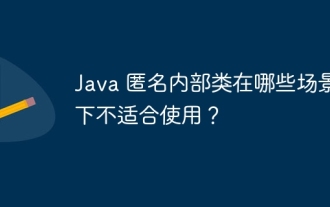
Anonymous inner classes are not suitable for use when: need to access private members, need multiple instances, need inheritance, need to access generic types
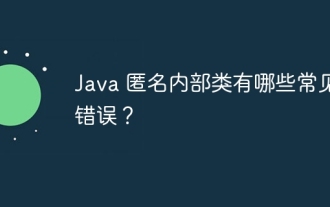
Anonymous inner class usage error: Accessing an out-of-scope variable using catching an undeclared exception in a non-thread-safe environment
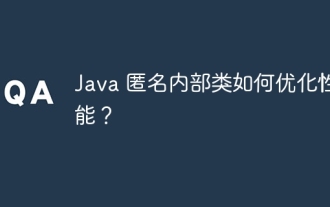
The performance problem of anonymous inner classes is that they are recreated every time they are used, which can be optimized through the following strategies: 1. Store anonymous inner classes in local variables; 2. Use non-static inner classes; 3. Use lambda expressions. Practical tests show that lambda expression optimization has the best effect.
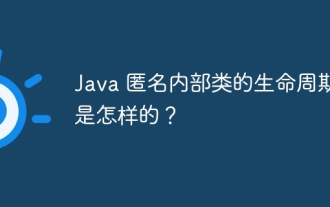
The lifetime of an anonymous inner class is determined by its scope: Method-local inner class: Valid only within the scope of the method that created it. Constructor inner class: bound to the outer class instance and released when the outer class instance is released. Static inner classes: loaded and unloaded at the same time as external classes.
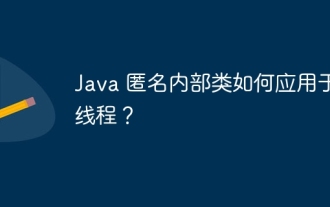
Anonymous inner classes simplify the creation of multi-threaded code, eliminating the need for naming and enabling instant definition and use of thread classes. The main advantage is to simplify the code, while the limitation is that it cannot be extended. Use when you need to quickly create one or two threads. Keep the code short. If more complex logic is required, a separate class file should be created.
