PHP technology advancement PHP SOCKET technology research
Today I tried to write a program that communicates between PHP and C language through socket. After reading the PHP manual, I found that there are several ways to establish a socket client.
1. Establish a socket connection through fsockopen(), and then use fputs( ) to send messages and use fgets() to receive messages.
2. Establish a socket connection through socket_create(), then use socket_send() or socket_write() to send messages, and use socket_recv() or socket_read() to send messages.
It’s strange, I saw this paragraph in the manual: "This extension module is experimental. The behavior of this module, including the names of its functions and any other documentation about this module may change without notice. PHP will change in future releases. We remind you to use this extension module at your own risk. "It seems that php4.0 socket communication is not yet completely stable.
The client I wrote today needs to communicate with the server twice. I used the above method to write a client program. I found that when there was only one communication, that is, the PHP client sent a message once and then received it. Return the message and close the connection. Both methods can implement functions correctly and quickly, but there are obvious differences when communicating twice. The first communication with the first method ends very quickly. I can see this through the output of the server, but It took several minutes for the second communication to end. I tried it several times and it still happened. I’m not sure what went wrong with my program, or there was something wrong with the connection this way. However, the second method failed to complete these two communications. Quick and correct! Very well done.
Finally, I wrote a class based on the second situation
////////////////////////////// File Description ////////////////////////////////////////// // Class Name : socket // Version : V1.0 // Functional Outline : create socket,and send message to server // Revision history : 2004/12/15 First version created // Current : 2004/12/15 Liu Yongsheng ////////////////////////////////////////////////////////////////////////////////////////// class socket{ var $socket; //socket 句柄 var $sendflag = ">>>"; var $recvflag = "<<<"; var $response; var $debug = 1; function socket($hostname,$port){ $address = gethostbyname($hostname); $this->socket = socket_create(AF_INET,SOCK_STREAM,SOL_TCP); $result = socket_connect($this->socket,$address,$port); if($this->debug == 1){ if ($result < 0) { echo "socket_connect() failed.\nReason: ($result) " . socket_strerror($result) . "<br>"; } else{ echo "connect OK.<br>"; } } } function sendmsg($msg){ socket_write($this->socket,$msg,strlen($msg)); $result = socket_read($this->socket,100); $this->response = $result; if($this->debug == 1){ printf("<font color=#CCCCCC>%s $msg</fon><br>",$this->sendflag); printf("<font color=blue>%s $result</font><br>",$this->recvflag); } return $result; } function close(){ socket_close($this->socket); } }
The above is the content of PHP technology advanced PHP SOCKET technology research. For more related articles, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
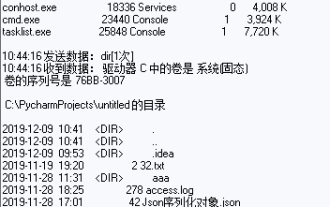
1. Socket programming based on TCP protocol 1. The socket workflow starts with the server side. The server first initializes the Socket, then binds to the port, listens to the port, calls accept to block, and waits for the client to connect. At this time, if a client initializes a Socket and then connects to the server (connect), if the connection is successful, the connection between the client and the server is established. The client sends a data request, the server receives the request and processes the request, then sends the response data to the client, the client reads the data, and finally closes the connection. An interaction ends. Use the following Python code to implement it: importso
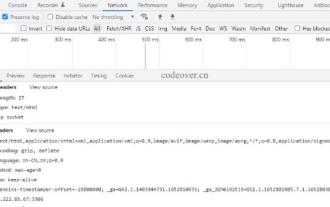
This article brings you relevant knowledge about php+socket, which mainly introduces IO multiplexing and how php+socket implements web server? Friends who are interested can take a look below. I hope it will be helpful to everyone.
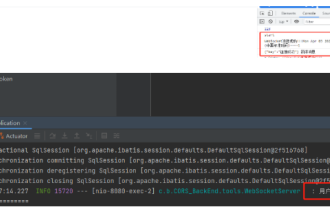
The first step on the SpringBoot side is to introduce dependencies. First we need to introduce the dependencies required for WebSocket, as well as the dependencies for processing the output format com.alibabafastjson1.2.73org.springframework.bootspring-boot-starter-websocket. The second step is to create the WebSocket configuration class importorg. springframework.context.annotation.Bean;importorg.springframework.context.annotation.Config
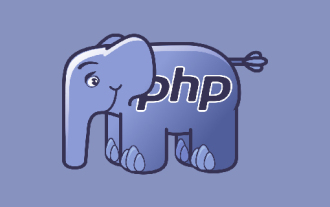
Solution to the problem that the php socket cannot be connected: 1. Check whether the socket extension is enabled in php; 2. Open the php.ini file and check whether "php_sockets.dll" is loaded; 3. Uncomment "php_sockets.dll".
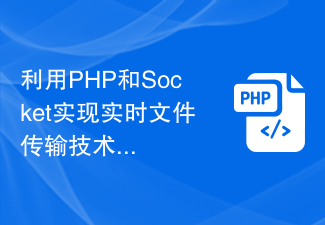
With the development of the Internet, file transfer has become an indispensable part of people's daily work and entertainment. However, traditional file transfer methods such as email attachments or file sharing websites have certain limitations and cannot meet the needs of real-time and security. Therefore, using PHP and Socket technology to achieve real-time file transfer has become a new solution. This article will introduce the technical principles, advantages and application scenarios of using PHP and Socket technology to achieve real-time file transfer, and demonstrate the implementation method of this technology through specific cases. technology
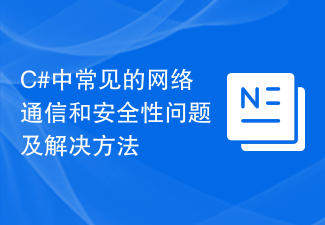
Common network communication and security problems and solutions in C# In today's Internet era, network communication has become an indispensable part of software development. In C#, we usually encounter some network communication problems, such as data transmission security, network connection stability, etc. This article will discuss in detail common network communication and security issues in C# and provide corresponding solutions and code examples. 1. Network communication problems Network connection interruption: During the network communication process, the network connection may be interrupted, which may cause
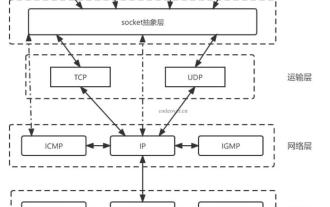
This article brings you relevant knowledge about php+socket. It mainly introduces what is socket? How does php+socket realize client-server data transmission? Friends who are interested can take a look below. I hope it will be helpful to everyone.
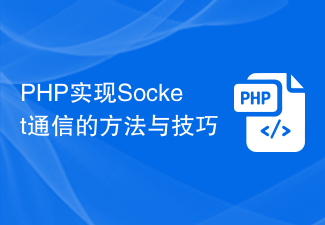
PHP is a commonly used development language that can be used to develop various web applications. In addition to common HTTP requests and responses, PHP also supports network communication through Sockets to achieve more flexible and efficient data interaction. This article will introduce the methods and techniques of how to implement Socket communication in PHP, and attach specific code examples. What is Socket Communication Socket is a method of communication in a network that can transfer data between different computers. by S
